Checked and Unchecked Exceptions in Java
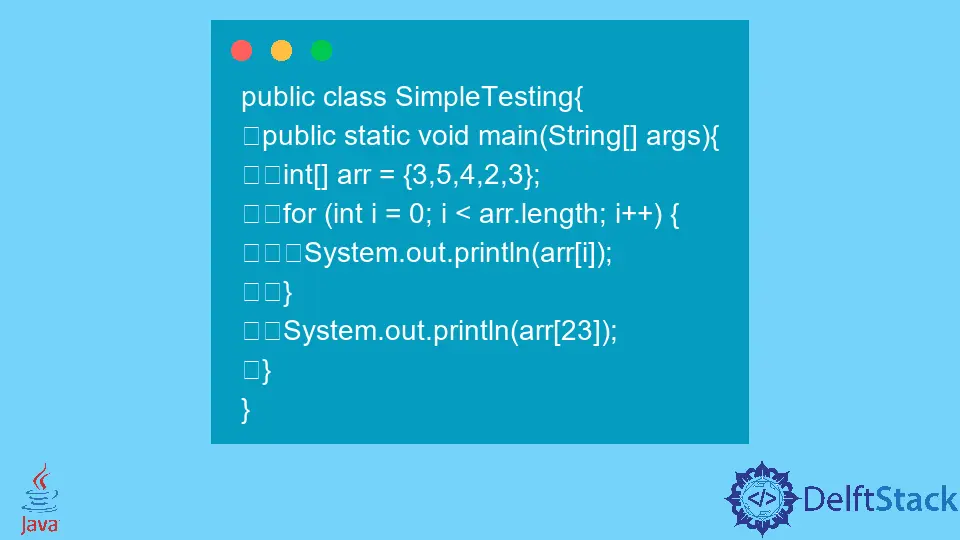
This tutorial introduces what checked and unchecked exceptions in Java are.
In Java, an exception is a situation that occurs during code execution and terminates the execution abnormally. This exception can occur at either compile-time or runtime. Java broadly categorizes the exception in two types, checked and unchecked. Exception, which is checked by the compiler at compile-time, is known as a checked exception. Meanwhile, an exception that is checked at runtime is called an unchecked exception.
To deal with exceptions, Java provides classes for each exception such as NullPointerException
, ArrayIndexOutofBoundsException
, IOException
, etc. The Exception
class is on the top of all the exception classes and anything that is a subclass of Exception
except for RuntimeException
and its subclasses is a checked exception.
Exception classes that inherit the RuntimeException
such as ArithmeticException
, NullPointerException
, and ArrayIndexOutOfBoundsException
are called unchecked exceptions. These are checked at runtime.
Checked Exceptions in Java
Let’s first understand what an exception is and how it affects the code execution. In this example, we are writing data to a file, and this code is prone to exceptions such as FileNotFoundException
, IOException
, etc. We did not provide any catch handler, and since these are checked exceptions, the Java compiler does not compile the code and throws the exceptions at compile time. See the example below.
import java.io.FileOutputStream;
public class SimpleTesting {
public static void main(String[] args) {
FileOutputStream fout =
new FileOutputStream("/home/root/eclipse-workspace/java-project/myfile.txt");
fout.write(1256);
fout.close();
System.out.println("Data written successfully");
}
}
Output:
Exception in thread "main" java.lang.Error: Unresolved compilation problems:
Unhandled exception type FileNotFoundException
Unhandled exception type IOException
Unhandled exception type IOException
at SimpleTesting.main(SimpleTesting.java:8)
To avoid any abnormal termination of the code, we must provide a catch handler to the code. Below is the same code as above, but it has a catch handler so that code does not terminate in between and execute fine. See the example below.
import java.io.FileOutputStream;
public class SimpleTesting {
public static void main(String[] args) {
try {
FileOutputStream fout =
new FileOutputStream("/home/irfan/eclipse-workspace/ddddd/myfile.txt");
fout.write(1256);
fout.close();
System.out.println("Data written successfully");
} catch (Exception e) {
System.out.println(e);
}
}
}
Output:
Data written successfully
Unchecked Exception in Java
The below code throws ArrayIndexOutOfBoundsException
, which is an unchecked exception. This code compiles successfully but does not run as we are accessing elements out of the range of the array; hence code throws a runtime exception. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = {3, 5, 4, 2, 3};
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
System.out.println(arr[23]);
}
}
Output:
3
5
4
2
3
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 23 out of bounds for length 5
at SimpleTesting.main(SimpleTesting.java:9)