Java 中的已检查和未检查的异常
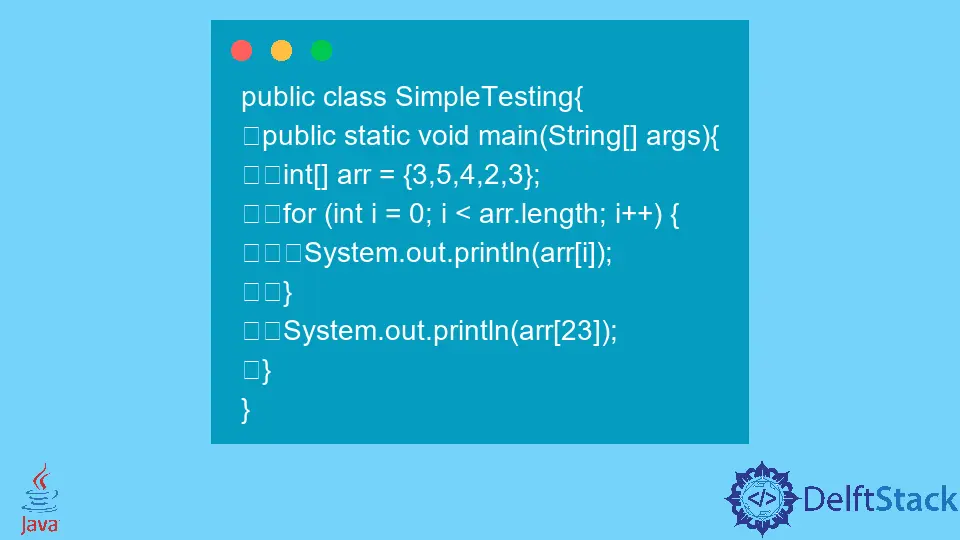
本教程介绍了 Java 中已检查和未检查的异常是什么。
在 Java 中,异常是在代码执行过程中发生并异常终止执行的情况。此异常可能发生在编译时或运行时。Java 将异常大致分为两种类型,检查的和未检查的。由编译器在编译时检查的异常称为已检查异常。同时,在运行时检查的异常称为未检查异常。
为了处理异常,Java 为每个异常提供了类,例如 NullPointerException
、ArrayIndexOutofBoundsException
、IOException
等。Exception
类位于所有异常类和任何属于 Exception 子类的顶部
除了 RuntimeException
及其子类是受检异常。
继承 RuntimeException
的异常类,例如 ArithmeticException
、NullPointerException
和 ArrayIndexOutOfBoundsException
称为未经检查的异常。这些是在运行时检查的。
Java 中被检查的异常
让我们首先了解什么是异常以及它如何影响代码执行。在这个例子中,我们将数据写入文件,这段代码容易出现诸如 FileNotFoundException
、IOException
等异常。我们没有提供任何 catch 处理程序,由于这些是检查异常,Java 编译器会不编译代码并在编译时抛出异常。请参阅下面的示例。
import java.io.FileOutputStream;
public class SimpleTesting {
public static void main(String[] args) {
FileOutputStream fout =
new FileOutputStream("/home/root/eclipse-workspace/java-project/myfile.txt");
fout.write(1256);
fout.close();
System.out.println("Data written successfully");
}
}
输出:
Exception in thread "main" java.lang.Error: Unresolved compilation problems:
Unhandled exception type FileNotFoundException
Unhandled exception type IOException
Unhandled exception type IOException
at SimpleTesting.main(SimpleTesting.java:8)
为了避免代码的任何异常终止,我们必须为代码提供一个 catch
处理程序。下面是与上面相同的代码,但它有一个 catch 处理程序,因此代码不会在两者之间终止并正常执行。请参阅下面的示例。
import java.io.FileOutputStream;
public class SimpleTesting {
public static void main(String[] args) {
try {
FileOutputStream fout =
new FileOutputStream("/home/irfan/eclipse-workspace/ddddd/myfile.txt");
fout.write(1256);
fout.close();
System.out.println("Data written successfully");
} catch (Exception e) {
System.out.println(e);
}
}
}
输出:
Data written successfully
Java 中的未检查异常
下面的代码抛出 ArrayIndexOutOfBoundsException
,这是一个未经检查的异常。这段代码编译成功但没有运行,因为我们正在访问数组范围之外的元素;因此代码抛出运行时异常。请参阅下面的示例。
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = {3, 5, 4, 2, 3};
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
System.out.println(arr[23]);
}
}
输出:
3
5
4
2
3
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 23 out of bounds for length 5
at SimpleTesting.main(SimpleTesting.java:9)