How to Get Char Array's Length in Java
-
Get the Length of a Char Array in Java Using the
length
Property - Get the Length of a Char Array Using a Loop in Java
- Get the Length of a Char Array Using the String Class in Java
- Conclusion
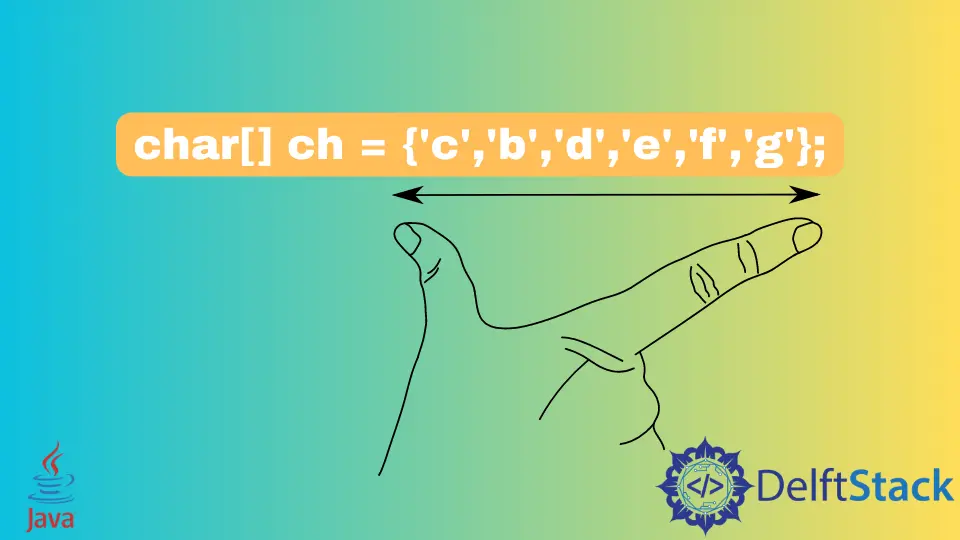
When working with char arrays in Java, it’s essential to determine their length to effectively manage and manipulate the data they hold. The length of a char array represents the number of elements within the array.
In this article, we will discuss three common methods to obtain the length of a char array in Java.
Get the Length of a Char Array in Java Using the length
Property
The length
property in Java is a member of an array that returns the number of elements (length) in the array. It is applicable to all array types, including char arrays.
For char arrays, the length
property provides the number of characters in the array.
To utilize this property, you simply access the length
property with the array variable you want to determine the length for, as shown below:
array.length
Here, array
is the variable representing the array.
Let’s create a complete example to demonstrate how to get the length of a char array using the length
property:
public class CharArrayLengthExample {
public static void main(String[] args) {
char[] charArray = {'H', 'e', 'l', 'l', 'o'};
int length = charArray.length;
System.out.println("Length of char array: " + length);
}
}
Output:
Length of char array: 5
In this example, we’ve created a char array charArray
with five characters and used the length
property to obtain the length of the array, which is then printed to the console.
Let’s see another example:
public class SimpleTesting {
public static void main(String[] args) {
try {
char[] ch = {'c', 'b', 'd', 'e', 'f', 'g'};
int length = ch.length;
System.out.println("Array Length = " + length);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Array Length = 6
As you can see, inside the main
method, a try-catch
block is used to handle potential exceptions. Within the try
block, a char array ch
is initialized with six specific characters.
The length
property of this char array is then obtained using ch.length
, which represents the number of characters in the array. Subsequently, a message displaying the array’s length is printed to the console using System.out.println()
.
In case an exception is thrown, it is caught by the catch
block, and the exception details are printed to the standard error stream using e.printStackTrace()
.
Get the Length of a Char Array Using a Loop in Java
We can also determine the length of a char array by using a loop. This is useful when you need to understand the process of how array length is determined and is particularly handy in scenarios where you may want to customize the counting process.
To determine the length of a char array using a loop, you can iterate through the array and count the number of elements until you reach the end of the array. Here’s a step-by-step approach to achieve this:
-
Initialize a variable to store the length of the char array and set it to 0 initially.
-
Iterate through each element of the char array using a loop.
-
For each iteration, increment the length variable by 1.
-
Once the loop has iterated through all elements in the char array, the length variable will hold the length of the array.
Let’s demonstrate this with a code example:
public class CharArrayLengthUsingLoop {
public static int getCharArrayLength(char[] charArray) {
int length = 0; // Initialize the length to 0
for (char c : charArray) {
length++;
}
return length;
}
public static void main(String[] args) {
char[] charArray = {'a', 'b', 'c', 'd', 'e', 'f'};
int arrayLength = getCharArrayLength(charArray);
System.out.println("Length of char array: " + arrayLength);
}
}
Output:
Length of char array: 6
In this example, the getCharArrayLength()
method takes a char array as a parameter and uses a for
loop to count the number of elements in the array by incrementing the length
variable. The main
method demonstrates how to use this method to get the length of a char array and prints the result.
Here’s another code example demonstrating a custom method to calculate the length of a char array:
public class SimpleTesting {
public static void main(String[] args) {
try {
char[] ch = {'c', 'b', 'd', 'e', 'f', 'g'};
int length = length(ch);
System.out.println("Array Length = " + length);
} catch (Exception e) {
e.printStackTrace();
}
}
static int length(final char[] b) {
int n = 0, t = 0;
while (true) {
try {
t = b[n++];
} catch (ArrayIndexOutOfBoundsException ex) {
n--;
break;
}
}
return n;
}
}
Output:
Array Length = 4
Here, we defined a custom method length()
to calculate the length of the char array ch
. We used a while
loop that iterates over the elements of the char array until an ArrayIndexOutOfBoundsException
is encountered.
It increments a counter (n
) and assigns the value of the array element at the current index to t
. If an exception occurs, it decrements n
and breaks out of the loop.
Get the Length of a Char Array Using the String Class in Java
Another approach we can use to determine the length of a char array is by using the String class constructor, which takes a char array as an argument. We can convert the char array to a String and then access the length()
method.
Here’s an example:
public class CharArrayLengthExample {
public static void main(String[] args) {
char[] charArray = {'H', 'e', 'l', 'l', 'o'};
String charArrayAsString = new String(charArray);
int length = charArrayAsString.length();
System.out.println("Length of the char array: " + length);
}
}
Output:
Length of the char array: 5
In this example, we first create a String charArrayAsString
by passing the char array charArray
to the String constructor. Then, we use the length()
method on charArrayAsString
to determine the length of the original char array.
Lastly, the length of the char array (or string) is printed to the console.
Conclusion
In summary, we have explored three commonly used methods for determining the length of the char array.
There’s the most straightforward and efficient way, which is directly accessing the length
property of the char array.
For those seeking a more manual approach, iterating through the elements in the char array using a loop and maintaining a counter allows you to determine the length.
Lastly, there is converting the char array into a String
and then utilizing the length()
method of the String
class.
Each of these methods serves its purpose and the choice of which one to use should be based on the specific requirements of your Java program.