How to Swap Arrays in Java
- Swap Two Arrays in Java Using Numeric Operators
- Swap Two Arrays in Java Using Bitwise Operators
-
Swap Two Arrays in Java Using
Collections.swap()
- Swap Two Arrays in Java Using a Temporary Variable
- Conclusion
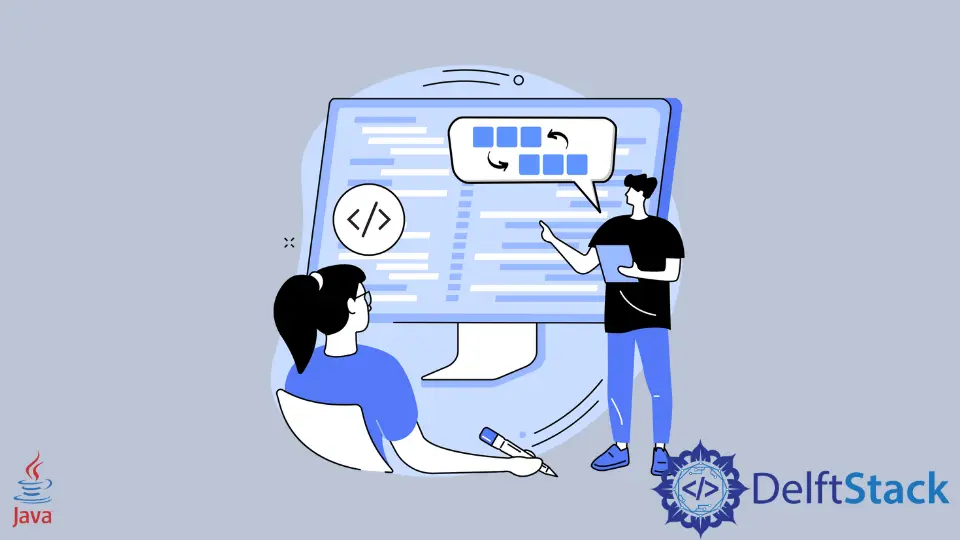
Swapping two arrays in Java can be a useful operation in various scenarios, whether you’re working on algorithmic problems or manipulating data structures.
In this article, we will explore different methods to swap two arrays in Java, such as numeric operators, bitwise operators, Collections.swap()
, and a temporary variable.
Swap Two Arrays in Java Using Numeric Operators
An efficient approach for swapping two arrays in Java is to utilize numeric operators for the swapping process.
The key idea behind using numeric operators for array swapping is to perform arithmetic operations on corresponding elements of the arrays. By strategically subtracting and adding elements, we can achieve the swap without the need for a third temporary variable.
Additionally, the absolute value is obtained to ensure correctness and prevent unexpected behavior when dealing with negative numbers.
Code Example: Swapping Two Arrays Using Numeric Operators
Here’s a simple example demonstrating how to swap two arrays in Java:
import java.util.Arrays;
public class SwapArrayNumeric {
public static void main(String[] args) throws Exception {
int[] a = {5, 9};
int[] b = {4, 3};
System.out.println("a[] before swapping : " + Arrays.toString(a));
System.out.println("b[] before swapping : " + Arrays.toString(b));
for (int i = 0; i < a.length; i++) {
a[i] = a[i] - b[i];
b[i] = a[i] + b[i];
a[i] = GetAbsoluteValue(a[i] - b[i]);
}
System.out.println("a[] after swapping : " + Arrays.toString(a));
System.out.println("b[] after swapping : " + Arrays.toString(b));
}
public static int GetAbsoluteValue(int a) {
return Math.abs(a);
}
}
In the provided Java code, we have two arrays, a
and b
, both containing integer values. The initial state of both arrays is printed using System.out.println
along with Arrays.toString(a)
and Arrays.toString(b)
.
Then, the arrays are swapped using a loop that iterates through each element. Within the loop, the corresponding elements of a
and b
undergo arithmetic operations to achieve the swap.
a[i] = a[i] - b[i];
b[i] = a[i] + b[i];
a[i] = GetAbsoluteValue(a[i] - b[i]);
The three lines of code above represent the steps in the swapping process:
a[i] - b[i]
: The value ofa[i]
is subtracted fromb[i]
, and the result is assigned toa[i]
.a[i] + b[i]
: The sum of the updateda[i]
and the originalb[i]
is assigned tob[i]
.GetAbsoluteValue(a[i] - b[i])
: The absolute value of the difference between the updateda[i]
andb[i]
is assigned toa[i]
. This step ensures that the result is positive, preventing unexpected behavior with negative numbers.
The method GetAbsoluteValue
is defined separately to calculate the absolute value using Math.abs
.
public static int GetAbsoluteValue(int a) {
return Math.abs(a);
}
Code Output:
As we can see, the output displays the arrays before and after the swapping operation, providing a clear illustration of the process. This demonstrates how the numeric operators effectively swap the elements between the two arrays in Java.
Swap Two Arrays in Java Using Bitwise Operators
An alternative method to swap two arrays in Java is utilizing bitwise operators. Bitwise XOR
(^
) is the key operator we’ll employ for this swapping technique.
Bitwise XOR
is a binary operator that returns a 1
in each bit position where the corresponding bits of the two operands are different and 0
where they are the same. In the context of array swapping, we leverage this property to efficiently swap elements without using a temporary variable.
The XOR
operation has the useful property that performing it twice with the same value restores the original value.
Code Example: Swapping Two Arrays Using Bitwise Operators
Here’s an example demonstrating how to swap two arrays in Java using bitwise operators:
import java.util.Arrays;
public class SwapArrayBitwise {
public static void main(String[] args) throws Exception {
int[] firstArr = {2, 4};
int[] secondArr = {6, 3};
System.out.println("firstArr before swapping : " + Arrays.toString(firstArr));
System.out.println("secondArr before swapping : " + Arrays.toString(secondArr));
for (int i = 0; i < firstArr.length; i++) {
firstArr[i] = firstArr[i] ^ secondArr[i];
secondArr[i] = firstArr[i] ^ secondArr[i];
firstArr[i] = firstArr[i] ^ secondArr[i];
}
System.out.println("firstArr after swapping : " + Arrays.toString(firstArr));
System.out.println("secondArr after swapping : " + Arrays.toString(secondArr));
}
}
In this Java program, two arrays, firstArr
and secondArr
, are declared and initialized with integer values. The initial state of both arrays is printed to the console.
int[] firstArr = {2, 4};
int[] secondArr = {6, 3};
System.out.println("firstArr before swapping : " + Arrays.toString(firstArr));
System.out.println("secondArr before swapping : " + Arrays.toString(secondArr));
A for
loop is employed to iterate through each element of the arrays. Within the loop, three bitwise XOR
operations are performed to swap the elements between the arrays.
for (int i = 0; i < firstArr.length; i++) {
firstArr[i] = firstArr[i] ^ secondArr[i];
secondArr[i] = firstArr[i] ^ secondArr[i];
firstArr[i] = firstArr[i] ^ secondArr[i];
}
These three lines of code represent the bitwise XOR
swapping process:
firstArr[i] ^ secondArr[i]
:XOR
operation to obtain theXOR
of corresponding elements.firstArr[i] ^ secondArr[i]
:XOR
operation to obtain the original value ofsecondArr[i]
.firstArr[i] ^ secondArr[i]
:XOR
operation to obtain the original value offirstArr[i]
.
This sequence effectively swaps the elements without using a temporary variable. The output of the swapped arrays is then printed to the console.
Code Output:
This showcases how bitwise XOR
operators provide an elegant and efficient solution for swapping elements between arrays in Java. The output verifies that the swapping process has occurred successfully.
Swap Two Arrays in Java Using Collections.swap()
Another method for swapping two arrays in Java is by leveraging the Collections.swap()
method. This approach is particularly useful when working with non-primitive arrays.
The Collections.swap()
method is part of the java.util.Collections
class and is designed for swapping elements at specified positions in a list. To use this method, we first need to convert our arrays into a list using Arrays.asList()
.
The syntax for Collections.swap()
is as follows:
Collections.swap(list, index1, index2);
Here, list
is the list containing the elements to be swapped, and index1
and index2
are the positions of the elements to be swapped.
Code Example: Swapping Two Arrays Using Collections.swap()
Here’s an example demonstrating how to swap two arrays in Java using Collections.swap()
:
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class SwapTwoElements {
public static void main(String[] args) throws Exception {
String[] firstArr = {"Hello", "World", "Happy", "Coding"};
System.out.println("firstArr before swapping : " + Arrays.toString(firstArr));
List<String> a = Arrays.asList(firstArr);
Collections.swap(a, 0, 2);
System.out.println("firstArr after swapping : " + Arrays.toString(firstArr));
}
}
Here, an array firstArr
is declared and initialized with string values. The initial state of the array is printed to the console.
String[] firstArr = {"Hello", "World", "Happy", "Coding"};
System.out.println("firstArr before swapping : " + Arrays.toString(firstArr));
Next, the array is converted into a list using Arrays.asList()
, and the Collections.swap()
method is called to swap the elements at positions 0 and 2.
List<String> a = Arrays.asList(firstArr);
Collections.swap(a, 0, 2);
It’s important to note that the swapping operation is directly reflected in the original array, firstArr
, since the array backs the list. The resulting state of firstArr
after swapping is then printed to the console.
Code Output:
This illustrates how Collections.swap()
provides a convenient and readable way to swap elements in arrays, especially when working with non-primitive types. The output confirms that the swapping operation has been applied to the original array.
Swap Two Arrays in Java Using a Temporary Variable
A straightforward and widely used method for swapping two arrays in Java involves using a temporary variable. This method is simple, easy to understand, and applicable to both primitive and non-primitive arrays.
The basic idea behind swapping arrays with a temporary variable is to assign the elements of one array to the temporary variable, then assign the elements of the second array to the first array, and finally assign the elements stored in the temporary variable to the second array.
The syntax for this process is as follows:
// Assuming arrays are of the same type and length
temp = array1;
array1 = array2;
array2 = temp;
Here, temp
is a temporary variable that holds the elements of one of the arrays during the swapping process.
Code Example: Swapping Two Arrays Using a Temporary Variable
Here’s an example demonstrating how to swap two arrays in Java using a temporary variable:
import java.util.Arrays;
public class SwapArrayWithTemp {
public static void main(String[] args) throws Exception {
int[] a = {5, 9};
int[] b = {4, 3};
System.out.println("a[] before swapping : " + Arrays.toString(a));
System.out.println("b[] before swapping : " + Arrays.toString(b));
// Swapping arrays using a temporary variable
int[] temp = a;
a = b;
b = temp;
System.out.println("a[] after swapping : " + Arrays.toString(a));
System.out.println("b[] after swapping : " + Arrays.toString(b));
}
}
In the Java code above, two arrays, a
and b
, are declared and initialized with integer values. The initial state of both arrays is printed to the console.
int[] a = {5, 9};
int[] b = {4, 3};
System.out.println("a[] before swapping : " + Arrays.toString(a));
System.out.println("b[] before swapping : " + Arrays.toString(b));
Next, the arrays are swapped using a temporary variable. The elements of array a
are assigned to the temporary variable, a
is then assigned the elements of array b
, and finally, b
is assigned the elements stored in the temporary variable.
int[] temp = a;
a = b;
b = temp;
This sequence effectively swaps the elements between arrays a
and b
using the temporary variable.
Code Output:
This illustrates how the swapping process using a temporary variable provides a straightforward and reliable solution for array manipulation in Java. The output confirms that the elements of arrays a
and b
have been successfully swapped.
Conclusion
In this article, we’ve explored multiple methods for swapping two arrays in Java, each with its strengths and considerations. The choice of method depends on factors such as memory efficiency, code readability, and specific project requirements.
Numeric and bitwise operators provide efficient solutions, while Collections.swap()
simplifies the process using utility classes. The classic use of a temporary variable prioritizes simplicity.
By understanding these techniques, you are better equipped to select the most appropriate approach for your array-swapping needs in Java. Whether prioritizing memory optimization or code simplicity, these methods offer versatile solutions for a common programming task.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn