Array Passed By Value or Passed By Reference in Java
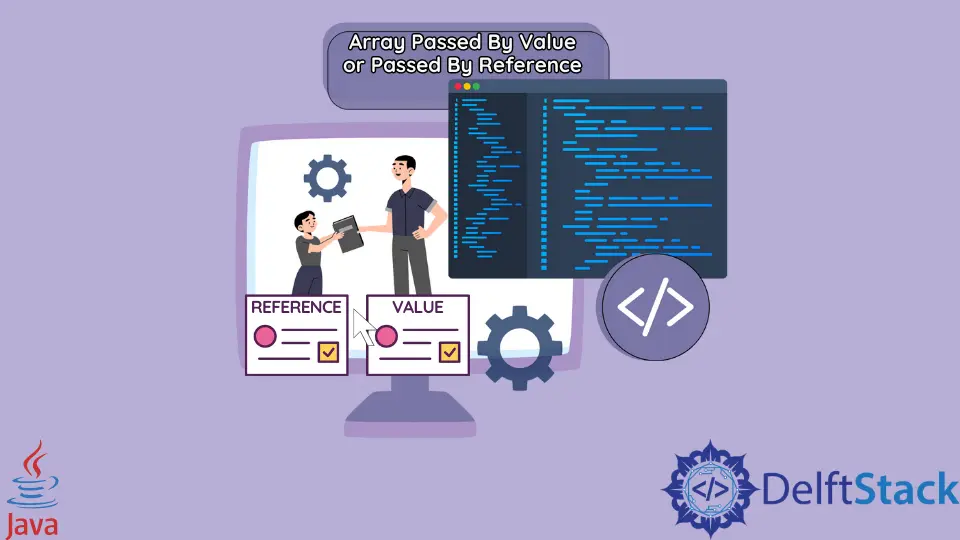
This tutorial introduces an array passed by value or reference in Java.
In this article, we’ll delve into the intricacies of array passing in Java, exploring the nuances of pass-by-value and pass-by-reference. Through practical examples and detailed explanations, we aim to demystify these concepts and provide clarity on their implications for array manipulation.
Passed by Value and Passed by Reference in Java
Passed by Value
In Java, passed by value is a fundamental concept in method parameter passing. When a primitive data type or an object reference is passed to a method, a copy of its value (for primitives) or the reference (for objects) is sent to the method.
This means that any modifications made to the parameter inside the method do not affect the original variable outside the method. Essentially, the method receives a snapshot of the value or reference, ensuring that changes made within the method do not impact the original data in the calling code.
While objects themselves are not passed directly, the reference to the object is passed by value, maintaining the integrity of the original object. Understanding this concept is crucial for effective programming and avoiding unexpected side effects when working with Java methods.
Passed by Reference
In Java, the term passed by reference is often misunderstood because Java strictly follows a passed by value approach. In Java, when an object is passed to a method, what is actually passed is the value of the reference to that object, not the object itself.
This means that the method receives a copy of the reference, allowing it to access and modify the object’s properties. However, unlike true pass-by-reference languages, modifications made to the reference itself, such as reassigning it to a new object, do not affect the original reference in the calling code.
In essence, Java is pass-by-value, but the subtlety lies in how this value is interpreted when dealing with objects—specifically, the value being the reference to the object rather than the object’s contents.
Let’s summarize the concepts of passed by value and passed by reference in Java:
Aspect | Passed by Value | Passed by Reference |
---|---|---|
Basic Idea | A copy of the actual value is passed to the method. | The reference (memory address) to the actual object is passed. |
Behavior with Primitives | Values of primitive data types are passed by value. | No applicable, as primitive data types do not have references. |
Behavior with Objects | The reference to the object is passed by value. | The reference to the object is passed, allowing modifications. |
Modification Effect | Changes made to the parameter inside the method do not affect the original value outside the method for primitives. | Changes made to the object inside the method affect the original object outside the method. |
Example Code | java int x = 5; modifyPrimitive(x); // No effect on x outside the method |
java int[] arr = {1, 2, 3}; modifyArray(arr); // Original array is modified outside the method |
Although Java is often said to be pass-by-value, it’s essential to understand that when objects are passed, it’s the reference to the object that is passed by value. This distinction can sometimes lead to confusion, as it may seem similar to pass-by-reference behavior in other languages.
Understanding Array Pass-by-Value and Pass-by-Reference in Java
In the realm of Java programming, the concepts of pass-by-value and pass-by-reference are fundamental to understanding how data is handled when passed to methods. These concepts are often a source of confusion, particularly when dealing with arrays.
In this article, we will explore how arrays work in Java, looking closely at pass-by-value and pass-by-reference. By using the example below, we’ll make it easier to understand how these concepts affect working with arrays.
public class ArrayPassingExample {
public static void main(String[] args) {
int[] myArray = {1, 2, 3, 4, 5};
// Passing the array by value
passArrayByValue(myArray);
System.out.println("After passing by value: " + arrayToString(myArray));
// Passing the array by reference
passArrayByReference(myArray);
System.out.println("After passing by reference: " + arrayToString(myArray));
}
public static void passArrayByValue(int[] arr) {
// Modifying the array within the method
for (int i = 0; i < arr.length; i++) {
arr[i] *= 2;
}
System.out.println("Inside passArrayByValue: " + arrayToString(arr));
}
public static void passArrayByReference(int[] arr) {
// Creating a new array and assigning it to the parameter
arr = new int[] {10, 20, 30, 40, 50};
System.out.println("Inside passArrayByReference: " + arrayToString(arr));
}
public static String arrayToString(int[] arr) {
StringBuilder result = new StringBuilder("[");
for (int i = 0; i < arr.length; i++) {
result.append(arr[i]);
if (i < arr.length - 1) {
result.append(", ");
}
}
result.append("]");
return result.toString();
}
}
In the initial step, we create an array named myArray
that holds integers ranging from 1 to 5. Following this, we explore two methods of array manipulation: passing by value and passing by reference.
In the first method, passArrayByValue
is invoked, passing the array as a parameter. Within the method, each element of the array is doubled, and the modified array is displayed both inside the method and after the method call in the main
method.
Subsequently, in the second method, passArrayByReference
is called, also passing the array as a parameter. Inside this method, a new array is generated and assigned to the parameter.
Similar to the first method, the modified array is printed within the method and after the method call in the main
method.
Output:
Inside passArrayByValue: [2, 4, 6, 8, 10]
After passing by value: [2, 4, 6, 8, 10]
Inside passArrayByReference: [10, 20, 30, 40, 50]
After passing by reference: [2, 4, 6, 8, 10]
Conclusion
The output demonstrates a key distinction between pass-by-value and pass-by-reference in Java. While pass-by-value allows modifications to the original array, pass-by-reference, when it comes to arrays, is limited in its ability to alter the original array reference.
Understanding these nuances is crucial for effective array manipulation and for avoiding common pitfalls when working with Java arrays in method calls.