How to Check an Array Contains a Particular Value in Java
-
Check an Array Contains a Particular Value Using the
array.contains()
Method in Java - Check an Array Contains a Particular Value Using Java 8 Streams
-
Check an Array Contains a Particular Value Using Simple
for
Loop
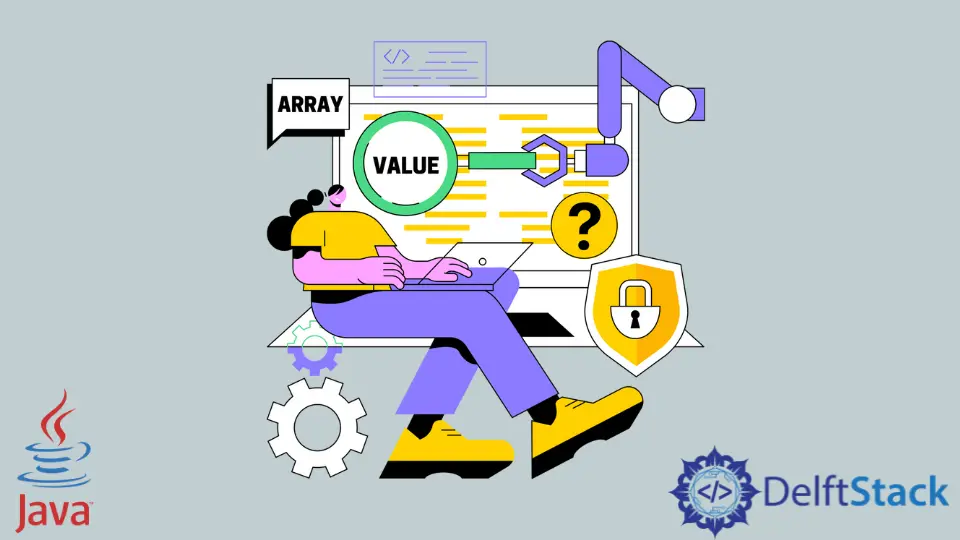
An array is a systematic collection of elements arranged in a definite order. Internally the array elements are stored in contiguous memory locations.
There are various ways to search an element in an array. Various methods lead to different times and complexity in them. Below are few listed ways to search an element in an array.
Check an Array Contains a Particular Value Using the array.contains()
Method in Java
In the below code block, we need to instantiate an array arr
with some predefined values. Now, initialize the string that we have to search.
Arrays
is a class that has various methods to manipulate the arrays. This class contains static factory methods and fields, and these are useful in searching and sorting elements in the array.
The asList()
method is the static method of the Arrays
class. It returns a fixed list of the specified array taken from the parameters.
contains()
is a method in the List
interface. It takes an element as a parameter, and we compare this argument with the elements present in the list. It returns the Boolean value true
if the specified element exists in the list and returns false
in either case. The method throws ClassCastException
if the type of the specified element is incompatible with this list and NullPointerException
if the specified value is null and this list does not permit a null value.
package findInList;
import java.util.Arrays;
public class FindAValueInArray {
public static void main(String[] args) {
String[] arr = new String[] {"1", "2", "3"};
String stringToSearch = "2";
System.out.println(Arrays.asList(arr).contains(stringToSearch));
}
}
The output of the above code is the same as the first code.
Check an Array Contains a Particular Value Using Simple for
Loop
In the below code block, we use the traditional for
loop to iterate over arrays. The for
loop is initialized in expression1
with a counter value as 0
. Now the counter value is compared with the length of an array. If the counter variable is smaller than the length of the defined array, then the statement inside the code block will get executed.
Inside the for
block, we compare each element of an array using the equals
method of the String
class. If the status evaluates to true, then the break
keyword is used to break the execution of the for
loop. If not found, the code will get executed as many times as the number of elements in the array.
After the loop, a print statement will evaluate the value of the status variable as true
if an element gets searched in the list, else would print the false
value.
package findInList;
public class FindAValueInArray {
public static void main(String[] args) {
String[] arr = new String[] {"1", "2", "3"};
String stringToSearch = "2";
boolean status = false;
for (int i = 0; i < arr.length; i++) {
status = arr[i].equals(stringToSearch);
if (status)
break;
}
System.out.println(status);
}
}
The output is again the same as the one given in the first code.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn