How to Copy Slice in Go
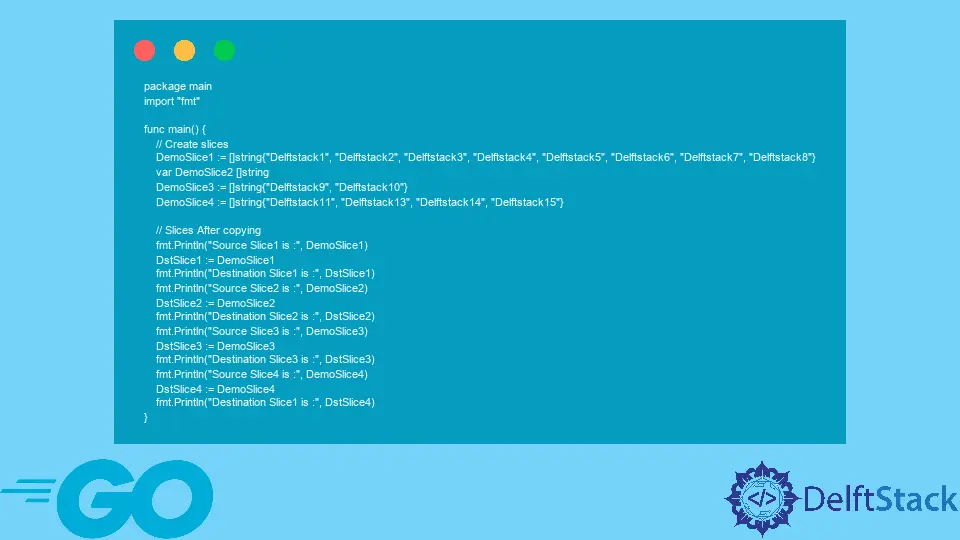
This tutorial demonstrates how to copy a slice in GoLang.
Copy Slice in GoLang
Copying a slice in GoLang can be achieved through different methods. The copy()
and append()
methods are usually used for this purpose, where the copy()
gets the deep copy of a given slice, and the append()
method will copy the content of a slice into an empty slice.
Use the Copy()
Method to Copy a Slice in Go
The copy()
method is considered the best method to copy a slice in the Golang because it creates a deep copy of the slice.
Syntax:
func copy(destination, source []Type) int
The destination is the copied slice, and the source is the slice from which we copy. The function returns the number of elements of the copied slice.
Code Example:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
DstSlice1 := make([]string, len(DemoSlice1))
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := copy(DstSlice1, DemoSlice1)
fmt.Println("\n Destination Slice 1 after copying:", DstSlice1)
fmt.Println("Total number of elements copied:", CopiedSlice1)
CopiedSlice2 := copy(DstSlice2, DemoSlice2)
fmt.Println("\n Destination Slice 2 after copying:", DstSlice2)
fmt.Println("Total number of elements copied:", CopiedSlice2)
CopiedSlice3 := copy(DstSlice3, DemoSlice3)
fmt.Println("\n Destination Slice 3 after copying:", DstSlice3)
fmt.Println("Total number of elements copied:", CopiedSlice3)
CopiedSlice4 := copy(DstSlice4, DemoSlice4)
fmt.Println("\n Destination Slice 4 after copying:", DstSlice4)
fmt.Println("Total number of elements copied:", CopiedSlice4)
}
The code above creates four source slices and four destination slices with different members or empty with the same length.
Output:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [ ]
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
Destination Slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Total number of elements copied: 8
Destination Slice 2 after copying: [Tutorials1]
Total number of elements copied: 0
Destination Slice 3 after copying: [Delftstack9 Delftstack10]
Total number of elements copied: 2
Destination Slice 4 after copying: [Delftstack11 Delftstack13 Delftstack14]
Total number of elements copied: 3
Program exited.
Use the Append()
Method to Copy a Slice in Go
The append method will append the content of a given slice to the destination slice.
Syntax:
func destination = append(destination, source...)
The append method will copy the content of the source slice to an empty destination slice or a slice with members. It will return the slice with previous and copied members, which is different from the copy method.
Code Example:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DstSlice1 []string
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := append(DstSlice1, DemoSlice1...)
fmt.Println("The copied slice 1 after copying: ", CopiedSlice1)
CopiedSlice2 := append(DstSlice2, DemoSlice2...)
fmt.Println("The copied slice 2 after copying: ", CopiedSlice2)
CopiedSlice3 := append(DstSlice3, DemoSlice3...)
fmt.Println("The copies slice 3 after copying: ", CopiedSlice3)
CopiedSlice4 := append(DstSlice4, DemoSlice4...)
fmt.Println("The copied slice 4 after copying: ", CopiedSlice4)
}
The code snippet above will copy a slice’s content to another.
Output:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : []
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
The copied slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
The copied slice 2 after copying: [Tutorials1]
The copies slice 3 after copying: [Tutorials1 Tutorials2 Delftstack9 Delftstack10]
The copied slice 4 after copying: [Tutorials1 Tutorials2 Tutorials3 Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited
Use the Assignment Method to Copy a Slice in Go
The assignment method copy is a shallow copy of a slice where we assign a source slice to the destination slice.
Code Example:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DemoSlice2 []string
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
// Slices After copying
fmt.Println("Source Slice1 is :", DemoSlice1)
DstSlice1 := DemoSlice1
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
DstSlice2 := DemoSlice2
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
DstSlice3 := DemoSlice3
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
DstSlice4 := DemoSlice4
fmt.Println("Destination Slice1 is :", DstSlice4)
}
As we can see, we assign the slices to other slices. This is a shallow method and not widely used because when we modify the content of copy, the original slice will also change.
Output:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Source Slice2 is : []
Destination Slice2 is : []
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Delftstack9 Delftstack10]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook