How to Create an Empty Slice in Go
- Initialize an Empty Slice in Go
-
Use
make()
to Create an Empty Slice in Go -
Use
new()
to Create an Empty Slice in Go - Conclusion
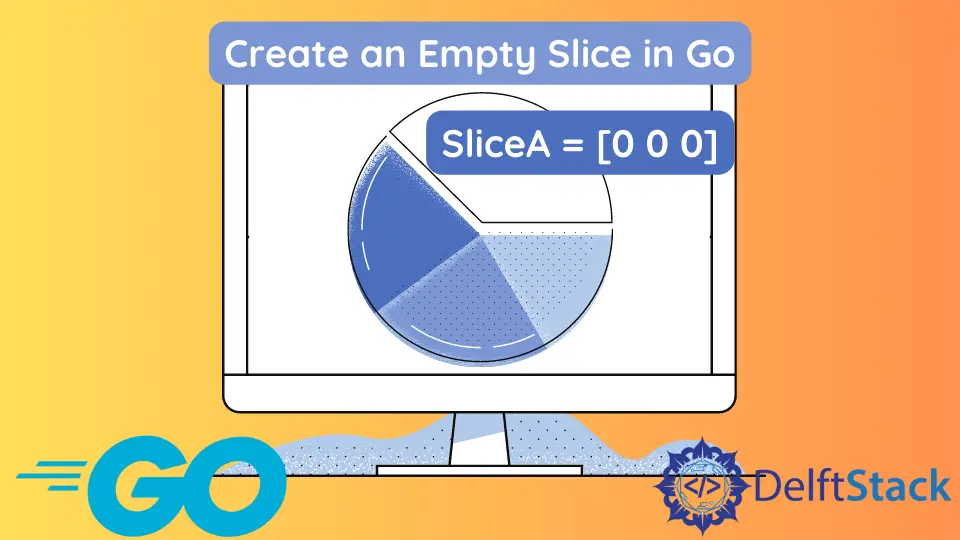
An empty slice, characterized by zero length and capacity, is a common requirement in programming.
In this tutorial, we’ll explore various methods to create empty slices in Go.
Initialize an Empty Slice in Go
Initializing an empty slice method or technique used to create a slice that has zero length and zero capacity represents an empty collection effectively. A slice in Go is a dynamically sized, flexible view into an underlying array, and it is a fundamental data structure used for working with sequences of elements.
We can initialize an empty slice in Go using the code below.
Example:
package main
import "fmt"
func main() {
b := []string{}
fmt.Println(b == nil)
}
In this code, we initialize an empty string slice denoted by b := []string{}
. The fmt.Println(b == nil)
statement checks whether the slice is nil
.
Here, we are using the comparison operator ==
to verify if the slice b
is a nil
slice.
Output:
false
Since an empty slice in Go is not nil
, the output of this code will be false
. This occurs because the empty slice b
has a zero length and zero capacity, representing an initialized but empty collection in Go.
Use make()
to Create an Empty Slice in Go
In Go, the make()
function is used to create slices, maps, and channels. When creating a slice with make()
, you can specify the initial length and capacity of the slice.
Basic Syntax:
make([]Type, length, capacity)
In the syntax, the Type
represents the data type of the elements in the slice, length
is the initial length of the slice, and capacity
is the initial capacity. For creating an empty slice, both length
and capacity
are set to zero.
Below are some examples of code using make()
.
Example 1:
package main
import "fmt"
func main() {
c := make([]string, 0)
fmt.Println(c == nil)
}
In this code, we create an empty string slice c
using the make()
function with a specified length of 0. The statement fmt.Println(c == nil)
checks if the slice is nil
.
Despite being an empty slice, the result of the comparison will be false
because a slice created using make()
is not nil
; it has been explicitly initialized. The make()
function allocates memory for the slice with a specified length and, if provided, capacity.
Output:
false
In this case, the length is set to 0, resulting in an empty but initialized slice, and hence, the output is false
.
Example 2:
package main
import "fmt"
func main() {
var sliceA = make([]int, 3, 6)
fmt.Printf("SliceA = %v, \nlength = %d, \ncapacity = %d\n",
sliceA, len(sliceA), cap(sliceA))
var sliceB = make([]int, 6)
fmt.Printf("SliceB = %v, \nlength = %d, \ncapacity = %d\n",
sliceB, len(sliceB), cap(sliceB))
}
In this code, we create two integer slices using the make()
function.
The first slice, sliceA
, is initialized with a length of 3 and a capacity of 6. The second slice, sliceB
, is initialized with a length and capacity both set to 6.
Then, we use the fmt.Printf()
function to print the values of both slices, their lengths, and their capacities.
Output:
SliceA = [0 0 0],
length = 3,
capacity = 6
SliceB = [0 0 0 0 0 0],
length = 6,
capacity = 6
The output demonstrates that sliceA
contains three zero-valued elements with a capacity of 6, while sliceB
contains six zero-valued elements with a matching length and capacity of 6. This illustrates the ability to specify initial lengths and capacities when using make()
to create slices in Go.
Use new()
to Create an Empty Slice in Go
The new()
function is employed for reserving memory for a new instance of a specified type, providing a pointer to the allocated memory as its result.
Basic Syntax:
variablePointer := new(Type)
In the syntax, the Type
represents the data type for which memory is being allocated. The variablePointer
is a variable that holds the memory address (pointer) to the newly allocated instance of the specified type.
However, using new()
alone is not sufficient to create an empty slice. Instead, you need to combine it with the make()
function to create an empty slice and obtain a pointer to it.
Example:
package main
import "fmt"
func main() {
var pointerToSlice = new([]float64)
*pointerToSlice = make([]float64, 0)
fmt.Println(*pointerToSlice == nil)
}
In this code, we declare a pointer variable pointerToSlice
to store the memory address of a float64
slice.
We use the new()
function to allocate memory for the slice and assign the pointer to the newly allocated memory. The line *pointerToSlice = make([]float64, 0)
initializes the slice with a length of 0, creating an empty slice.
Finally, we check if the pointer is pointing to a nil
slice with the statement fmt.Println(*pointerToSlice == nil)
.
Output:
false
The output of this code is false
since the slice, though empty, is not nil
; it has been explicitly initialized. This demonstrates the combination of new()
and make()
to create an empty slice and obtain a pointer to it in Go.
While using new()
in combination with make()
provides a way to create an empty slice and obtain a pointer to it, it’s worth noting that this approach is less common than other methods, such as using slice literals or the make()
function directly.
Conclusion
In conclusion, we’ve explored various methods for initializing empty slices in Go. Whether through the concise declaration of a slice literal, leveraging the versatile make()
function, or combining new()
and make()
for a pointer-based approach, we’ve demonstrated the flexibility of managing empty slices in Go.
Each method serves its purpose, offering developers a range of options based on specific programming requirements. As we navigate the dynamic landscape of Go programming, the ability to effortlessly create and manipulate empty slices empowers us to build efficient and scalable solutions.