How to Delete an Element From a Slice in Golang
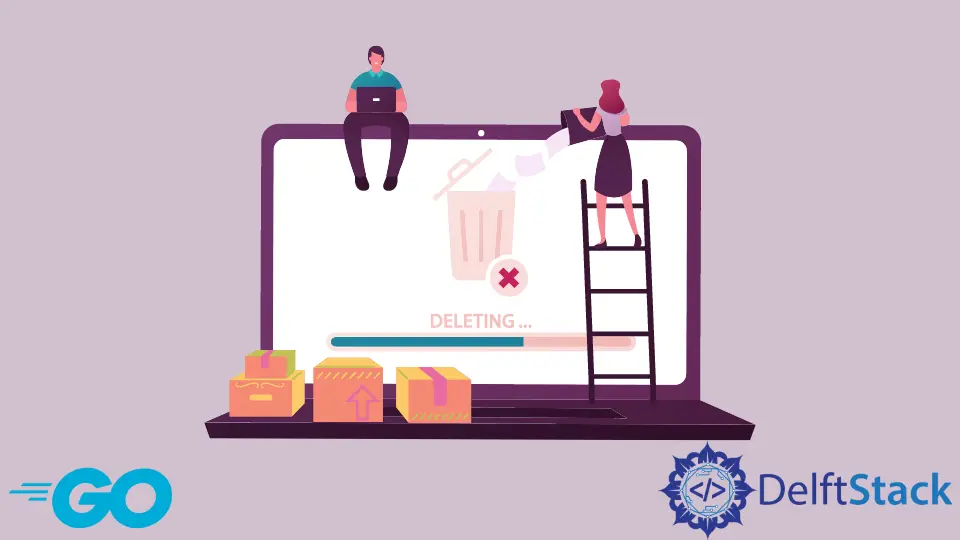
In Go, slice
is a variable-sized array that has indexing as of array but its size is not fixed as it can be resized. Any element from a slice
can be deleted from the slice
because of their dynamic nature. Deleting an element from a slice
is quite different from other languages due to the absence of built-in functions in Go to delete an element from a slice
.
Create Sub-Slices From a Given Slice
Before we dive deep into deleting an element from a slice
, we will have a look at creating subslice
from a given slice
as it is one of the main components for deleting an element from slice
.
package main
import "fmt"
func main() {
var Slice1 = []int{1, 2, 3, 4}
fmt.Printf("slice1: %v\n", Slice1)
Slice2 := Slice1[:2]
fmt.Printf("slice2: %v\n", Slice2)
}
Output:
slice1: [1 2 3 4]
slice2: [1 2]
Here, slice2
is a sub-slice formed from slice1
which contains all the elements from the beginning i.e. index 0
to index 2-1
i.e. 1
. Note here that indexing of slice
in Go starts from 0
.
package main
import "fmt"
func main() {
var Slice1 = []int{1, 2, 3, 4}
fmt.Printf("slice1: %v\n", Slice1)
Slice2 := Slice1[2:]
fmt.Printf("slice2: %v\n", Slice2)
}
Output:
slice1: [1 2 3 4]
slice2: [3 4]
Here, slice2
is a sub-slice formed from slice1
which contains all the elements from index 2
to end of the slice.
Delete Elements From Slice in Go
The task of deleting elements from slice
can be accomplished in different approaches based on our requirements. The various ways to delete an element from slice
are discussed below:
When the Order Is Important
If we wish to maintain the order of slices after removing the element, we shift the position of all elements at the right of deleted elements towards left by one.
package main
import "fmt"
func remove(slice []int, s int) []int {
return append(slice[:s], slice[s+1:]...)
}
func main() {
var Slice1 = []int{1, 2, 3, 4, 5}
fmt.Printf("slice1: %v\n", Slice1)
Slice2 := remove(Slice1, 2)
fmt.Printf("slice2: %v\n", Slice2)
}
Output:
slice1: [1 2 3 4 5]
slice2: [1 2 4 5]
Here slice2
is formed after removing the element at index 2
from slice1
.
When the Order Is Not Important
package main
import "fmt"
func remove(s []int, i int) []int {
s[i] = s[len(s)-1]
return s[:len(s)-1]
}
func main() {
var Slice1 = []int{1, 2, 3, 4, 5}
fmt.Printf("slice1: %v\n", Slice1)
Slice2 := remove(Slice1, 2)
fmt.Printf("slice2: %v\n", Slice2)
}
Output:
slice1: [1 2 3 4 5]
slice2: [1 2 5 4]
Here slice2
is formed after removing the element at index 2
from slice1
but the order of elements is not preserved here. It is faster than the previous method.
So, when the order has a higher priority than the speed, we use the first method and if the speed has a higher priority than the order we use the second method to delete an element from a slice
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn