How to Check if a Slice Contains an Element in Golang
- Method 1: Using a Simple Loop
- Method 2: Using a Map for Faster Lookups
-
Method 3: Using the
sort
Package and Binary Search - Conclusion
- FAQ
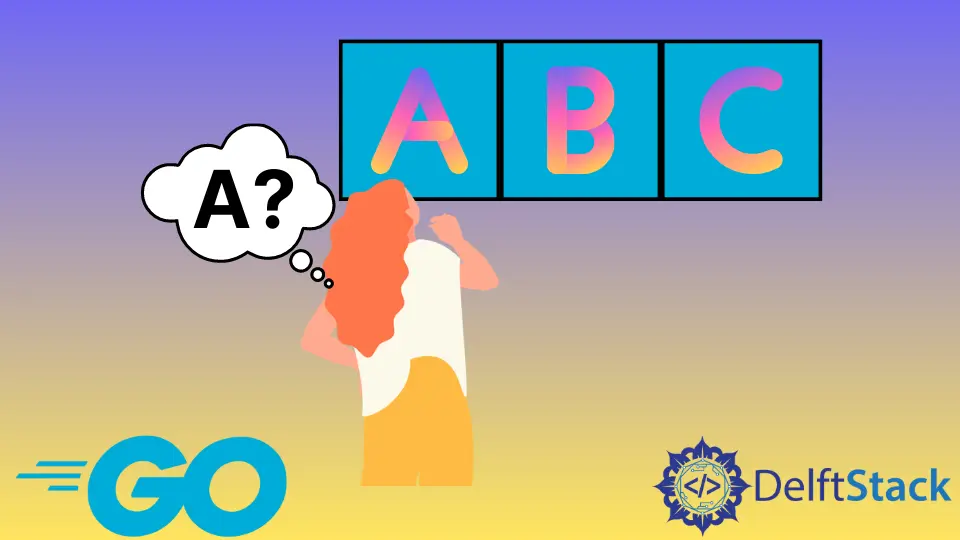
In the world of programming, checking for the existence of an element in a data structure is a common task. In Golang, slices are versatile and commonly used, but they don’t come with built-in methods to check for element presence.
This tutorial will guide you through various methods to determine if a slice contains a specific element in Golang. Whether you’re a beginner or an experienced developer, understanding how to efficiently check for element existence in slices can enhance your coding skills and improve the performance of your applications. Let’s dive into the methods that will help you accomplish this task effectively.
Method 1: Using a Simple Loop
One of the most straightforward ways to check if an element exists in a slice is by using a simple loop. This method iterates through each element in the slice and compares it to the target element. If a match is found, you can return true; otherwise, return false after the loop completes.
Here’s how you can implement this approach in Golang:
package main
import "fmt"
func contains(slice []int, element int) bool {
for _, v := range slice {
if v == element {
return true
}
}
return false
}
func main() {
numbers := []int{1, 2, 3, 4, 5}
fmt.Println(contains(numbers, 3))
fmt.Println(contains(numbers, 6))
}
Output:
true
false
This method is efficient for small to medium-sized slices. The contains
function takes a slice of integers and an integer element to search for. It iterates through the slice using a for
loop, checking each element against the target. If it finds a match, it returns true
. If the loop completes without finding the element, it returns false
. This approach is easy to understand and implement, making it a popular choice for many developers.
Method 2: Using a Map for Faster Lookups
If you’re dealing with larger slices and performance is a concern, using a map can significantly speed up the process of checking for an element. By converting the slice into a map, you can take advantage of the constant time complexity for lookups.
Here’s how you can implement this method in Golang:
package main
import "fmt"
func contains(slice []int, element int) bool {
elementMap := make(map[int]struct{})
for _, v := range slice {
elementMap[v] = struct{}{}
}
_, exists := elementMap[element]
return exists
}
func main() {
numbers := []int{1, 2, 3, 4, 5}
fmt.Println(contains(numbers, 3))
fmt.Println(contains(numbers, 6))
}
Output:
true
false
In this method, we create a map called elementMap
where the keys are the elements of the slice. We then loop through the slice and add each element to the map. After populating the map, we can check for the existence of the target element in constant time. This method is particularly beneficial when you need to check for multiple elements, as the initial setup of the map only needs to be done once.
Method 3: Using the sort
Package and Binary Search
If your slice is sorted, you can use binary search to check for the existence of an element. This method is highly efficient, with a time complexity of O(log n), making it suitable for large datasets.
Here’s how to implement this approach in Golang:
package main
import (
"fmt"
"sort"
)
func contains(slice []int, element int) bool {
sort.Ints(slice)
index := sort.Search(len(slice), func(i int) bool {
return slice[i] >= element
})
return index < len(slice) && slice[index] == element
}
func main() {
numbers := []int{5, 1, 3, 2, 4}
fmt.Println(contains(numbers, 3))
fmt.Println(contains(numbers, 6))
}
Output:
true
false
In this method, we first sort the slice using sort.Ints()
. Then, we use sort.Search()
to find the index of the element. The search function checks if the current element is greater than or equal to the target. Finally, we verify if the index is within bounds and if the element at that index matches the target. This method is efficient for sorted slices but does require the overhead of sorting, which should be considered based on your use case.
Conclusion
Checking if a slice contains an element in Golang can be achieved through various methods, each with its own advantages and use cases. Whether you opt for a simple loop for clarity, a map for faster lookups, or binary search for efficiency with sorted data, understanding these approaches will enhance your programming toolkit. By mastering these techniques, you can write more efficient and effective Golang code. Experiment with these methods and find the one that best suits your needs!
FAQ
- How do I check if a string slice contains a specific string?
You can use a similar approach as shown in the examples, just replace the integer type with string type in the function signature.
-
Is there a built-in function in Golang to check for element existence in slices?
No, Golang does not provide a built-in function for this purpose, so you need to implement your own methods. -
Can I use the methods shown for slices of other types, like structs?
Yes, the methods can be adapted for slices of any type, including structs, by modifying the comparison logic accordingly. -
What is the time complexity of the loop method?
The time complexity of the loop method is O(n), where n is the number of elements in the slice. -
When should I use a map instead of a loop?
Use a map when you need to check for the existence of elements multiple times or when dealing with large slices, as it provides faster lookups.