Golang 카피 슬라이스
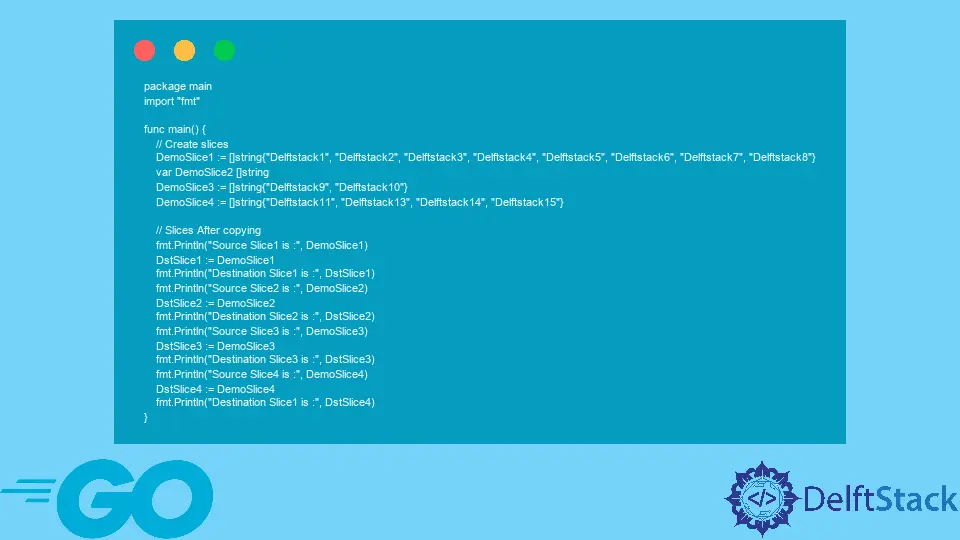
이 튜토리얼은 GoLang에서 슬라이스를 복사하는 방법을 보여줍니다.
GoLang에서 슬라이스 복사
GoLang에서 슬라이스를 복사하는 방법은 여러 가지가 있습니다. copy()
및 append()
메서드는 일반적으로 이러한 목적으로 사용됩니다. 여기서 copy()
는 주어진 슬라이스의 전체 복사본을 가져오고 append()
메서드는 빈 조각으로 자릅니다.
Copy()
메서드를 사용하여 Go에서 슬라이스 복사
copy()
메서드는 슬라이스의 깊은 복사본을 생성하기 때문에 Golang에서 슬라이스를 복사하는 가장 좋은 방법으로 간주됩니다.
통사론:
func copy(destination, source []Type) int
대상은 복사된 슬라이스이고 소스는 우리가 복사한 슬라이스입니다. 이 함수는 복사된 조각의 요소 수를 반환합니다.
코드 예:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
DstSlice1 := make([]string, len(DemoSlice1))
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := copy(DstSlice1, DemoSlice1)
fmt.Println("\n Destination Slice 1 after copying:", DstSlice1)
fmt.Println("Total number of elements copied:", CopiedSlice1)
CopiedSlice2 := copy(DstSlice2, DemoSlice2)
fmt.Println("\n Destination Slice 2 after copying:", DstSlice2)
fmt.Println("Total number of elements copied:", CopiedSlice2)
CopiedSlice3 := copy(DstSlice3, DemoSlice3)
fmt.Println("\n Destination Slice 3 after copying:", DstSlice3)
fmt.Println("Total number of elements copied:", CopiedSlice3)
CopiedSlice4 := copy(DstSlice4, DemoSlice4)
fmt.Println("\n Destination Slice 4 after copying:", DstSlice4)
fmt.Println("Total number of elements copied:", CopiedSlice4)
}
위의 코드는 멤버가 다르거나 길이가 같은 비어 있는 4개의 소스 슬라이스와 4개의 대상 슬라이스를 만듭니다.
출력:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [ ]
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
Destination Slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Total number of elements copied: 8
Destination Slice 2 after copying: [Tutorials1]
Total number of elements copied: 0
Destination Slice 3 after copying: [Delftstack9 Delftstack10]
Total number of elements copied: 2
Destination Slice 4 after copying: [Delftstack11 Delftstack13 Delftstack14]
Total number of elements copied: 3
Program exited.
Append()
메서드를 사용하여 Go에서 슬라이스 복사
append 메소드는 주어진 슬라이스의 내용을 대상 슬라이스에 추가합니다.
통사론:
func destination = append(destination, source...)
추가 방법은 소스 조각의 내용을 빈 대상 조각이나 구성원이 있는 조각에 복사합니다. 복사 방법과 다른 이전 및 복사된 구성원이 있는 슬라이스를 반환합니다.
코드 예:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DstSlice1 []string
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := append(DstSlice1, DemoSlice1...)
fmt.Println("The copied slice 1 after copying: ", CopiedSlice1)
CopiedSlice2 := append(DstSlice2, DemoSlice2...)
fmt.Println("The copied slice 2 after copying: ", CopiedSlice2)
CopiedSlice3 := append(DstSlice3, DemoSlice3...)
fmt.Println("The copies slice 3 after copying: ", CopiedSlice3)
CopiedSlice4 := append(DstSlice4, DemoSlice4...)
fmt.Println("The copied slice 4 after copying: ", CopiedSlice4)
}
위의 코드 스니펫은 슬라이스의 내용을 다른 것으로 복사합니다.
출력:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : []
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
The copied slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
The copied slice 2 after copying: [Tutorials1]
The copies slice 3 after copying: [Tutorials1 Tutorials2 Delftstack9 Delftstack10]
The copied slice 4 after copying: [Tutorials1 Tutorials2 Tutorials3 Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited
할당 방법을 사용하여 Go에서 슬라이스 복사
복사 할당 방법은 소스 슬라이스를 대상 슬라이스에 할당하는 슬라이스의 얕은 복사본입니다.
코드 예:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DemoSlice2 []string
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
// Slices After copying
fmt.Println("Source Slice1 is :", DemoSlice1)
DstSlice1 := DemoSlice1
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
DstSlice2 := DemoSlice2
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
DstSlice3 := DemoSlice3
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
DstSlice4 := DemoSlice4
fmt.Println("Destination Slice1 is :", DstSlice4)
}
보시다시피 슬라이스를 다른 슬라이스에 할당합니다. 이것은 얕은 방법이며 복사 내용을 수정하면 원본 조각도 변경되기 때문에 널리 사용되지 않습니다.
출력:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Source Slice2 is : []
Destination Slice2 is : []
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Delftstack9 Delftstack10]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook