GoLang Sort Slice of Structs
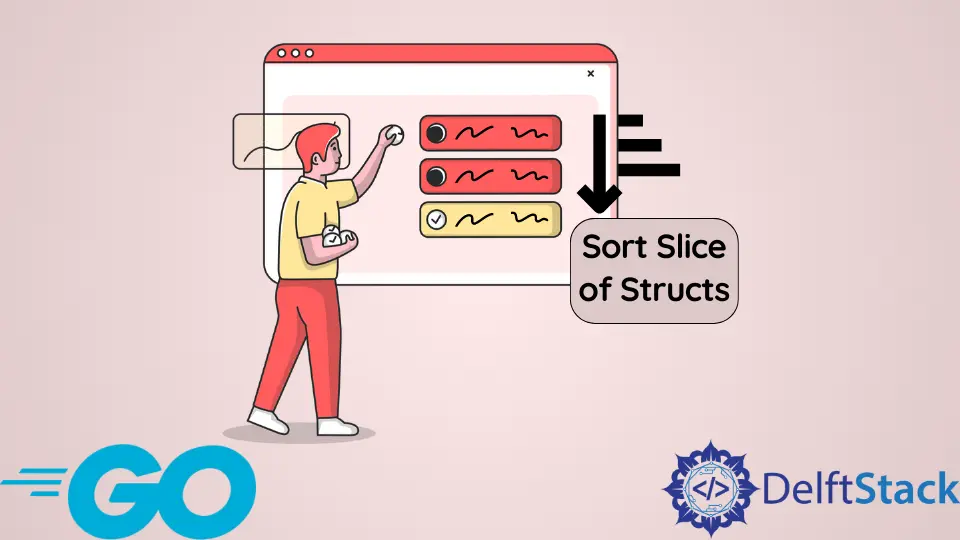
이 튜토리얼은 GoLang에서 구조체 조각을 정렬하는 방법을 보여줍니다.
GoLang Sort Slice of Structs
GoLang은 구조체 조각을 정렬하는 두 가지 방법을 제공합니다. 하나는 sort.Slice
이고 다른 하나는 sort.SliceStable
입니다. 또한 이 두 가지 방법과 함께 less
함수를 사용하여 구조체 조각을 정렬해야 합니다.
이러한 메서드의 구문은 다음과 같습니다.
//sort.Slice
func Slice(x StructSlice, less func(i, j int) bool)
//sort.SliceStable
func Slice(x StructSlice, less func(i, j int) bool)
여기서 x
는 구조체의 슬라이스이고 슬라이스는 less
기능을 기준으로 정렬됩니다. GoLang에서 구조체 조각을 정렬하는 방법을 이해하기 위해 몇 가지 예를 구현해 보겠습니다.
필드별로 구조체 조각 정렬
구조체 조각을 필드별로 정렬하는 것은 구조체 조각을 정렬하는 기본 예입니다. 구조체 슬라이스의 멤버 값을 필드를 기반으로 하는 동일한 구조체 슬라이스의 다른 멤버와 비교하는 경우 예를 살펴보겠습니다.
package main
import (
"fmt"
"sort"
)
type Employee struct {
Name string
Salary int
}
func main() {
// Sort in Ascending order
employees := []Employee{
{Name: "John", Salary: 1500},
{Name: "Joe", Salary: 3000},
{Name: "Jack", Salary: 3400},
}
sort.Slice(employees, func(i, j int) bool {
return employees[i].Salary < employees[j].Salary
})
fmt.Println(employees)
// Sort in Descending order
employees1 := []Employee{
{Name: "John", Salary: 1500},
{Name: "Joe", Salary: 3000},
{Name: "Jack", Salary: 3400},
}
sort.Slice(employees1, func(i, j int) bool {
return employees1[i].Salary > employees1[j].Salary
})
fmt.Println(employees1)
}
위의 코드에는 Name
과 Salary
라는 두 개의 필드가 있는 Employee
구조체가 포함되어 있습니다. 그런 다음 구조체 조각을 만들고 Slice
메서드를 사용하여 Salary
필드를 기준으로 오름차순으로 정렬합니다. 다른 슬라이스를 내림차순으로 정렬합니다.
출력을 참조하십시오.
[{John 1500} {Joe 3000} {Jack 3400}]
[{Jack 3400} {Joe 3000} {John 1500}]
이제 파일 이름과 값의 키-값 쌍(맵)을 포함하는 이러한 유형의 슬라이스에서 sort.SliceStable
을 사용할 수 없습니다. sort.SliceStable
을 사용하면 값만 포함하는 간단한 구조체 조각을 정렬할 수 있습니다.
package main
import (
"fmt"
"sort"
)
type Employee struct {
Name string
Salary int
}
func main() {
// Sort in Ascending order
employees := []Employee{
{"John", 1500},
{"Joe", 3000},
{"Jack", 3400},
}
sort.SliceStable(employees, func(i, j int) bool {
return employees[i].Salary < employees[j].Salary
})
fmt.Println(employees)
// Sort in Descending order
employees1 := []Employee{
{"John", 1500},
{"Joe", 3000},
{"Jack", 3400},
}
sort.SliceStable(employees1, func(i, j int) bool {
return employees1[i].Salary > employees1[j].Salary
})
fmt.Println(employees1)
}
위의 코드는 구조체 슬라이스에 이름과 값의 키-값 쌍이 없는 필드를 기반으로 구조체 슬라이스를 유사하게 정렬합니다.
출력을 참조하십시오.
[{John 1500} {Joe 3000} {Jack 3400}]
[{Jack 3400} {Joe 3000} {John 1500}]
여러 필드로 구조체 조각 정렬
여러 필드로 구조체 조각을 정렬하는 것도 가능합니다. less
기능을 확장해야 합니다. 예를 들어 보겠습니다.
package main
import (
"fmt"
"sort"
)
type Employee struct {
Name string
Position string
}
func main() {
// Sort in Descending order
employees := []Employee{
{"Michael", "Developer"},
{"Jack", "Manager"},
{"Joe", "CEO"},
{"Leonard", "Intern"},
{"Sheldon", "Developer"},
}
sort.SliceStable(employees, func(i, j int) bool {
if employees[i].Position != employees[j].Position {
return employees[i].Position < employees[j].Position
}
return employees[i].Name < employees[j].Name
})
fmt.Println(employees)
// Sort in Ascending order
employees1 := []Employee{
{"Michael", "Developer"},
{"Jack", "Manager"},
{"Joe", "CEO"},
{"Leonard", "Intern"},
{"Sheldon", "Developer"},
}
sort.SliceStable(employees1, func(i, j int) bool {
if employees1[i].Position != employees1[j].Position {
return employees1[i].Position > employees1[j].Position
}
return employees1[i].Name > employees1[j].Name
})
fmt.Println(employees1)
}
위의 코드는 이름
과 직위
를 기준으로 정렬합니다. 여기서 두 직원의 직위가 동일한 경우 이름
필드에 따라 정렬됩니다. 구조체의 슬라이스는 내림차순 및 오름차순으로 정렬됩니다.
출력을 참조하십시오.
[{Joe CEO} {Michael Developer} {Sheldon Developer} {Leonard Intern} {Jack Manager}]
[{Jack Manager} {Leonard Intern} {Sheldon Developer} {Michael Developer} {Joe CEO}]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook관련 문장 - Go Slice
- Golang의 슬라이스에서 요소를 삭제하는 방법
- Golang 카피 슬라이스
- Go에서 []String과 ...String의 차이점
- Go에서 빈 슬라이스 만들기
- 슬라이스에 Golang의 요소가 포함되어 있는지 확인