구조체의 Golang 배열
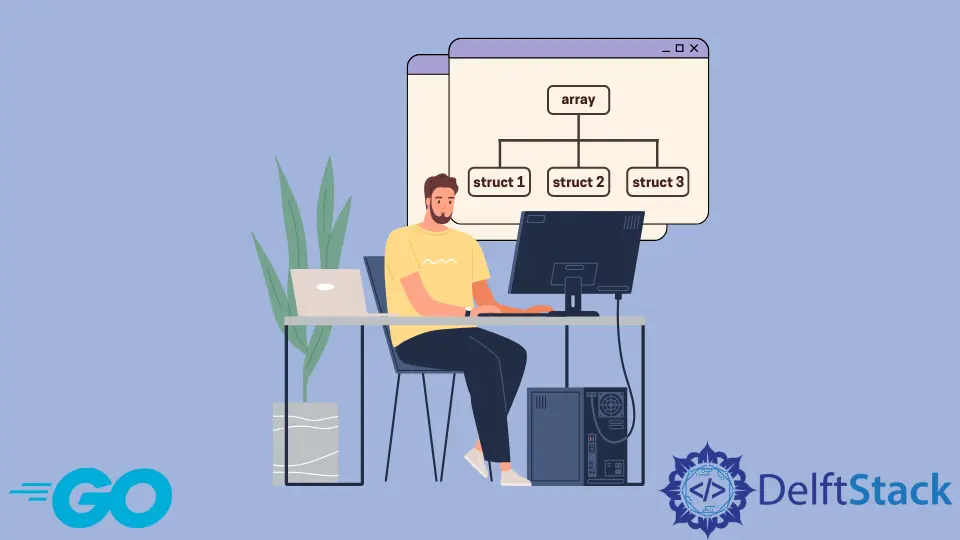
이 튜토리얼은 Golang에서 구조체 배열을 만들고 사용하는 방법을 보여줍니다.
Golang에서 구조체 배열 만들기
구조체는 Golang에서 사용자 정의 유형으로 간주되며, 다양한 유형의 데이터를 한 유형에 저장하는 데 사용됩니다. 이 개념은 일반적으로 클래스를 사용하여 여러 유형의 데이터 또는 해당 속성을 저장하는 OOP에서 사용됩니다.
Golang에서는 속성 집합이 있는 모든 실제 엔터티를 저장하는 구조체를 사용할 수 있습니다. Golang에는 배열의 구조체를 설정하는 기능이 있습니다.
예를 들어:
type Delftstack struct {
SiteName string
tutorials []tutorial
}
type tutorial struct {
tutorialName string
tutorialId int
tutorialLanguage string
}
위의 코드는 Delftstack
구조체 유형이 tutorial
구조체 유형의 슬라이스를 사용하는 것을 보여줍니다. 여기서 tutorial
구조체는 배열로 사용됩니다. 중첩 구조체로 간주할 수도 있습니다.
코드에서 구조체 배열을 사용하는 방법을 보여주는 예를 살펴보겠습니다.
package main
import "fmt"
type Delftstack struct {
SiteName string
tutorials []tutorial
}
type tutorial struct {
tutorialName string
tutorialId int
tutorialLanguage string
}
func main() {
PythonTutorial := tutorial{"Introduction to Python", 10, "Python"}
JavaTutorial := tutorial{"Introduction to Java", 20, "Java"}
GOTutorial := tutorial{"Introduction to Golang", 30, "Golang"}
tutorials := []tutorial{PythonTutorial, JavaTutorial, GOTutorial}
Delftstack := Delftstack{"Delftstack.com", tutorials}
fmt.Printf("The site with tutorials is %v", Delftstack)
}
위의 코드는 Delftstack
구조체를 초기화한 다음 Delftstack
구조체에서 tutorial
구조체 배열을 사용합니다. 마지막으로 tutorials
배열과 함께 사이트 이름을 인쇄합니다.
출력을 참조하십시오.
The site with tutorials is {Delftstack.com [{Introduction to Python 10 Python} {Introduction to Java 20 Java} {Introduction to Golang 30 Golang}]}
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook