Golang Array of Structs
- Understanding Structs in Golang
- Creating an Array of Structs
- Accessing Elements in an Array of Structs
- Modifying Elements in an Array of Structs
- Conclusion
- FAQ
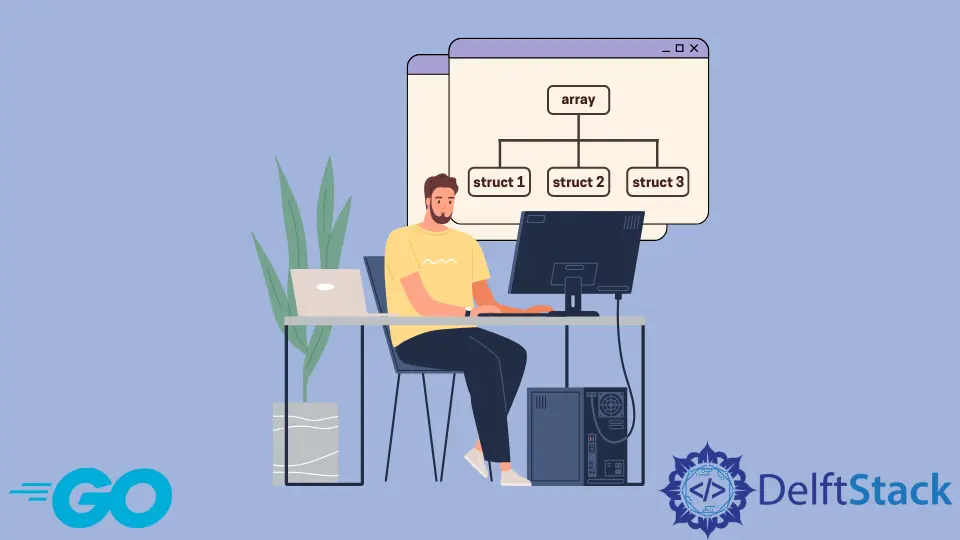
In the world of Golang, understanding how to create and use an array of structs is essential for efficient data management. Structs in Golang are composite data types that allow you to group variables of different types under a single entity. When you combine structs with arrays, you can create complex data structures that are both powerful and flexible.
This tutorial will guide you through the process of creating and using an array of structs in Golang, providing practical examples and explanations along the way. Whether you’re a beginner or looking to refine your skills, this guide will equip you with the knowledge you need to harness the power of arrays and structs in your Go applications.
Understanding Structs in Golang
Before diving into arrays of structs, it’s crucial to grasp what structs are in Golang. A struct is a user-defined type that allows you to encapsulate related data. For instance, if you want to represent a person, you might define a struct with fields like name, age, and email. Here’s a simple example of defining a struct in Golang:
type Person struct {
Name string
Age int
Email string
}
In this example, we define a struct named Person
with three fields. The Name
field is of type string, Age
is an integer, and Email
is also a string. This structure allows you to group related information about a person in a single entity.
Creating an Array of Structs
Once you have defined a struct, creating an array of that struct is straightforward. You can declare an array of structs just like you would with any other type. Here’s how you can create an array of Person
structs:
people := [3]Person{
{"Alice", 30, "alice@example.com"},
{"Bob", 25, "bob@example.com"},
{"Charlie", 35, "charlie@example.com"},
}
In this example, we declare an array named people
that can hold three Person
structs. Each struct is initialized with values for the Name
, Age
, and Email
fields. This array now contains three distinct Person
instances, each with its own set of data.
Output:
Array of structs created with three Person instances.
The power of using an array of structs lies in its ability to store multiple records of the same type. This is particularly useful when you need to manage collections of data, such as a list of users, products, or any other entities relevant to your application.
Accessing Elements in an Array of Structs
Accessing elements in an array of structs is similar to accessing elements in a regular array. You can use the index to retrieve specific structs and then access their fields. Here’s an example:
for i := 0; i < len(people); i++ {
fmt.Printf("Name: %s, Age: %d, Email: %s\n", people[i].Name, people[i].Age, people[i].Email)
}
In this code snippet, we use a loop to iterate through the people
array. For each Person
struct, we print out the Name
, Age
, and Email
. This demonstrates how you can easily access and manipulate data stored within an array of structs.
Output:
Name: Alice, Age: 30, Email: alice@example.com
Name: Bob, Age: 25, Email: bob@example.com
Name: Charlie, Age: 35, Email: charlie@example.com
This method is efficient for processing collections of data, allowing you to perform operations like filtering, sorting, or aggregating information based on the fields of the structs.
Modifying Elements in an Array of Structs
Modifying an element in an array of structs is just as simple as accessing it. You can directly assign new values to the fields of a struct at a specific index. Here’s how you can update the age of a person in the people
array:
people[1].Age = 26
fmt.Printf("Updated Age of Bob: %d\n", people[1].Age)
In this example, we change Bob’s age from 25 to 26. After the modification, we print the updated age to confirm the change.
Output:
Updated Age of Bob: 26
This capability allows for dynamic updates to your data structures, making it easy to maintain and manipulate information as your application evolves.
Conclusion
Creating and using an array of structs in Golang is a powerful way to manage complex data. By defining structs, initializing arrays, and accessing or modifying their elements, you can build robust applications that handle various data types efficiently. Whether you’re building a simple application or a complex system, mastering arrays of structs will significantly enhance your programming skills in Golang. Keep experimenting with these concepts to deepen your understanding and improve your coding practices.
FAQ
-
What is a struct in Golang?
A struct in Golang is a composite data type that groups together variables of different types under a single entity. -
How do I create an array of structs in Golang?
You can create an array of structs by declaring an array and initializing it with instances of the struct type. -
Can I modify the fields of a struct in an array?
Yes, you can modify the fields of a struct in an array by accessing the struct using its index and assigning new values to its fields. -
What are the benefits of using arrays of structs?
Arrays of structs allow you to manage collections of related data efficiently, making it easier to perform operations like filtering, sorting, and aggregating. -
How do I access elements in an array of structs?
You can access elements in an array of structs using their index, just like you would with a regular array.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Go Struct
- How to Convert Go Struct to JSON
- How to Print Struct Variables in Console in Go
- How to Convert Struct to String in Golang
- How to Sort Slice of Structs in Go