How to Convert Struct to String in Golang
-
Use the
fmt.Sprintf
Method to Convert a Struct to a String in Go -
Use the
json.Marshal
Method to Convert a Struct to JSON String in Go - Use Reflection to Convert a Struct to String in Go
- Conclusion
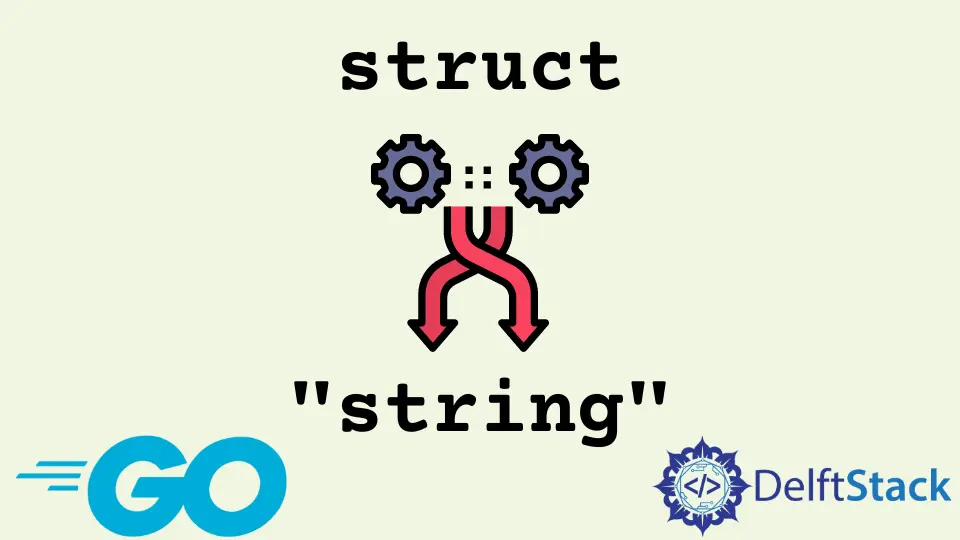
When working with Go, converting a struct to a string is a common requirement in various scenarios, ranging from debugging to serialization. While Go doesn’t have a direct method to convert a struct to a string like some other programming languages, it offers multiple approaches to achieve this conversion effectively.
In this article, we’ll explore diverse methods and techniques for converting a struct to a string in Go. Each method brings its strengths and considerations, catering to different use cases and preferences of developers.
From leveraging the fmt
package for string formatting or utilizing JSON encoding to employing the power of reflection, we’ll delve into detailed examples and explanations for each method. By the end of this article, you’ll have a clear understanding of various methodologies available in Go for struct-to-string conversions, empowering you to choose the most suitable approach for your specific development needs.
Use the fmt.Sprintf
Method to Convert a Struct to a String in Go
fmt.Sprintf
is a function within Go’s fmt
package that returns a formatted string. It functions similarly to Printf
, but instead of printing the output to the standard output, it returns the formatted string.
Syntax:
func Sprintf(format string, a ...interface{}) string
Parameters:
format string
: This is the format string that specifies how subsequent arguments should be formatted. It contains plain text along with format verbs (such as%s
,%d
,%f
, etc.) that are replaced by the corresponding values in subsequent arguments.a ...interface{}
: This is a variadic parameter that represents a variable number of arguments of typeinterface{}
. These arguments are the values that correspond to the format specifiers in theformat
string.
Return Value:
string
: Thefmt.Sprintf
method returns a string resulting from the formatting operation specified by theformat
string and the subsequent arguments.
fmt.Sprintf
is a versatile method for formatting strings in Go. It’s handy for converting a struct to a string by utilizing format specifiers.
Example:
package main
import "fmt"
type myStructure struct {
bar string
}
func (f myStructure) String() string {
return fmt.Sprintf("The structure I made has the following data: %s", f.bar)
}
func main() {
fmt.Println(myStructure{"Hello, World! GoLang is fun!"})
}
Output:
The structure I made has the following data: Hello, World! GoLang is fun!
The code showcases how to customize a Go struct’s string representation using fmt.Sprintf()
and the String()
method. It defines a myStructure
struct with a single string field, bar
.
The String()
method, overriding the default behavior, formats a string combining a preset message and the bar
field’s value using fmt.Sprintf()
. In the main()
function, an instance of myStructure
is created with the string "Hello, World! GoLang is fun!"
assigned to bar
.
Upon calling fmt.Println()
with this instance, the customized String()
method is automatically invoked, presenting the formatted string representation of the myStructure
instance.
This illustrates how the String()
method can control and tailor a struct’s output when using formatting functions in Go.
Use the json.Marshal
Method to Convert a Struct to JSON String in Go
Go’s standard library includes a powerful JSON encoder and decoder, which can be used to convert a struct to a JSON string. The json.Marshal
method from the encoding/json
package is used to serialize data into JSON format.
The syntax of json.Marshal
is as follows:
func Marshal(v interface{}) ([]byte, error)
Parameters:
v
: This parameter represents the value to be encoded into JSON. It accepts aninterface{}
type, which means it can accept any Go data structure that can be serialized into JSON, such as structs, maps, slices, etc.
Return values:
[]byte
: The method returns a byte slice ([]byte
) containing the JSON-encoded data if the serialization is successful.error
: It also returns an error, which will be nil if the serialization succeeds. If there’s an issue during serialization, the error will describe the problem encountered.
Example Code:
package main
import (
"encoding/json"
"fmt"
)
func main() {
type MyStructure struct {
Message string `json:"From Structure"`
}
val := &MyStructure{
Message: "Hello, World!",
}
// convert struct to json string
jsonBytes, err := json.Marshal(val)
fmt.Println(string(jsonBytes), err)
}
Output:
{"From Structure":"Hello, World!"} <nil>
This code snippet demonstrates how to convert a custom Go struct (MyStructure
) into its JSON representation using the json.Marshal()
function. It showcases the process of encoding a struct into a JSON string, providing a way to serialize Go data for various purposes such as data interchange, storage, or communication with other systems that support JSON.
Note that while using the above method, only exported fields of the defined struct are available to the external library. Hence, only the export fields of our struct will be copied in the converted JSON string.
Use Reflection to Convert a Struct to String in Go
Reflection in Go allows examining and manipulating variables at runtime. It can be employed to convert a struct to a string by iterating through its fields.
Reflection in Go provides the ability to examine the type, structure, and values of variables at runtime. The reflect
package equips developers with functions and types to introspect and manipulate variables dynamically, allowing operations like inspecting field names and values within a struct.
Consider a scenario where a generic approach is required to convert any given struct to a string without prior knowledge of its fields. Reflection enables us to achieve this by iteratively examining and retrieving the fields and their values.
package main
import (
"fmt"
"reflect"
)
type Person struct {
Name string
Age int
}
func structToString(s interface{}) string {
val := reflect.ValueOf(s)
if val.Kind() != reflect.Struct {
return ""
}
var str string
for i := 0; i < val.NumField(); i++ {
str += fmt.Sprintf("%s: %v, ", val.Type().Field(i).Name, val.Field(i))
}
return str[:len(str)-2]
}
func main() {
person := Person{Name: "Charlie", Age: 35}
fmt.Println(structToString(person))
}
Output:
Name: Charlie, Age: 35
This code showcases a function called structToString
that utilizes reflection to convert any given struct into a string representation. The function takes an empty interface as input, inspects the provided struct using reflection from the reflect
package, and iterates through its fields.
By dynamically retrieving field names and values, it constructs a string representation of the struct. The main()
function demonstrates this functionality by creating an instance of a Person
struct, populating it with name and age values, and then printing the resulting string representation obtained via the structToString
function.
This method illustrates the flexibility of reflection in Go, enabling the handling and conversion of struct data to strings dynamically, regardless of the specific struct’s composition.
Conclusion
This article delves into multiple techniques for converting structs to strings in Go, catering to various needs in development.
fmt.Sprintf
: Offers a customizable method using format specifiers, enabling the creation of specific string representations for structs.json.Marshal
: Allows easy serialization of structs into JSON strings, facilitating data interchange and storage, though limited to exported fields.Reflection
: Empowers a generic approach to convert any struct to a string dynamically. By inspecting fields and values at runtime, it provides flexibility without prior knowledge of the struct’s structure.
Each method showcased its unique strengths, from simplicity and customizability to dynamic handling, catering to different use cases in Go programming. Choosing the appropriate method depends on specific requirements, whether it’s for formatting, serialization, or dynamic struct handling.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn