How to Print Struct Variables in Console in Go
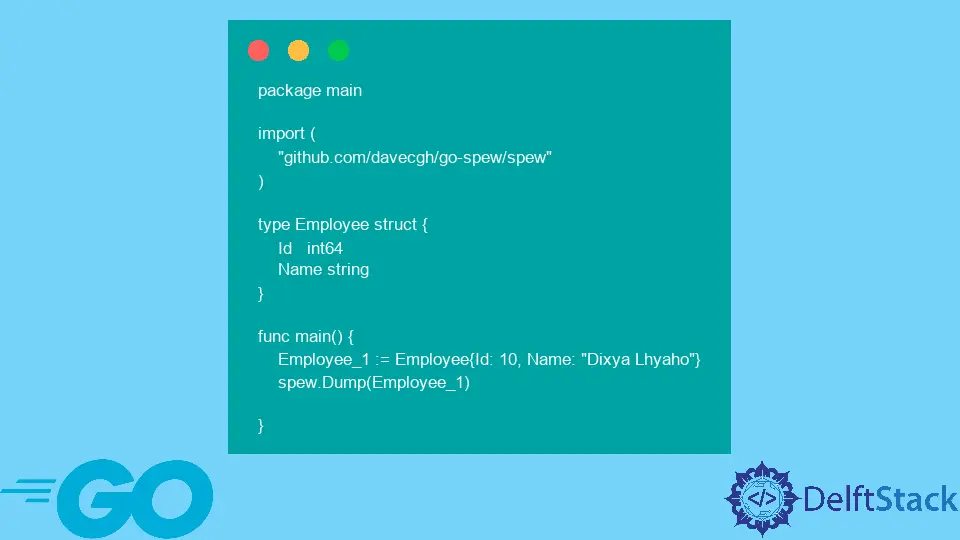
In Go, struct
is a collection of different fields of the same or different data types. A struct
is similar to the class in the Object-Oriented Programming paradigm. We can print structs using Printf
function of package fmt
along with special tags as arguments to Printf
function. Similarly, we can also print structs using special packages such as encoding/json
, go-spew
, and Pretty Printer Library
.
Declare struct
in Go
Structures in Go are created using the struct
keyword.
package main
import "fmt"
type info struct {
Name string
Address string
Pincode int
}
func main() {
a1 := info{"Dikhsya Lhyaho", "Jhapa", 123}
fmt.Println("Info of Dikhsya: ", a1)
}
Output:
Info of Dikhsya: {Dikhsya Lhyaho Jhapa 123}
We can print struct
variables with various packages in Go. Some of them are described below:
Printf
Function of fmt
Package
We can use the Printf
function of package fmt
with a special formatting option. The available formatting options to display variables using fmt
are:
Format | Description |
---|---|
%v |
Print the variable value in a default format |
%+v |
Add field names with the value |
%#v |
a Go-syntax representation of the value |
%T |
a Go-syntax representation of the type of the value |
%% |
a literal percent sign; It consumes no value |
Example Codes:
package main
import "fmt"
type Employee struct {
Id int64
Name string
}
func main() {
Employee_1 := Employee{Id: 10, Name: "Dixya Lhyaho"}
fmt.Printf("%+v\n", Employee_1) // with Variable name
fmt.Printf("%v\n", Employee_1) // Without Variable Name
fmt.Printf("%d\n", Employee_1.Id)
fmt.Printf("%s\n", Employee_1.Name)
}
Output:
{Id:10 Name:Dixya Lhyaho}
{10 Dixya Lhyaho}
10
Dixya Lhyaho
Marshal
Function of Package encoding/json
Another way is to use the function Marshal
of package encoding/json
.
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Id int64
Name string
}
func main() {
Employee_1 := Employee{Id: 10, Name: "Dixya Lhyaho"}
jsonE, _ := json.Marshal(Employee_1)
fmt.Println(string(jsonE))
}
Output:
{"Id":10,"Name":"Dixya Lhyaho"}
Dump
Function of Package go-spew
Another way is to use the Dump
function of package go-spew
.
.
package main
import (
"github.com/davecgh/go-spew/spew"
)
type Employee struct {
Id int64
Name string
}
func main() {
Employee_1 := Employee{Id: 10, Name: "Dixya Lhyaho"}
spew.Dump(Employee_1)
}
Output:
(main.Employee) {
Id: (int64) 10,
Name: (string) (len=12) "Dixya Lhyaho"
}
To install the go-spew
package run the following command in your terminal:
go get -u github.com/davecgh/go-spew/spew
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn