How to Convert Go Struct to JSON
- Convert Go Struct to JSON
- Convert Nested Structs to JSON in Go
- Indentation in Struct to JSON Conversion in Go
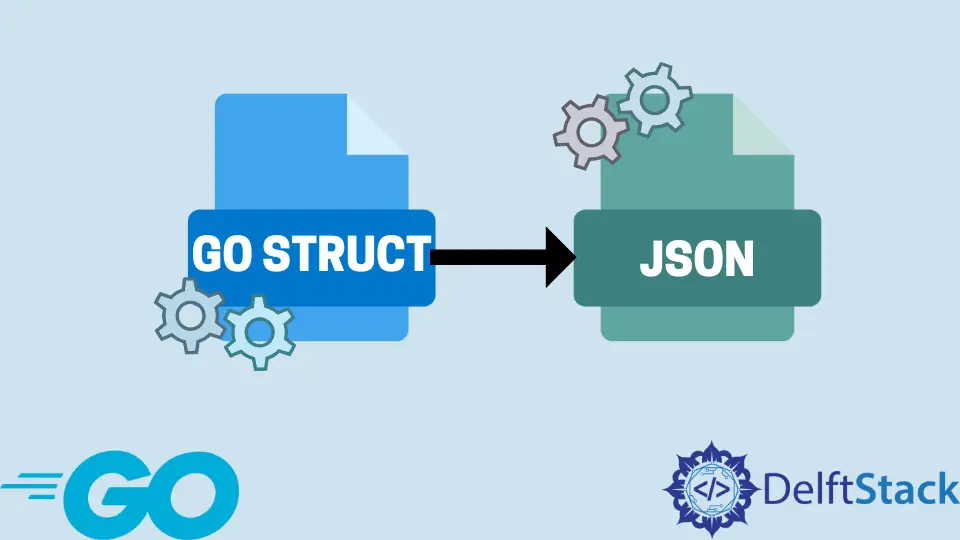
JSON is a lightweight language independent format for storing and transporting data. In Go, we have the encoding/json
package, which contains many inbuilt methods for JSON related operations. We can convert struct data into JSON using marshaling.
Convert Go Struct to JSON
The marshal
method receives struct object and returns a byte slice of the encoded data, which we can change to JSON in Go.
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Name string `json:"empname"`
Number int `json:"empid"`
}
func main() {
employee_1 := &Employee{Name: "Dixya Lhyaho", Number: 10}
e, err := json.Marshal(employee_1)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(e))
}
Output:
{"empname":"Dixya Lhyaho","empid":10}
If we change the Number
property to number
, number
is now ignored in the marshaling process as the json/encoding
package can’t see it.
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Name string `json:"empname"`
number int `json:"empid"`
}
func main() {
employee_1 := &Employee{Name: "Dixya Lhyaho", number: 10}
e, err := json.Marshal(employee_1)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(e))
}
Output:
{"empname":"Dixya Lhyaho"}
Convert Nested Structs to JSON in Go
Let’s define a more complex struct with nested struct. Within the definition of the struct, we define the JSON tags that map the fields of our structs directly to the fields in our marshaled JSON.
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Position string `json:"position"`
Name Name `json:"name"`
}
type Name struct {
FirstName string `json:"firstname"`
Surname string `json:"surname"`
}
func main() {
name := Name{FirstName: "Dikxya", Surname: "Lhyaho"}
employee := Employee{Position: "Senior Developer", Name: name}
byteArray, err := json.Marshal(employee)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(byteArray))
}
Output:
{"position":"Senior Developer","name":{"firstname":"Dikxya","surname":"Lhyaho"}}
Indentation in Struct to JSON Conversion in Go
We can use json.MarshalIndent()
function instead of the json.Marshal()
function to print JSON in a more readable format. We pass two additional arguments, prefix string
and the indent string
to MarshalIndent
.
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Position string `json:"position"`
Name Name `json:"name"`
}
type Name struct {
FirstName string `json:"firstname"`
Surname string `json:"surname"`
}
func main() {
name := Name{FirstName: "Dikxya", Surname: "Lhyaho"}
employee := Employee{Position: "Senior Developer", Name: name}
byteArray, err := json.MarshalIndent(employee, "", " ")
if err != nil {
fmt.Println(err)
}
fmt.Println(string(byteArray))
}
Output:
{
"position": "Senior Developer",
"name": {
"firstname": "Dikxya",
"surname": "Lhyaho"
}
}
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn