How to Convert JSON to Struct in Go
-
Use the
Unmarshal
Method to Convert JSON to Struct in Go - Convert an Array of JSON to Struct in Go
- Convert a Nested JSON to Struct in Go
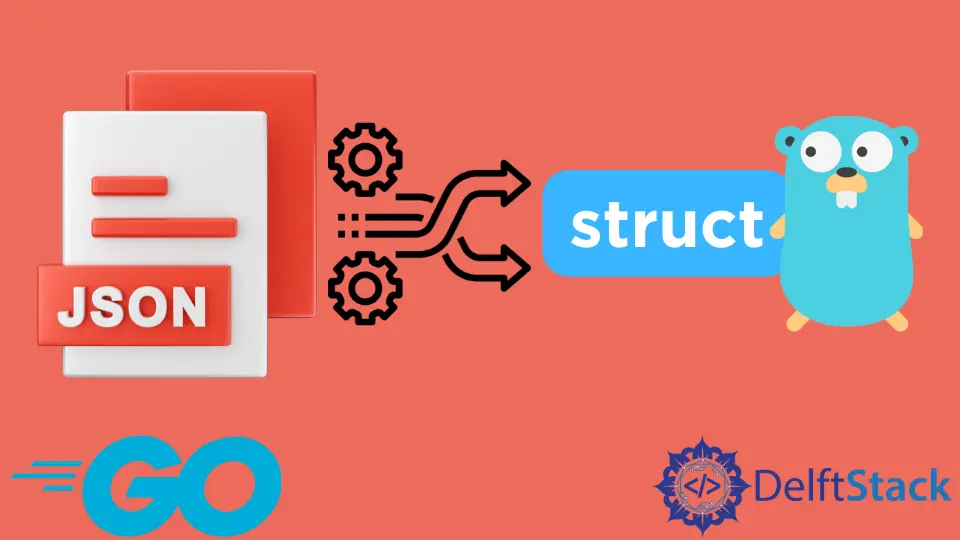
This tutorial demonstrates how to convert JSON to a struct in GoLang.
Use the Unmarshal
Method to Convert JSON to Struct in Go
The encoding/json
package of GoLang provides a function Unmarshal
, which converts JSON data to byte format. This function can parse the structured and non-structured JSON data into the []byte
form.
We can use this byte conversion to convert the JSON to a struct. Let’s try an example to convert JSON to a struct using the Unmarshal
method:
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Name string
ID int
Salary int
Position string
}
func main() {
EmployeeJSON := `{"Name": "Sheeraz", "ID": 10, "Salary": 3000, "Position": "Senior Developer" }`
var employees Employee
json.Unmarshal([]byte(EmployeeJSON), &employees)
fmt.Printf("Name: %s, ID: %d , Salary: %d , Position: %s", employees.Name, employees.ID, employees.Salary, employees.Position)
fmt.Println("\n", employees)
}
The code above converts the given JSON to a struct by using the Unmarshal
method of the JSON standard library of GoLang. See the output:
Name: Sheeraz, ID: 10 , Salary: 3000 , Position: Senior Developer
{Sheeraz 10 3000 Senior Developer}
Program exited.
Convert an Array of JSON to Struct in Go
As we can see, the above example converts a single JSON to a struct, but what if we have an array of JSON? Let’s try an example to convert the array of JSON to a struct:
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Name string
ID int
Salary int
Position string
}
func main() {
EmployeeJSON := `[{"Name": "Sheeraz", "ID": 10, "Salary": 3000, "Position": "Senior Developer" },
{"Name": "Jack", "ID": 20, "Salary": 2000, "Position": "Junior Developer" },
{"Name": "John", "ID": 30, "Salary": 1500, "Position": "Junior Developer" },
{"Name": "Mike", "ID": 40, "Salary": 1000, "Position": "Intern" }
]`
var employees []Employee
json.Unmarshal([]byte(EmployeeJSON), &employees)
fmt.Printf("Employees Structs : %+v", employees)
}
The code above will convert the array of JSON to an array of structs. See the output:
Employees Structs : [
{Name:Sheeraz ID:10 Salary:3000 Position:Senior Developer}
{Name:Jack ID:20 Salary:2000 Position:Junior Developer}
{Name:John ID:30 Salary:1500 Position:Junior Developer}
{Name:Mike ID:40 Salary:1000 Position:Intern}]
Program exited.
Convert a Nested JSON to Struct in Go
JSONs are also created in a nested form where a field in JSON contains another field. To convert this type of JSONS, we have to create nested structs.
Let’s see an example:
package main
import (
"encoding/json"
"fmt"
)
type EmployeeType struct {
Gender string
Contract string
}
type Employee struct {
Name string
ID int
Salary int
Position string
EmployeeType EmployeeType
}
func main() {
EmployeeJSON := `{"Name": "Sheeraz", "ID": 10, "Salary": 3000, "Position": "Senior Developer", "EmployeeType": {"Gender": "Male" ,"Contract": "Fulltime"} }`
var employees Employee
json.Unmarshal([]byte(EmployeeJSON), &employees)
fmt.Printf("Name: %s, ID: %d , Salary: %d , Position: %s, Gender: %s, Contract: %s",
employees.Name, employees.ID, employees.Salary, employees.Position, employees.EmployeeType.Gender, employees.EmployeeType.Contract)
fmt.Println("\n", employees)
}
The code above will convert a nested JSON object to a nested struct. See the output:
Name: Sheeraz, ID: 10 , Salary: 3000 , Position: Senior Developer, Gender: Male, Contract: Fulltime
{Sheeraz 10 3000 Senior Developer {Male Fulltime}}
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook