Go에서 JSON을 구조체로 변환
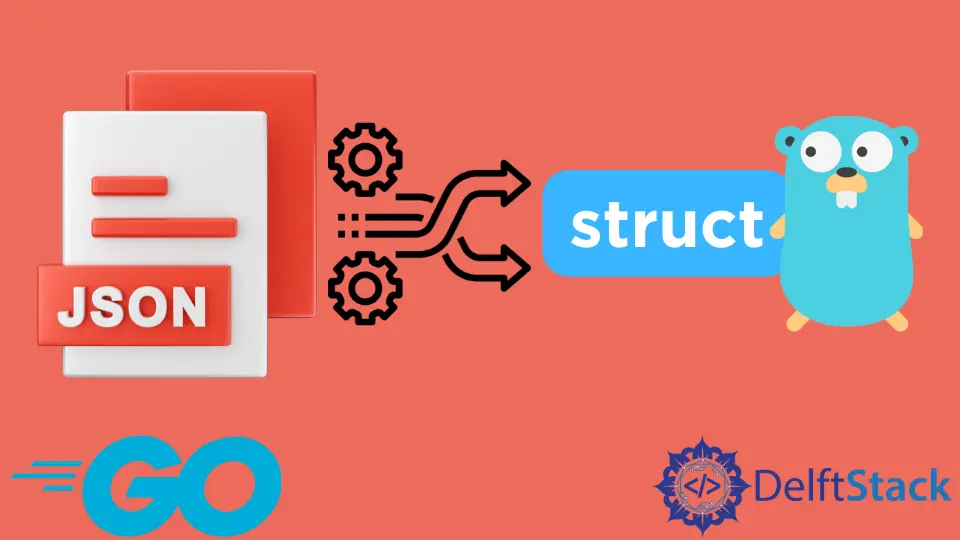
이 튜토리얼은 JSON을 GoLang의 구조체로 변환하는 방법을 보여줍니다.
Unmarshal
메서드를 사용하여 Go에서 JSON을 구조체로 변환
GoLang의 encoding/json
패키지는 JSON 데이터를 바이트 형식으로 변환하는 Unmarshal
기능을 제공합니다. 이 함수는 구조화 및 비구조화 JSON 데이터를 []byte
형식으로 구문 분석할 수 있습니다.
이 바이트 변환을 사용하여 JSON을 구조체로 변환할 수 있습니다. Unmarshal
메서드를 사용하여 JSON을 구조체로 변환하는 예제를 시도해 보겠습니다.
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Name string
ID int
Salary int
Position string
}
func main() {
EmployeeJSON := `{"Name": "Sheeraz", "ID": 10, "Salary": 3000, "Position": "Senior Developer" }`
var employees Employee
json.Unmarshal([]byte(EmployeeJSON), &employees)
fmt.Printf("Name: %s, ID: %d , Salary: %d , Position: %s", employees.Name, employees.ID, employees.Salary, employees.Position)
fmt.Println("\n", employees)
}
위의 코드는 GoLang의 JSON 표준 라이브러리의 Unmarshal
메서드를 사용하여 주어진 JSON을 구조체로 변환합니다. 출력을 참조하십시오.
Name: Sheeraz, ID: 10 , Salary: 3000 , Position: Senior Developer
{Sheeraz 10 3000 Senior Developer}
Program exited.
Go에서 JSON 배열을 구조체로 변환
보시다시피 위의 예는 단일 JSON을 구조체로 변환하지만 JSON 배열이 있으면 어떻게 될까요? JSON 배열을 구조체로 변환하는 예제를 살펴보겠습니다.
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Name string
ID int
Salary int
Position string
}
func main() {
EmployeeJSON := `[{"Name": "Sheeraz", "ID": 10, "Salary": 3000, "Position": "Senior Developer" },
{"Name": "Jack", "ID": 20, "Salary": 2000, "Position": "Junior Developer" },
{"Name": "John", "ID": 30, "Salary": 1500, "Position": "Junior Developer" },
{"Name": "Mike", "ID": 40, "Salary": 1000, "Position": "Intern" }
]`
var employees []Employee
json.Unmarshal([]byte(EmployeeJSON), &employees)
fmt.Printf("Employees Structs : %+v", employees)
}
위의 코드는 JSON 배열을 구조체 배열로 변환합니다. 출력을 참조하십시오.
Employees Structs : [
{Name:Sheeraz ID:10 Salary:3000 Position:Senior Developer}
{Name:Jack ID:20 Salary:2000 Position:Junior Developer}
{Name:John ID:30 Salary:1500 Position:Junior Developer}
{Name:Mike ID:40 Salary:1000 Position:Intern}]
Program exited.
Go에서 중첩된 JSON을 구조체로 변환
JSON은 JSON의 필드에 다른 필드가 포함된 중첩 형식으로도 생성됩니다. 이 유형의 JSONS를 변환하려면 중첩된 구조체를 만들어야 합니다.
예를 보자:
package main
import (
"encoding/json"
"fmt"
)
type EmployeeType struct {
Gender string
Contract string
}
type Employee struct {
Name string
ID int
Salary int
Position string
EmployeeType EmployeeType
}
func main() {
EmployeeJSON := `{"Name": "Sheeraz", "ID": 10, "Salary": 3000, "Position": "Senior Developer", "EmployeeType": {"Gender": "Male" ,"Contract": "Fulltime"} }`
var employees Employee
json.Unmarshal([]byte(EmployeeJSON), &employees)
fmt.Printf("Name: %s, ID: %d , Salary: %d , Position: %s, Gender: %s, Contract: %s",
employees.Name, employees.ID, employees.Salary, employees.Position, employees.EmployeeType.Gender, employees.EmployeeType.Contract)
fmt.Println("\n", employees)
}
위의 코드는 중첩된 JSON 개체를 중첩된 구조체로 변환합니다. 출력을 참조하십시오.
Name: Sheeraz, ID: 10 , Salary: 3000 , Position: Senior Developer, Gender: Male, Contract: Fulltime
{Sheeraz 10 3000 Senior Developer {Male Fulltime}}
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook