Go 中如何在控制台终端中打印结构体变量
Suraj Joshi
2023年12月11日
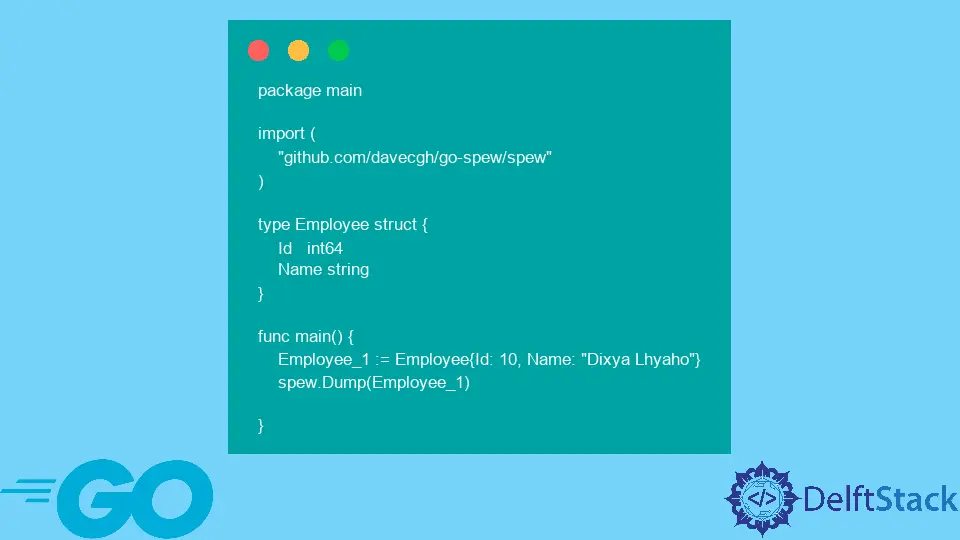
在 Go 中,结构体 struct
是具有相同或不同数据类型的不同字段的集合。结构体类似于面向对象编程范例中的类。我们可以使用软件包 fmt
的 Printf
功能以及特殊标记作为 Printf
功能的参数来打印结构。同样,我们也可以使用特殊的程序包来打印结构,例如 encoding/json
,go-spew
和 Pretty Printer Library
。
在 Go 中声明结构体 struct
Go 中的结构体是使用 struct
关键字创建的。
package main
import "fmt"
type info struct {
Name string
Address string
Pincode int
}
func main() {
a1 := info{"Dikhsya Lhyaho", "Jhapa", 123}
fmt.Println("Info of Dikhsya: ", a1)
}
输出:
Info of Dikhsya: {Dikhsya Lhyaho Jhapa 123}
我们可以在 Go 中使用各种软件包打印 struct
变量。其中一些描述如下:
fmt 包的 Printf 功能
我们可以将软件包 fmt
的 Printf
功能与特殊格式设置一起使用。使用 fmt
显示变量的可用格式选项是:
格式 | 描述 |
---|---|
%v |
以默认格式打印变量值 |
%+v |
用值添加字段名称 |
%#v |
该值的 Go 语法表示形式 |
%T |
值类型的 Go 语法表示形式 |
%% |
文字百分号;不消耗任何价值 |
示例代码:
package main
import "fmt"
type Employee struct {
Id int64
Name string
}
func main() {
Employee_1 := Employee{Id: 10, Name: "Dixya Lhyaho"}
fmt.Printf("%+v\n", Employee_1) // with Variable name
fmt.Printf("%v\n", Employee_1) // Without Variable Name
fmt.Printf("%d\n", Employee_1.Id)
fmt.Printf("%s\n", Employee_1.Name)
}
输出:
{Id:10 Name:Dixya Lhyaho}
{10 Dixya Lhyaho}
10
Dixya Lhyaho
encoding/json
包的 Marshal
函数
另一种方法是使用 encoding/json
包的 Marshal
函数。
package main
import (
"encoding/json"
"fmt"
)
type Employee struct {
Id int64
Name string
}
func main() {
Employee_1 := Employee{Id: 10, Name: "Dixya Lhyaho"}
jsonE, _ := json.Marshal(Employee_1)
fmt.Println(string(jsonE))
}
输出:
{"Id":10,"Name":"Dixya Lhyaho"}
go-spew
软件包的 Dump
函数
另一种方法是使用 go-spew
软件包的 Dump
函数。
。
package main
import (
"github.com/davecgh/go-spew/spew"
)
type Employee struct {
Id int64
Name string
}
func main() {
Employee_1 := Employee{Id: 10, Name: "Dixya Lhyaho"}
spew.Dump(Employee_1)
}
输出:
(main.Employee) {
Id: (int64) 10,
Name: (string) (len=12) "Dixya Lhyaho"
}
要安装 go-spew
软件包,请在终端中运行以下命令:
go get -u github.com/davecgh/go-spew/spew
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn