Go에서 구조체의 문자열 표현 가져오기
Musfirah Waseem
2023년6월20일
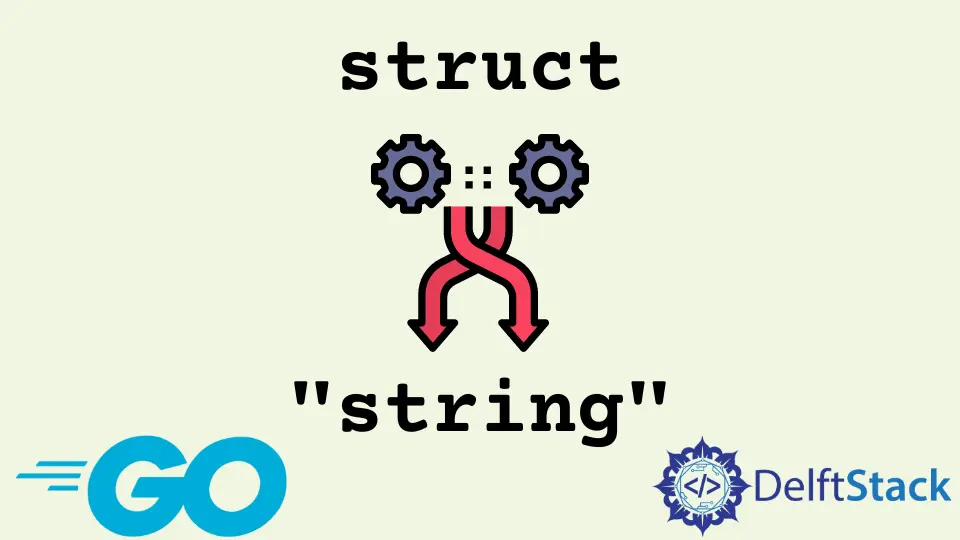
Go를 사용하면 구조에서 데이터를 직렬화하는 여러 가지 간단한 표준 방법을 사용할 수 있습니다.
Go에서 String
메서드를 사용하여 구조체를 문자열로 변환
GoLang 패키지 String
은 UTF-8로 인코딩된 문자열을 조작하고 편집하는 간단한 기능을 구현하는 데 도움이 됩니다.
예제 코드:
package main
import "fmt"
type myStructure struct {
bar string
}
func (f myStructure) String() string {
return fmt.Sprintf("The structure I made has the following data: %s", f.bar)
}
func main() {
fmt.Println(myStructure{"Hello, World! GoLang is fun!"})
}
출력:
The structure I made has the following data: Hello, World! GoLang is fun!
위의 코드에서 우리는 구조체를 문자열로 변환할 수 있는 myStructure
라는 명명된 구조체에 String()
함수를 첨부했습니다.
json.Marshal
메서드를 사용하여 Go에서 구조체를 JSON으로 변환
GoLang encoding/json
패키지에는 JSON으로 또는 JSON에서 변환하는 데 사용할 수 있는 유틸리티가 있습니다. json.Marshal
메서드는 구조체를 JSON으로 변환할 수 있습니다.
예제 코드:
package main
import (
"encoding/json"
"fmt"
)
func main() {
type MyStructure struct {
Message string `json:"From Structure"`
}
val := &MyStructure{
Message: "Hello, World!",
}
// convert struct to json string
jsonBytes, err := json.Marshal(val)
fmt.Println(string(jsonBytes), err)
}
출력:
{"From Structure":"Hello, World!"} <nil>
위의 방법을 사용하는 동안 정의된 구조체의 내보낸 필드만 외부 라이브러리에서 사용할 수 있습니다. 따라서 구조체의 내보내기 필드만 변환된 JSON 문자열에 복사됩니다.
작가: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn