Constant Array in Go
- Constant Arrays in Go
-
Use the
[...]
Operator for Fixed-Size Arrays -
Use the
iota
Identifier to Create a Constant Array-Like Structure - Conclusion
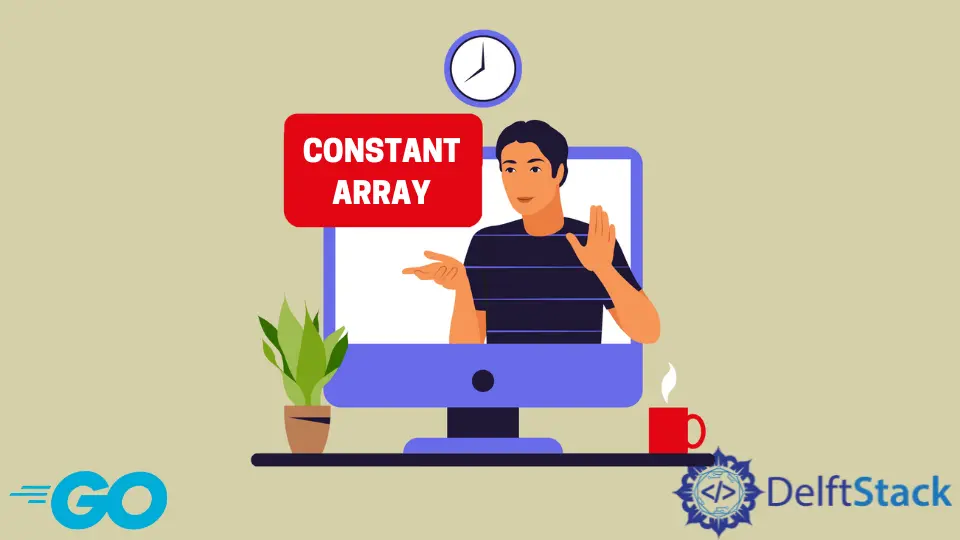
Go developers often prefer using global constants over global variables for setting up their code. However, a common challenge arises when attempting to create constant versions of more complex data types like arrays, maps, or slices.
In Go, constants are indeed constant, and they are generated at compile time. These constants can only be of certain types, including integers, characters (runes), strings, or Booleans.
This limitation stems from the fact that constant expressions must be known at compile time to be evaluated by the Go compiler.
The inability to create constant arrays, maps, or slices directly poses a limitation to developers. However, there are alternative approaches to work with constant-like structures in Go, such as using fixed-size arrays and ensuring that the values within them remain constant.
Constant Arrays in Go
Go does not support constant arrays and slices because, as mentioned earlier, constant values in Go are determined at build time. At runtime, arrays or slices are dynamic and, therefore, cannot be constants.
If you try to create a constant array in Go, you’ll encounter a compilation error. Here’s an example:
package main
import "fmt"
func main() {
const a = [1]int{1}
fmt.Println(a)
}
When you attempt to compile this code, you will receive the following error message:
const initializer [1]int literal is not a constant
This error highlights the fact that you cannot create a constant array in Go.
Use the [...]
Operator for Fixed-Size Arrays
While you cannot create constant arrays in Go, you can work with fixed-size arrays using the [...]
operator.
This operator ensures that you obtain an array with a fixed size, although the values within the array can change. Here’s an example of declaring and working with a fixed-size array in Go:
package main
import "fmt"
func main() {
a := [...]int{1, 2, 3, 4, 5, 6, 7, 8, 9}
fmt.Println(a)
}
Output:
[1 2 3 4 5 6 7 8 9]
In this code, a
is declared as a fixed-size array with the [...]
operator, and it contains the values 1, 2, 3, 4, 5, 6, 7, 8, 9
. The [...]
operator ensures that the size of the array is fixed at compile time.
While the values in the array can change during runtime, the size remains constant. This approach allows you to work with array-like structures in a way that is suitable for your needs.
Use the iota
Identifier to Create a Constant Array-Like Structure
In Go, the iota
identifier is a special constant generator that is often used in conjunction with the const
keyword to create sequences of values within a constant block. It’s commonly employed when you need to create a set of related constants, such as an array of values.
While iota
itself is not used to directly create arrays, you can use it to generate a sequence of constant values that simulate the behavior of a constant array with consecutive elements.
Let’s explore how you can use iota
to create a constant array-like structure in Go:
package main
import "fmt"
const (
Monday = iota // 0
Tuesday // 1
Wednesday // 2
Thursday // 3
Friday // 4
Saturday // 5
Sunday // 6
)
func main() {
daysOfWeek := [...]int{Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday}
fmt.Println(daysOfWeek)
}
Output:
[0 1 2 3 4 5 6]
In this example, we define constants for the days of the week using iota
. iota
starts with the value 0
for the first constant (Monday
) and increments by 1
for each subsequent constant.
Then, we create a fixed-size array, daysOfWeek
, and populate it with the constants. This array essentially behaves as a constant array with days of the week.
By using iota
in conjunction with const
, you create a sequence of constants cleanly and efficiently. This technique is especially useful when you need to define a set of related, ordered values within an array-like structure in your Go code.
Keep in mind that while the values themselves are constants, the resulting array can still be modified, as Go does not support truly constant arrays. However, by convention and through discipline, you can treat the array as if it were constant, avoiding modifications in your code.
Conclusion
In summary, creating constant arrays in Go is not possible due to the nature of constant values being determined at compile time. However, you can use the [...]
operator to work with fixed-size arrays, which can provide the desired level of constancy for your specific use case.
By understanding these principles, Go developers can effectively manage complex data types and structures in their code.