How to Reverse an Array in Go
Jay Singh
Feb 02, 2024
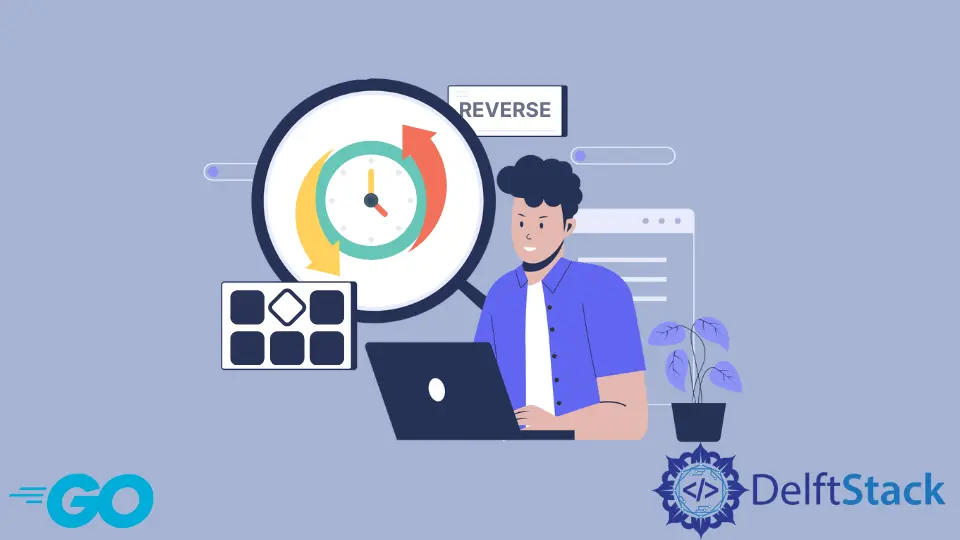
This tutorial will demonstrate how to reverse an array in Go.
Reverse an Array Using the for
Loop in Go
Due to the many return values in Go, reversing an array is quite simple. We can loop through the first half of the array, switching each element with its mirrored counterpart one at a time.
We will create a function that takes an array as input in this example. We will iterate through the input array, swap the beginning and last elements of the supplied array, and then return the array.
Example 1:
package main
import "fmt"
func reverseArray(arr []int) []int {
for i, j := 0, len(arr)-1; i < j; i, j = i+1, j-1 {
arr[i], arr[j] = arr[j], arr[i]
}
return arr
}
func main() {
fmt.Println(reverseArray([]int{100, 200, 300, 400, 500}))
}
Output:
[500 400 300 200 100]
Example 2:
package main
import "fmt"
func reverse(numbers []int) []int {
for i := 0; i < len(numbers)/2; i++ {
j := len(numbers) - i - 1
numbers[i], numbers[j] = numbers[j], numbers[i]
}
return numbers
}
func main() {
fmt.Printf("%v\n", reverse([]int{100, 200, 300, 400, 500}))
}
Output:
[500 400 300 200 100]