Golang-Kopie-Slice
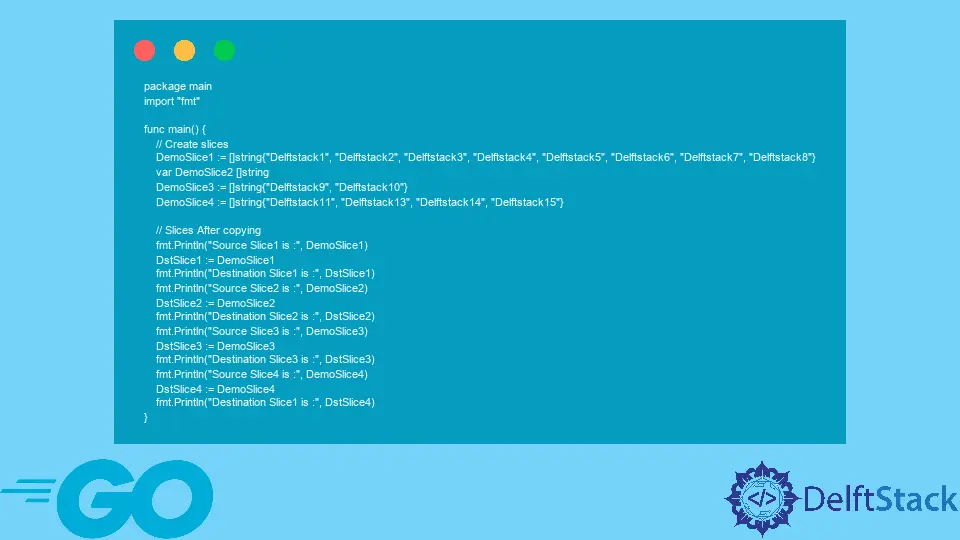
Dieses Tutorial zeigt, wie Sie ein Slice in GoLang kopieren.
Slice in GoLang kopieren
Das Kopieren eines Slice in GoLang kann durch verschiedene Methoden erreicht werden. Zu diesem Zweck werden normalerweise die Methoden copy()
und append()
verwendet, wobei die Methode copy()
die tiefe Kopie eines bestimmten Slices erhält und die Methode append()
den Inhalt von a kopiert in eine leere Scheibe schneiden.
Verwenden Sie die Copy()
-Methode, um ein Slice in Go zu kopieren
Die copy()
-Methode gilt als die beste Methode, um ein Slice im Golang zu kopieren, da sie eine tiefe Kopie des Slice erstellt.
Syntax:
func copy(destination, source []Type) int
Das Ziel ist das kopierte Slice und die Quelle ist das Slice, von dem wir kopieren. Die Funktion gibt die Anzahl der Elemente des kopierten Slice zurück.
Codebeispiel:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
DstSlice1 := make([]string, len(DemoSlice1))
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := copy(DstSlice1, DemoSlice1)
fmt.Println("\n Destination Slice 1 after copying:", DstSlice1)
fmt.Println("Total number of elements copied:", CopiedSlice1)
CopiedSlice2 := copy(DstSlice2, DemoSlice2)
fmt.Println("\n Destination Slice 2 after copying:", DstSlice2)
fmt.Println("Total number of elements copied:", CopiedSlice2)
CopiedSlice3 := copy(DstSlice3, DemoSlice3)
fmt.Println("\n Destination Slice 3 after copying:", DstSlice3)
fmt.Println("Total number of elements copied:", CopiedSlice3)
CopiedSlice4 := copy(DstSlice4, DemoSlice4)
fmt.Println("\n Destination Slice 4 after copying:", DstSlice4)
fmt.Println("Total number of elements copied:", CopiedSlice4)
}
Der obige Code erstellt vier Quell-Slices und vier Ziel-Slices mit unterschiedlichen Membern oder leer mit derselben Länge.
Ausgang:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [ ]
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
Destination Slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Total number of elements copied: 8
Destination Slice 2 after copying: [Tutorials1]
Total number of elements copied: 0
Destination Slice 3 after copying: [Delftstack9 Delftstack10]
Total number of elements copied: 2
Destination Slice 4 after copying: [Delftstack11 Delftstack13 Delftstack14]
Total number of elements copied: 3
Program exited.
Verwenden Sie die Append()
-Methode, um ein Slice in Go zu kopieren
Die Append-Methode hängt den Inhalt eines bestimmten Slice an das Ziel-Slice an.
Syntax:
func destination = append(destination, source...)
Die Append-Methode kopiert den Inhalt des Quell-Slice in einen leeren Ziel-Slice oder einen Slice mit Mitgliedern. Es wird das Segment mit vorherigen und kopierten Elementen zurückgeben, was sich von der Kopiermethode unterscheidet.
Codebeispiel:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DstSlice1 []string
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := append(DstSlice1, DemoSlice1...)
fmt.Println("The copied slice 1 after copying: ", CopiedSlice1)
CopiedSlice2 := append(DstSlice2, DemoSlice2...)
fmt.Println("The copied slice 2 after copying: ", CopiedSlice2)
CopiedSlice3 := append(DstSlice3, DemoSlice3...)
fmt.Println("The copies slice 3 after copying: ", CopiedSlice3)
CopiedSlice4 := append(DstSlice4, DemoSlice4...)
fmt.Println("The copied slice 4 after copying: ", CopiedSlice4)
}
Das obige Code-Snippet kopiert den Inhalt eines Slice in ein anderes.
Ausgang:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : []
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
The copied slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
The copied slice 2 after copying: [Tutorials1]
The copies slice 3 after copying: [Tutorials1 Tutorials2 Delftstack9 Delftstack10]
The copied slice 4 after copying: [Tutorials1 Tutorials2 Tutorials3 Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited
Verwenden Sie die Zuweisungsmethode, um ein Slice in Go zu kopieren
Die Zuweisungsmethode Kopie ist eine flache Kopie eines Slice, bei der wir dem Ziel-Slice ein Quell-Slice zuweisen.
Codebeispiel:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DemoSlice2 []string
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
// Slices After copying
fmt.Println("Source Slice1 is :", DemoSlice1)
DstSlice1 := DemoSlice1
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
DstSlice2 := DemoSlice2
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
DstSlice3 := DemoSlice3
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
DstSlice4 := DemoSlice4
fmt.Println("Destination Slice1 is :", DstSlice4)
}
Wie wir sehen können, weisen wir die Slices anderen Slices zu. Dies ist eine oberflächliche Methode und wird nicht häufig verwendet, da sich das ursprüngliche Slice ebenfalls ändert, wenn wir den Inhalt der Kopie ändern.
Ausgang:
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Source Slice2 is : []
Destination Slice2 is : []
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Delftstack9 Delftstack10]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook