Rebanada de copia de Golang
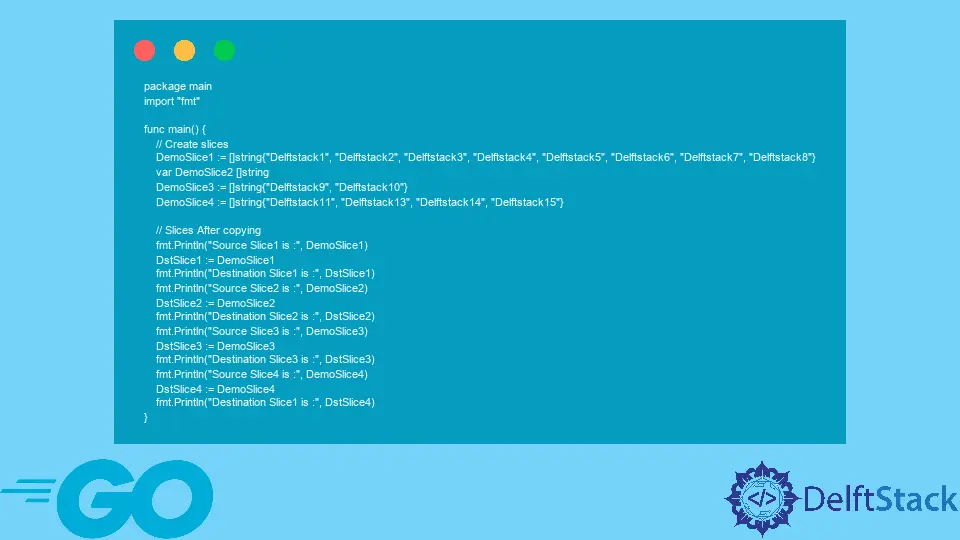
Este tutorial demuestra cómo copiar un segmento en GoLang.
Copiar segmento en GoLang
La copia de un segmento en GoLang se puede lograr a través de diferentes métodos. Los métodos copy()
y append()
suelen utilizarse para este propósito, donde copy()
obtiene la copia en profundidad de un segmento dado, y el método append()
copiará el contenido de un segmento. corte en una rebanada vacía.
Use el método Copiar ()
para copiar una rebanada en Go
El método copy()
se considera el mejor método para copiar un segmento en Golang porque crea una copia profunda del segmento.
Sintaxis:
func copy(destination, source []Type) int
El destino es el segmento copiado y el origen es el segmento desde el que copiamos. La función devuelve el número de elementos del segmento copiado.
Ejemplo de código:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
DstSlice1 := make([]string, len(DemoSlice1))
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := copy(DstSlice1, DemoSlice1)
fmt.Println("\n Destination Slice 1 after copying:", DstSlice1)
fmt.Println("Total number of elements copied:", CopiedSlice1)
CopiedSlice2 := copy(DstSlice2, DemoSlice2)
fmt.Println("\n Destination Slice 2 after copying:", DstSlice2)
fmt.Println("Total number of elements copied:", CopiedSlice2)
CopiedSlice3 := copy(DstSlice3, DemoSlice3)
fmt.Println("\n Destination Slice 3 after copying:", DstSlice3)
fmt.Println("Total number of elements copied:", CopiedSlice3)
CopiedSlice4 := copy(DstSlice4, DemoSlice4)
fmt.Println("\n Destination Slice 4 after copying:", DstSlice4)
fmt.Println("Total number of elements copied:", CopiedSlice4)
}
El código anterior crea cuatro segmentos de origen y cuatro segmentos de destino con diferentes miembros o vacíos con la misma longitud.
Producción :
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [ ]
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
Destination Slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Total number of elements copied: 8
Destination Slice 2 after copying: [Tutorials1]
Total number of elements copied: 0
Destination Slice 3 after copying: [Delftstack9 Delftstack10]
Total number of elements copied: 2
Destination Slice 4 after copying: [Delftstack11 Delftstack13 Delftstack14]
Total number of elements copied: 3
Program exited.
Utilice el método Append()
para copiar un segmento en Go
El método append agregará el contenido de un segmento dado al segmento de destino.
Sintaxis:
func destination = append(destination, source...)
El método append copiará el contenido del segmento de origen en un segmento de destino vacío o en un segmento con miembros. Devolverá el segmento con miembros anteriores y copiados, que es diferente del método de copia.
Ejemplo de código:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DstSlice1 []string
var DemoSlice2 []string
DstSlice2 := []string{"Tutorials1"}
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DstSlice3 := []string{"Tutorials1", "Tutorials2"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
DstSlice4 := []string{"Tutorials1", "Tutorials2", "Tutorials3"}
// Slices Before copying
fmt.Println("Source Slice1 is :", DemoSlice1)
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
fmt.Println("Destination Slice1 is :", DstSlice4)
// Copy the slices
CopiedSlice1 := append(DstSlice1, DemoSlice1...)
fmt.Println("The copied slice 1 after copying: ", CopiedSlice1)
CopiedSlice2 := append(DstSlice2, DemoSlice2...)
fmt.Println("The copied slice 2 after copying: ", CopiedSlice2)
CopiedSlice3 := append(DstSlice3, DemoSlice3...)
fmt.Println("The copies slice 3 after copying: ", CopiedSlice3)
CopiedSlice4 := append(DstSlice4, DemoSlice4...)
fmt.Println("The copied slice 4 after copying: ", CopiedSlice4)
}
El fragmento de código anterior copiará el contenido de un segmento a otro.
Producción :
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : []
Source Slice2 is : []
Destination Slice2 is : [Tutorials1]
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Tutorials1 Tutorials2]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Tutorials1 Tutorials2 Tutorials3]
The copied slice 1 after copying: [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
The copied slice 2 after copying: [Tutorials1]
The copies slice 3 after copying: [Tutorials1 Tutorials2 Delftstack9 Delftstack10]
The copied slice 4 after copying: [Tutorials1 Tutorials2 Tutorials3 Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited
Utilice el método de asignación para copiar un sector en Go
La copia del método de asignación es una copia superficial de un segmento donde asignamos un segmento de origen al segmento de destino.
Ejemplo de código:
package main
import "fmt"
func main() {
// Create slices
DemoSlice1 := []string{"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6", "Delftstack7", "Delftstack8"}
var DemoSlice2 []string
DemoSlice3 := []string{"Delftstack9", "Delftstack10"}
DemoSlice4 := []string{"Delftstack11", "Delftstack13", "Delftstack14", "Delftstack15"}
// Slices After copying
fmt.Println("Source Slice1 is :", DemoSlice1)
DstSlice1 := DemoSlice1
fmt.Println("Destination Slice1 is :", DstSlice1)
fmt.Println("Source Slice2 is :", DemoSlice2)
DstSlice2 := DemoSlice2
fmt.Println("Destination Slice2 is :", DstSlice2)
fmt.Println("Source Slice3 is :", DemoSlice3)
DstSlice3 := DemoSlice3
fmt.Println("Destination Slice3 is :", DstSlice3)
fmt.Println("Source Slice4 is :", DemoSlice4)
DstSlice4 := DemoSlice4
fmt.Println("Destination Slice1 is :", DstSlice4)
}
Como podemos ver, asignamos los cortes a otros cortes. Este es un método superficial y no muy utilizado porque cuando modificamos el contenido de la copia, la porción original también cambiará.
Producción :
Source Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Destination Slice1 is : [Delftstack1 Delftstack2 Delftstack3 Delftstack4 Delftstack5 Delftstack6 Delftstack7 Delftstack8]
Source Slice2 is : []
Destination Slice2 is : []
Source Slice3 is : [Delftstack9 Delftstack10]
Destination Slice3 is : [Delftstack9 Delftstack10]
Source Slice4 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Destination Slice1 is : [Delftstack11 Delftstack13 Delftstack14 Delftstack15]
Program exited.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook