How to Check if a File Exists or Not in Go
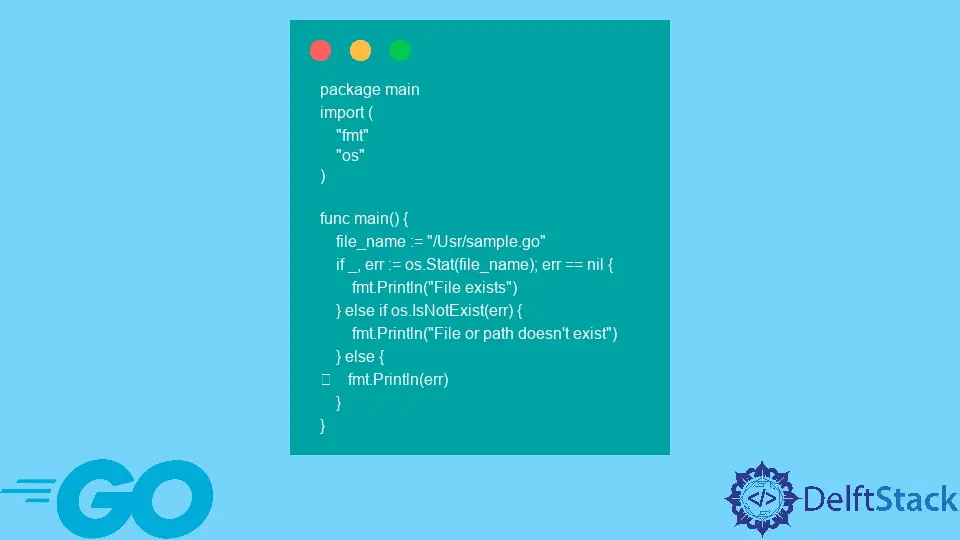
In the world of programming, checking whether a file exists is a fundamental task that can save you from unexpected errors and improve your application’s robustness. Whether you’re building a web application, a command-line tool, or any software that interacts with the file system, knowing how to verify the existence of a file in Go is essential.
In this tutorial, we will walk you through the steps to check if a file exists or not using Go. You’ll learn different methods to accomplish this, complete with clear code examples and explanations. By the end of this article, you will have a solid understanding of file handling in Go, ensuring your applications run smoothly and efficiently.
Method 1: Using os.Stat
The first method to check if a file exists in Go is by using the os.Stat
function. This function retrieves the file information and returns an error if the file does not exist. It’s a straightforward and effective way to check for file existence.
Here’s how you can use os.Stat
:
package main
import (
"fmt"
"os"
)
func main() {
filename := "example.txt"
_, err := os.Stat(filename)
if os.IsNotExist(err) {
fmt.Println("File does not exist.")
} else {
fmt.Println("File exists.")
}
}
Output:
File exists.
In this code snippet, we first import the necessary packages: fmt
for printing messages and os
for file operations. We define the filename we want to check. The os.Stat
function is called with the filename, which returns two values: the file information and an error. We then use os.IsNotExist(err)
to check if the error indicates that the file does not exist. If it does, we print a message saying the file does not exist. If there’s no error, we confirm that the file exists. This method is efficient and leverages Go’s built-in error handling mechanisms.
Method 2: Using os.Open
Another method to check if a file exists is by attempting to open the file using os.Open
. If the file does not exist, the operation will return an error, which we can use to determine the file’s existence.
Here’s an example using os.Open
:
package main
import (
"fmt"
"os"
)
func main() {
filename := "example.txt"
file, err := os.Open(filename)
if err != nil {
fmt.Println("File does not exist.")
return
}
defer file.Close()
fmt.Println("File exists.")
}
Output:
File exists.
In this example, we again import the necessary packages and define the filename. We attempt to open the file with os.Open
. If the file does not exist or cannot be opened for any reason, an error will be returned. We check this error and print a corresponding message. If there’s no error, we use defer file.Close()
to ensure the file is closed properly after we’re done with it. This method is particularly useful when you also need to read from the file immediately after checking its existence.
Method 3: Using ioutil.ReadFile
A third approach is to use ioutil.ReadFile
, which reads the entire file into memory. This method can also be utilized to check for file existence, although it’s typically used for reading file contents.
Here’s how you can implement this:
package main
import (
"fmt"
"io/ioutil"
)
func main() {
filename := "example.txt"
_, err := ioutil.ReadFile(filename)
if err != nil {
fmt.Println("File does not exist.")
return
}
fmt.Println("File exists.")
}
Output:
File exists.
In this code snippet, we import fmt
for output and io/ioutil
for file reading. We attempt to read the file using ioutil.ReadFile
, which returns the file contents and an error. If the file does not exist, the error is captured, and we print a message indicating that the file does not exist. If the operation is successful, we print that the file exists. While this method is effective, it’s worth noting that it reads the entire file into memory, which may not be ideal for large files.
Conclusion
Checking if a file exists in Go is a critical operation that can help you build more reliable applications. Throughout this tutorial, we’ve explored three different methods: using os.Stat
, os.Open
, and ioutil.ReadFile
. Each method has its advantages, depending on your specific needs. Whether you prefer the simplicity of os.Stat
, the direct approach of os.Open
, or the reading capabilities of ioutil.ReadFile
, Go provides robust tools for file handling. By mastering these techniques, you can ensure that your applications handle files efficiently and gracefully.
FAQ
-
How do I check if a directory exists in Go?
You can use the same methods mentioned in this article, likeos.Stat
, but ensure to check if the path is a directory using theIsDir()
method on the returned file information. -
Can I check for file existence in a different directory?
Yes, you can specify the full path of the file you want to check in the methods discussed.
-
Is there a performance difference between these methods?
Yes,os.Stat
is generally faster for merely checking existence, whileioutil.ReadFile
reads the entire file into memory, which can be slower, especially for large files. -
What should I do if I need to create the file if it doesn’t exist?
You can useos.OpenFile
with the appropriate flags to create the file if it doesn’t exist. -
Are there any third-party libraries for file handling in Go?
Yes, there are several libraries available, such asafero
, which provide more advanced file system operations.