Vérifier si un fichier existe ou non dans Go
Jay Singh
23 aout 2022
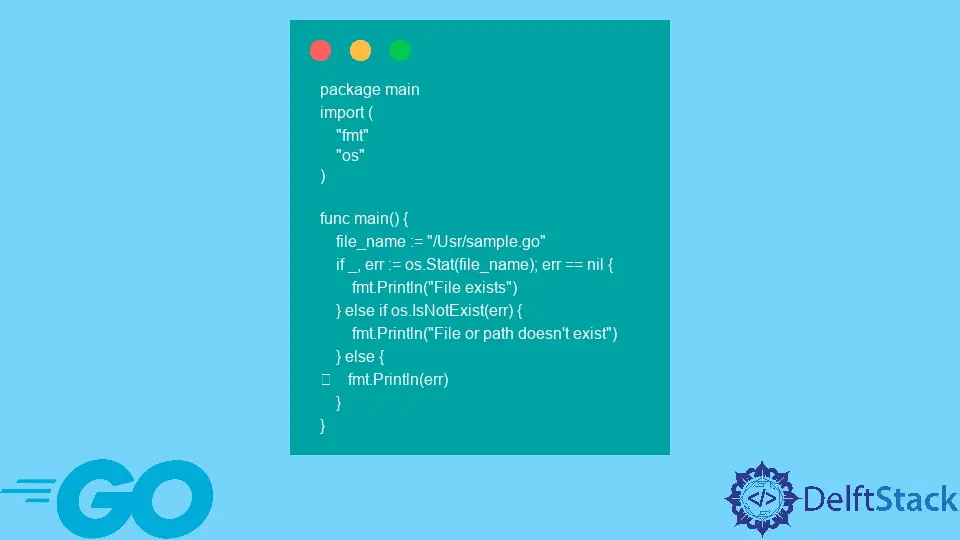
Cet article discutera de la vérification si un fichier existe ou non dans Go.
Utilisez IsNotExist()
et Stat()
pour vérifier si le fichier existe ou non dans Go
Nous utilisons les méthodes IsNotExist()
et Stat()
du package os
du langage de programmation Go pour déterminer si un fichier existe.
La fonction Stat()
retourne un objet qui contient des informations sur un fichier. Si le fichier n’existe pas, il produit un objet d’erreur.
Vous trouverez ci-dessous des exemples de code utilisant IsNotExist()
et Stat()
.
Exemple 1:
package main
import (
"fmt"
"os"
)
// function to check if file exists
func doesFileExist(fileName string) {
_, error := os.Stat(fileName)
// check if error is "file not exists"
if os.IsNotExist(error) {
fmt.Printf("%v file does not exist\n", fileName)
} else {
fmt.Printf("%v file exist\n", fileName)
}
}
func main() {
// check if demo.txt exists
doesFileExist("demo.txt")
// check if demo.csv exists
doesFileExist("demo.csv")
}
Production:
demo.txt file exist
demo.csv file does not exist
Exemple 2 :
package main
import (
"fmt"
"os"
)
func main() {
file_name := "/Usr/sample.go"
if _, err := os.Stat(file_name); err == nil {
fmt.Println("File exists")
} else if os.IsNotExist(err) {
fmt.Println("File or path doesn't exist")
} else {
fmt.Println(err)
}
}
Production:
File or path doesn't exist