在 Go 中检查文件是否存在
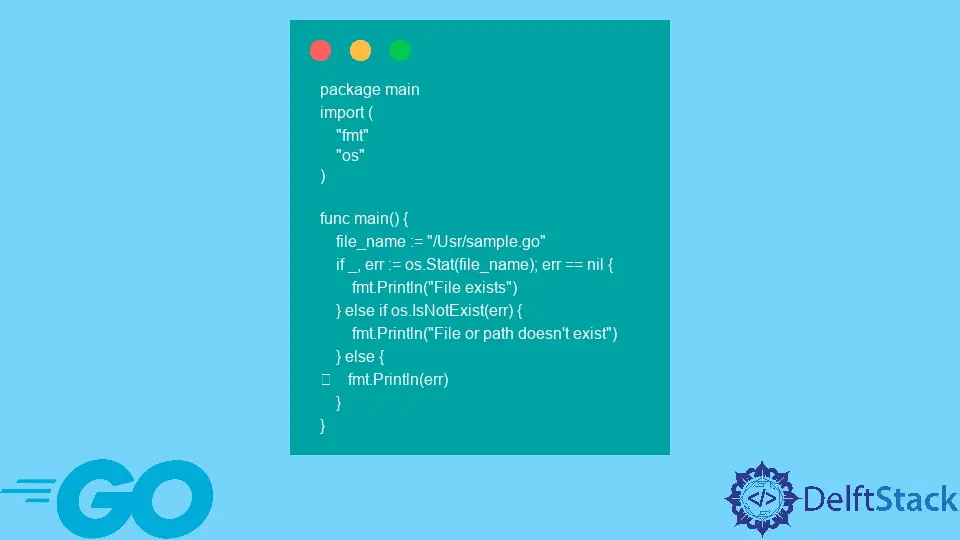
本文将讨论在 Go 中检查文件是否存在。
在 Go 中使用 IsNotExist()
和 Stat()
检查文件是否存在
我们使用 Go 编程语言中 os
包中的 IsNotExist()
和 Stat()
方法来确定文件是否存在。
Stat()
函数返回一个包含文件信息的对象。如果文件不存在,它会产生一个错误对象。
下面是使用 IsNotExist()
和 Stat()
的代码示例。
示例 1:
package main
import (
"fmt"
"os"
)
// function to check if file exists
func doesFileExist(fileName string) {
_, error := os.Stat(fileName)
// check if error is "file not exists"
if os.IsNotExist(error) {
fmt.Printf("%v file does not exist\n", fileName)
} else {
fmt.Printf("%v file exist\n", fileName)
}
}
func main() {
// check if demo.txt exists
doesFileExist("demo.txt")
// check if demo.csv exists
doesFileExist("demo.csv")
}
输出:
demo.txt file exist
demo.csv file does not exist
示例 2:
package main
import (
"fmt"
"os"
)
func main() {
file_name := "/Usr/sample.go"
if _, err := os.Stat(file_name); err == nil {
fmt.Println("File exists")
} else if os.IsNotExist(err) {
fmt.Println("File or path doesn't exist")
} else {
fmt.Println(err)
}
}
输出:
File or path doesn't exist
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe