在 Go 中读取/写入文件
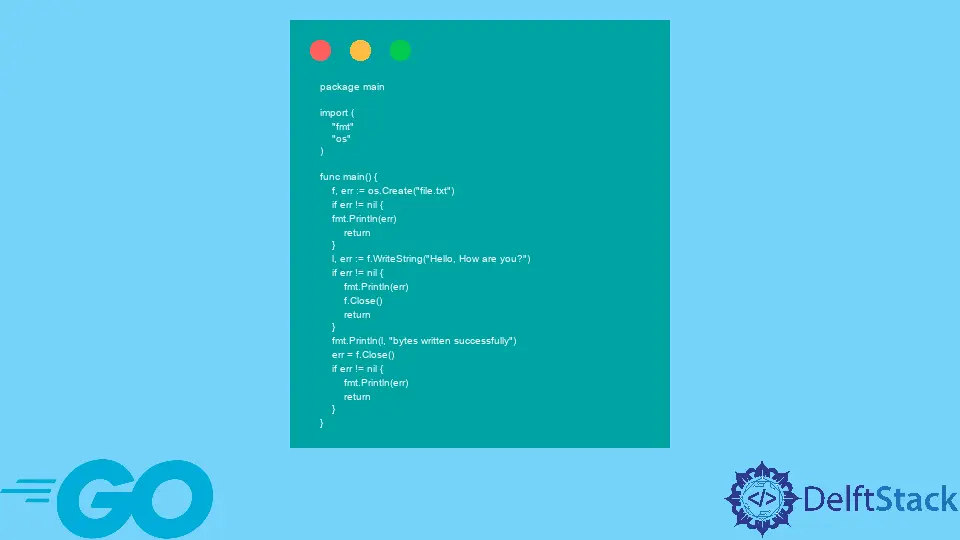
数据可以保存在文件中,从而赋予其结构并允许其永久或临时保存,具体取决于存储介质。
以编程方式处理文件包括获取元数据、创建新文件以及将数据从文件格式读取和写入程序的内部数据结构。
Go 的内置库为文件相关活动提供了出色的支持,例如创建、读/写、重命名、移动和复制文件,以及收集其元数据信息等。
本教程将演示如何在 Go 中读取/写入文件。
在 Go 中使用 WriteString
写入文件
在此示例中,文件写入操作涉及将字符串写入文件。这是一个容易遵循的过程。
以下代码将在程序目录中生成一个名为 file.txt
的文件。然后,如果你用任何文本编辑器打开该文件,就可以在文件中找到 Hello, How are you?
的文字。
让我们看看下面的源代码。
package main
import (
"fmt"
"os"
)
func main() {
f, err := os.Create("file.txt")
if err != nil {
fmt.Println(err)
return
}
l, err := f.WriteString("Hello, How are you?")
if err != nil {
fmt.Println(err)
f.Close()
return
}
fmt.Println(l, "bytes written successfully")
err = f.Close()
if err != nil {
fmt.Println(err)
return
}
}
输出:
19 bytes written successfully
在 Go 中使用 io/ioutil
读取/写入文件
WriteString
方法写入文件,而 ReadFile
函数从下面程序中的文本文件中读取内容。如果 file.txt
不存在,应用程序将创建它,如果存在则截断它。
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
)
func CreateFile() {
file, err := os.Create("file.txt")
if err != nil {
log.Fatalf("failed creating file: %s", err)
}
defer file.Close()
len, err := file.WriteString("Hello, How are you?.")
if err != nil {
log.Fatalf("failed writing to file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len)
fmt.Printf("\nFile Name: %s", file.Name())
}
func ReadFile() {
data, err := ioutil.ReadFile("file.txt")
if err != nil {
log.Panicf("failed reading data from file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len(data))
fmt.Printf("\nData: %s", data)
fmt.Printf("\nError: %v", err)
}
func main() {
fmt.Printf("//// Create a file and Write the content ////\n")
CreateFile()
fmt.Printf("\n\n //// Read file //// \n")
ReadFile()
}
输出:
//// Create a file and Write the content ////
Length: 20 bytes
File Name: file.txt
//// Read file ////
Length: 20 bytes
Data: Hello, How are you?.
Error: <nil>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe