Go에서 파일 읽기/쓰기
Jay Singh
2023년10월8일
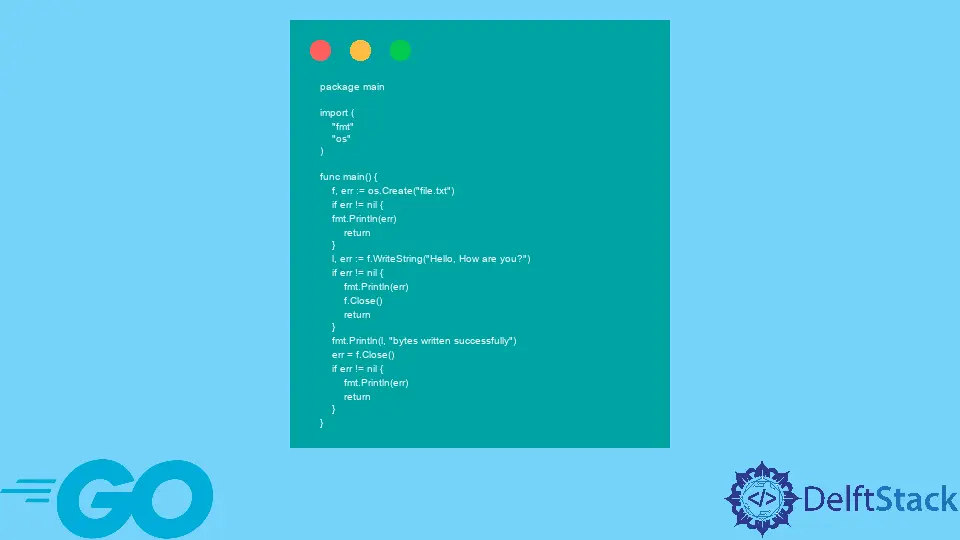
데이터는 파일에 저장할 수 있어 구조를 부여하고 저장 매체에 따라 영구적으로 또는 일시적으로 보관할 수 있습니다.
프로그래밍 방식으로 파일 작업에는 메타데이터 가져오기, 새 파일 만들기, 파일 형식에서 프로그램 내부 데이터 구조로 데이터 읽기 및 쓰기가 포함됩니다.
Go의 내장 라이브러리는 무엇보다도 파일 생성, 읽기/쓰기, 이름 바꾸기, 이동, 복사, 메타데이터 정보 수집과 같은 파일 관련 활동에 대한 탁월한 지원을 제공합니다.
이 자습서는 Go에서 파일을 읽고 쓰는 방법을 보여줍니다.
Go에서 WriteString
을 사용하여 파일에 쓰기
이 예에서 파일 쓰기 작업에는 파일에 문자열 쓰기가 포함됩니다. 이것은 따라하기 쉬운 절차입니다.
다음 코드는 프로그램 디렉토리에 file.txt
라는 파일을 생성합니다. 그런 다음, 이 파일을 텍스트 편집기로 열면 Hello, How are you?
텍스트를 찾을 수 있습니다.
아래의 소스 코드를 살펴보자.
package main
import (
"fmt"
"os"
)
func main() {
f, err := os.Create("file.txt")
if err != nil {
fmt.Println(err)
return
}
l, err := f.WriteString("Hello, How are you?")
if err != nil {
fmt.Println(err)
f.Close()
return
}
fmt.Println(l, "bytes written successfully")
err = f.Close()
if err != nil {
fmt.Println(err)
return
}
}
출력:
19 bytes written successfully
Go에서 io/ioutil
을 사용하여 파일에서 읽기/쓰기
WriteString
메서드는 파일에 쓰는 반면 ReadFile
기능은 아래 프로그램의 텍스트 파일에서 내용을 읽습니다. file.txt
가 존재하지 않는 경우 애플리케이션은 이를 생성하거나 존재하는 경우 이를 자릅니다.
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
)
func CreateFile() {
file, err := os.Create("file.txt")
if err != nil {
log.Fatalf("failed creating file: %s", err)
}
defer file.Close()
len, err := file.WriteString("Hello, How are you?.")
if err != nil {
log.Fatalf("failed writing to file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len)
fmt.Printf("\nFile Name: %s", file.Name())
}
func ReadFile() {
data, err := ioutil.ReadFile("file.txt")
if err != nil {
log.Panicf("failed reading data from file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len(data))
fmt.Printf("\nData: %s", data)
fmt.Printf("\nError: %v", err)
}
func main() {
fmt.Printf("//// Create a file and Write the content ////\n")
CreateFile()
fmt.Printf("\n\n //// Read file //// \n")
ReadFile()
}
출력:
//// Create a file and Write the content ////
Length: 20 bytes
File Name: file.txt
//// Read file ////
Length: 20 bytes
Data: Hello, How are you?.
Error: <nil>