在 Go 中讀取/寫入檔案
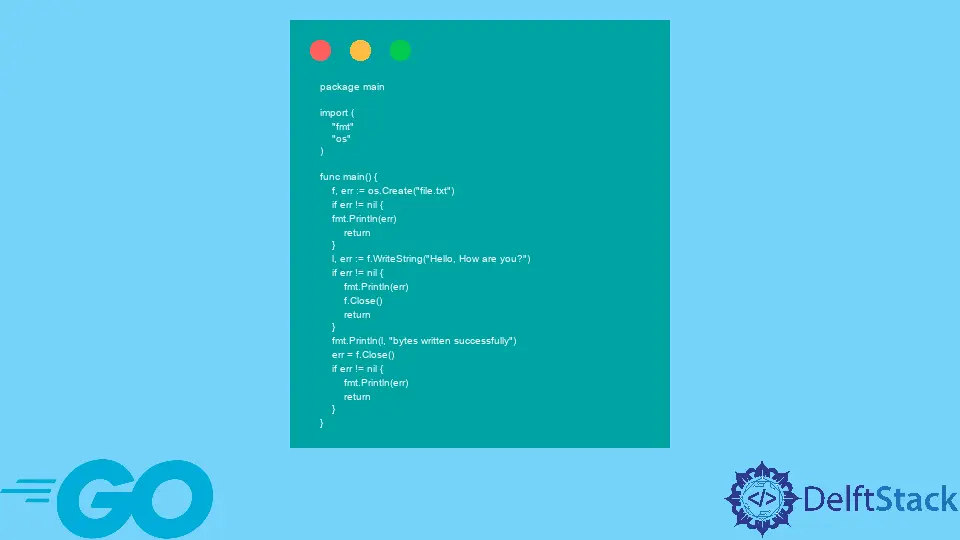
資料可以儲存在檔案中,從而賦予其結構並允許其永久或臨時儲存,具體取決於儲存介質。
以程式設計方式處理檔案包括獲取後設資料、建立新檔案以及將資料從檔案格式讀取和寫入程式的內部資料結構。
Go 的內建庫為檔案相關活動提供了出色的支援,例如建立、讀/寫、重新命名、移動和複製檔案,以及收集其後設資料資訊等。
本教程將演示如何在 Go 中讀取/寫入檔案。
在 Go 中使用 WriteString
寫入檔案
在此示例中,檔案寫入操作涉及將字串寫入檔案。這是一個容易遵循的過程。
以下程式碼將在程式目錄中生成一個名為 file.txt
的檔案。然後,如果你用任何文字編輯器開啟該檔案,就可以在檔案中找到 Hello, How are you?
的文字。
讓我們看看下面的原始碼。
package main
import (
"fmt"
"os"
)
func main() {
f, err := os.Create("file.txt")
if err != nil {
fmt.Println(err)
return
}
l, err := f.WriteString("Hello, How are you?")
if err != nil {
fmt.Println(err)
f.Close()
return
}
fmt.Println(l, "bytes written successfully")
err = f.Close()
if err != nil {
fmt.Println(err)
return
}
}
輸出:
19 bytes written successfully
在 Go 中使用 io/ioutil
讀取/寫入檔案
WriteString
方法寫入檔案,而 ReadFile
函式從下面程式中的文字檔案中讀取內容。如果 file.txt
不存在,應用程式將建立它,如果存在則截斷它。
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
)
func CreateFile() {
file, err := os.Create("file.txt")
if err != nil {
log.Fatalf("failed creating file: %s", err)
}
defer file.Close()
len, err := file.WriteString("Hello, How are you?.")
if err != nil {
log.Fatalf("failed writing to file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len)
fmt.Printf("\nFile Name: %s", file.Name())
}
func ReadFile() {
data, err := ioutil.ReadFile("file.txt")
if err != nil {
log.Panicf("failed reading data from file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len(data))
fmt.Printf("\nData: %s", data)
fmt.Printf("\nError: %v", err)
}
func main() {
fmt.Printf("//// Create a file and Write the content ////\n")
CreateFile()
fmt.Printf("\n\n //// Read file //// \n")
ReadFile()
}
輸出:
//// Create a file and Write the content ////
Length: 20 bytes
File Name: file.txt
//// Read file ////
Length: 20 bytes
Data: Hello, How are you?.
Error: <nil>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe