How to Read/Write From/to a File in Go
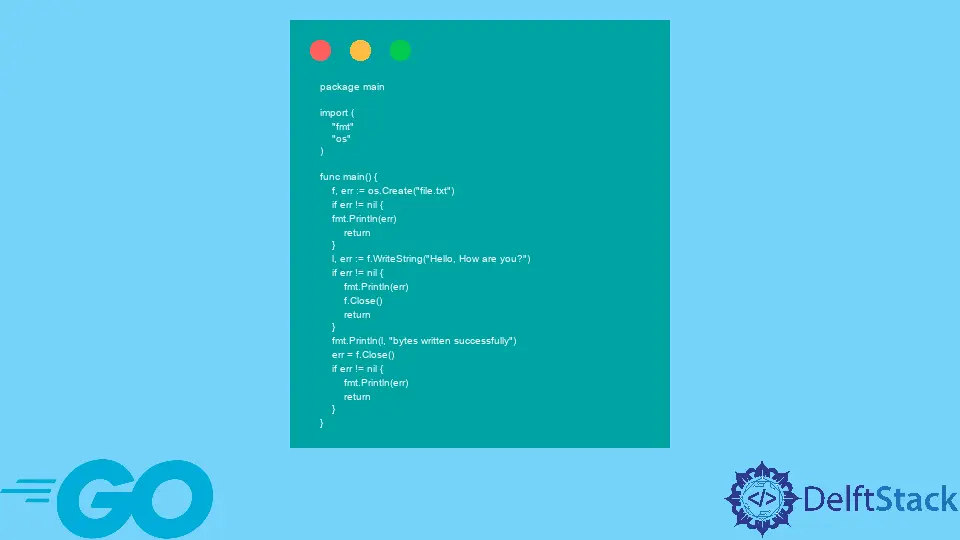
Data can be saved in a file, which gives it structure and allows it to be kept permanently or temporarily, depending on the storage media.
Programmatically working with a file includes obtaining metadata, creating new files, and reading and writing data to and from a file format into a program’s internal data structure.
Go’s built-in library provides excellent support for file-related activities such as creating, reading/writing, renaming, moving, and copying a file, and collecting its metadata information, among other things.
This tutorial will demonstrate how to read/write from/to a file in Go.
Write to a File Using WriteString
in Go
In this example, the file writing action involves writing a string to a file. This is an easy procedure to follow.
The following code will generate a file named file.txt
in the program’s directory. Then, the text Hello, How are you?
can be found in the file after you open it with any text editor.
Let’s have a look at the source code below.
package main
import (
"fmt"
"os"
)
func main() {
f, err := os.Create("file.txt")
if err != nil {
fmt.Println(err)
return
}
l, err := f.WriteString("Hello, How are you?")
if err != nil {
fmt.Println(err)
f.Close()
return
}
fmt.Println(l, "bytes written successfully")
err = f.Close()
if err != nil {
fmt.Println(err)
return
}
}
Output:
19 bytes written successfully
Read/Write From/to a File Using io/ioutil
in Go
The WriteString
method writes to a file, while the ReadFile
function reads content from a text file in the program below. If file.txt
does not exist, the application will create it or truncate it if it does.
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
)
func CreateFile() {
file, err := os.Create("file.txt")
if err != nil {
log.Fatalf("failed creating file: %s", err)
}
defer file.Close()
len, err := file.WriteString("Hello, How are you?.")
if err != nil {
log.Fatalf("failed writing to file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len)
fmt.Printf("\nFile Name: %s", file.Name())
}
func ReadFile() {
data, err := ioutil.ReadFile("file.txt")
if err != nil {
log.Panicf("failed reading data from file: %s", err)
}
fmt.Printf("\nLength: %d bytes", len(data))
fmt.Printf("\nData: %s", data)
fmt.Printf("\nError: %v", err)
}
func main() {
fmt.Printf("//// Create a file and Write the content ////\n")
CreateFile()
fmt.Printf("\n\n //// Read file //// \n")
ReadFile()
}
Output:
//// Create a file and Write the content ////
Length: 20 bytes
File Name: file.txt
//// Read file ////
Length: 20 bytes
Data: Hello, How are you?.
Error: <nil>