How to Read a File Into String in Go
-
Use the
ioutil.ReadFile()
Method in Go -
Use the
File.Read()
Method in Go -
Use the
buf.ReadFrom()
Method in Go -
Use the
strings.Builder
Method in Go
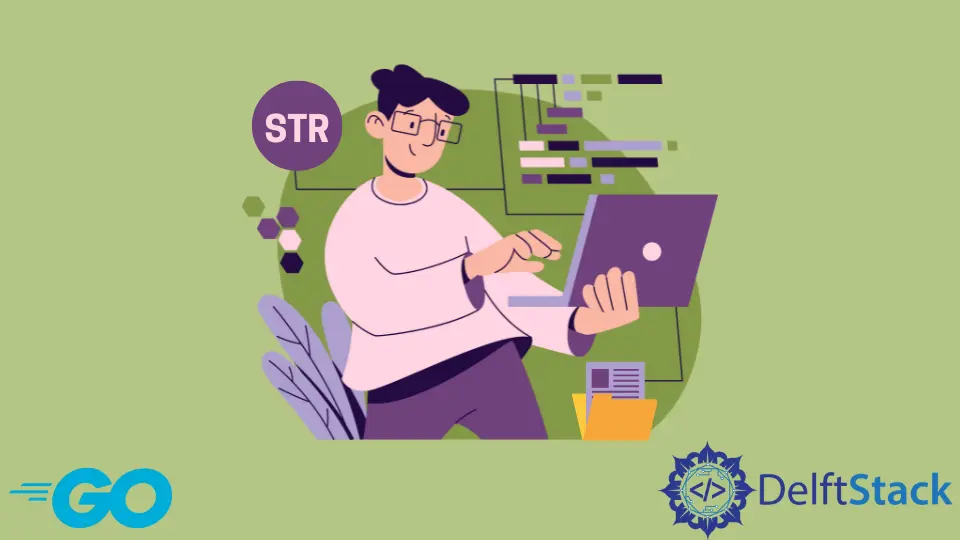
GoLang provides many file operations tools, one of which is how to read a file into a string. The ioutil.ReadFile()
, File.Read()
, buf.ReadFrom()
, and strings.Builder
are just a few of the methods that can be used to efficiently write a file’s content into a string.
Use the ioutil.ReadFile()
Method in Go
package main
import (
"fmt"
"io/ioutil"
)
func main() {
inputData := []byte("Hello, World!")
err := ioutil.WriteFile("File.txt", inputData, 0777)
Data, err := ioutil.ReadFile("File.txt")
if err != nil {
fmt.Println(err)
}
fmt.Print(string(Data))
}
Output:
Hello, World!
The above code allows us to create a new file and write data. The created file is then read using the ioutil.ReadFile()
method.
Use the File.Read()
Method in Go
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
"io"
)
func main() {
inputData := []byte("Hello, World!")
err := ioutil.WriteFile("file.txt", inputData, 0777)
f, err := os.Open("file.txt")
if err != nil {
log.Fatalf("Error in reading file: %v", err)
}
defer f.Close()
buf := make([]byte, 1024)
for {
n, err := f.Read(buf)
if err == io.EOF {
break
}
if err != nil {
fmt.Println(err)
continue
}
if n > 0 {
fmt.Println(string(buf[:n]))
}
}
}
Output:
Hello, World!
In the above code, we are reading the file by dividing it. We specify the buffer size we want to read each time, and then only len(buf)
is allocated in memory.
The file is then read in pieces through each iteration. This method is mostly used for large files, where a file’s content cannot fit entirely into memory.
Use the buf.ReadFrom()
Method in Go
package main
import (
"fmt"
"io/ioutil"
"os"
"bytes"
"log"
)
func main() {
inputData := []byte("Hello, World!")
err := ioutil.WriteFile("File.txt", inputData, 0777)
f, err := os.Open("File.txt")
if err != nil {
log.Fatal(err)
}
defer f.Close()
buf := new(bytes.Buffer)
buf.ReadFrom(f)
data := buf.String()
fmt.Print(data)
}
Output:
Hello, World!
In the above code, we have used buf.ReadFrom()
that reads data from the file until EOF
and appends it to the buffer; the buffer size grows as needed.
Use the strings.Builder
Method in Go
package main
import (
"io/ioutil"
"io"
"os"
"strings"
)
func main() {
inputData := []byte("Hello, World!")
err := ioutil.WriteFile("file.txt", inputData, 0777)
f, err := os.Open("file.txt")
if err != nil {
panic(err)
}
defer f.Close()
b := new(strings.Builder)
io.Copy(b, f)
print(b.String())
}
Output:
Hello, World!
In the above code, strings.Builder
concatenate the strings. The data is read from the file piece by piece and then put together to be printed.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedInRelated Article - Go File
- How to Efficiently Read a File Line by Line in GO
- How to Read/Write From/to a File in Go
- How to Check if a File Exists or Not in Go