How to Parse Date String in Go
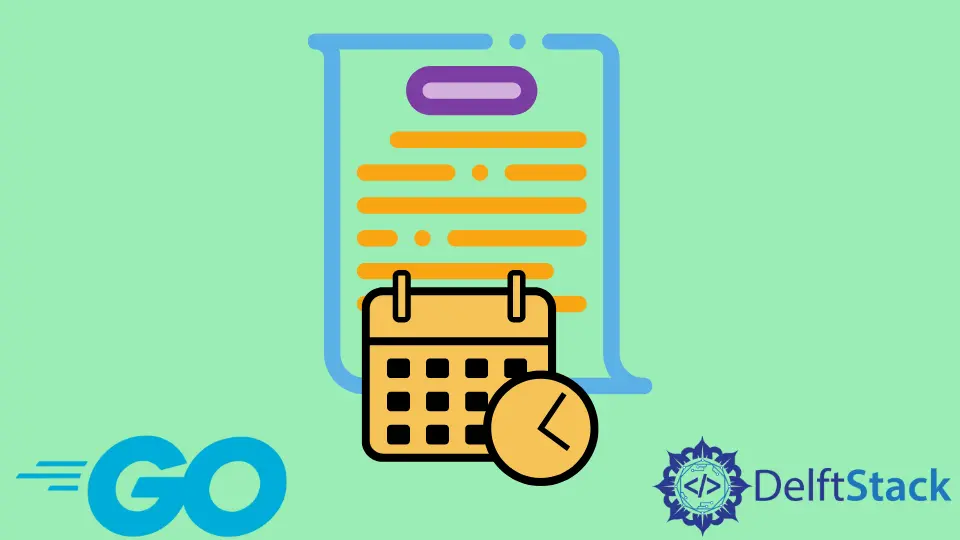
Date parsing is one of the most common tasks encountered in the programming world. Go uses a standard date, unlike other programming languages that use codes to represent parts of a date/time string representation. Parsing in Go is done with the help of Parse
and Format
functions in the time
package.
Representation of Date and Time in Go
To parse the date in Go, we use something called layout
. There is a standard time in Go which we need to remember while parsing dates in Go:
Mon Jan 2 15:04:05 MST 2006
It is January 2nd, 3:04:05 PM of 2006, UTC-0700
.
Parse Date String in Go
To parse a date string, we pass this layout to the time.Parse
function. We use real-time as the format without specifying the date using letters in parse
function which makes parse
function quite interesting.
time.Parse
function takes layout consisting of time format placeholder as a first argument and actual formatted string representing time as a second argument.
package main
import (
"fmt"
"time"
)
func main() {
timeT, _ := time.Parse("2006-01-02", "2020-04-14")
fmt.Println(timeT)
timeT, _ = time.Parse("06-01-02", "20-04-14")
fmt.Println(timeT)
timeT, _ = time.Parse("2006-Jan-02", "2020-Apr-14")
fmt.Println(timeT)
timeT, _ = time.Parse("2006-Jan-02 Monday 03:04:05", "2020-Apr-14 Tuesday 23:19:25")
fmt.Println(timeT)
timeT, _ = time.Parse("2006-Jan-02 Monday 03:04:05 PM MST -07:00", "2020-Apr-14 Tuesday 11:19:25 PM IST +05:30")
fmt.Println(timeT)
}
Output:
2020-04-14 00:00:00 +0000 UTC
2020-04-14 00:00:00 +0000 UTC
2020-04-14 00:00:00 +0000 UTC
0001-01-01 00:00:00 +0000 UTC
2020-04-14 23:19:25 +0530 IST
Go has some predefined time layout constant in its package.
Constant | Layout |
---|---|
ANSIC |
"Mon Jan _2 15:04:05 2006" |
UnixDate |
"Mon Jan _2 15:04:05 MST 2006" |
RubyDate |
"Mon Jan 02 15:04:05 -0700 2006" |
RFC822 |
"02 Jan 06 15:04 MST" |
RFC822Z |
"02 Jan 06 15:04 -0700" |
RFC850 |
"Monday, 02-Jan-06 15:04:05 MST" |
RFC1123 |
"Mon, 02 Jan 2006 15:04:05 MST" |
RFC1123Z |
"Mon, 02 Jan 2006 15:04:05 -0700" |
RFC3339 |
"2006-01-02T15:04:05Z07:00" |
RFC3339Nano |
"2006-01-02T15:04:05.999999999Z07:00" |
Stamp |
"3:04PM" |
StampMilli |
"Jan _2 15:04:05.000" |
StampMicro |
"Jan _2 15:04:05.000000" |
StampNano |
"Jan _2 15:04:05.000000000" |
We could use the predefined constant as above instead of layout string to parse the date-time.
package main
import (
"fmt"
"time"
)
func main() {
timeT, _ := time.Parse(time.RFC3339, "2020-04-15T13:45:26Z")
fmt.Println(timeT)
}
Output:
2020-04-15 13:45:26 +0000 UTC
If we have a time.Time
type, we use the Format
function to convert it to a string
.
package main
import (
"fmt"
"time"
)
func main() {
input := "2020-04-14"
layout := "2006-01-02"
t, _ := time.Parse(layout, input)
fmt.Println(t)
fmt.Println(t.Format("02-Jan-2006"))
}
Output:
2020-04-14 00:00:00 +0000 UTC
14-Apr-2020
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn