How to Efficiently Concatenate Strings in Go
-
With Plus (
+
) Operator -
With Multiple Arguments in
Print()
Function -
With
Join()
Function -
Sprintf()
Method -
bytes.Buffer
Method -
strings.Builder
Method -
Append With
+=
Operator - Comparison of Different Methods
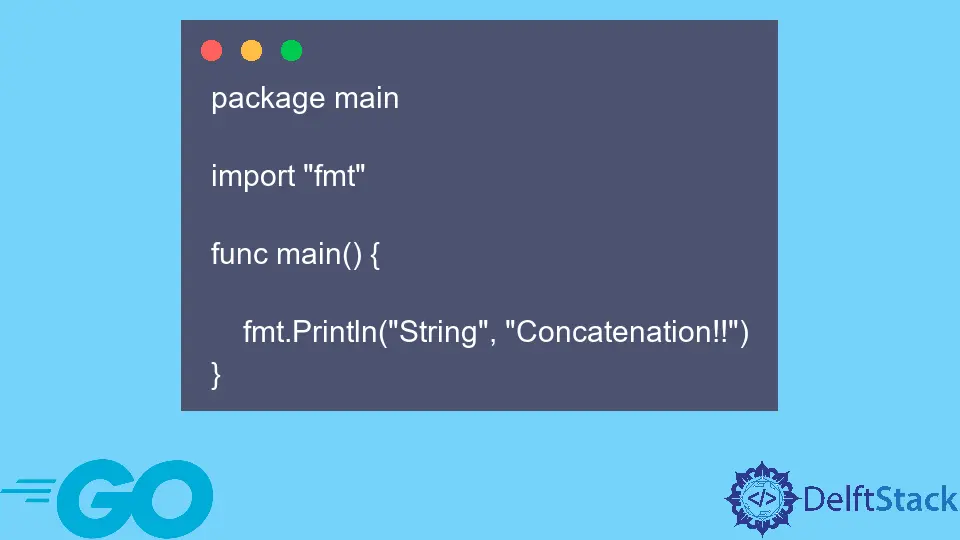
The act of merging two or more strings
into a single new string
is called String Concatenation
. String Concatenation
is one of the most widely performed operations in programming.
In this article, we will discuss different methods to concatenate strings in Go programming language with their pros and cons.
With Plus (+
) Operator
Using +
is the simplest and most widely used method for string concatenation. Sometimes +
operator is also called concatenation operator.
package main
import "fmt"
func main() {
str_1 := "Welcome to "
str_2 := "the Blog!!"
fmt.Println("str_1: ", str_1)
fmt.Println("str_2: ", str_2)
fmt.Println("Combined String: ", str_1+str_2)
}
Output:
str_1: Welcome to
str_2: the Blog!!
Combined String: Welcome to the Blog!!
With Multiple Arguments in Print()
Function
We can pass multiple strings as arguments to Print()
function in Go
. The strings are automatically concatenated by adding white space between strings.
package main
import "fmt"
func main() {
fmt.Println("String", "Concatenation!!")
}
Output:
String Concatenation!!
With Join()
Function
We also can concatenate strings using join()
function present in string package which takes a slice of strings and a separator to join them with producing a single string.
Syntax:
func Join(str []string, sep string) string
where str
is the slice of strings from which we concatenate elements and sep
is the separator placed between slices.
package main
import (
"fmt"
"strings" //for join() function
)
func main() {
str_slices := []string{"This", "is",
"String", "Concatenation!!"}
str_concat := strings.Join(str_slices, "-")
fmt.Println(str_concat)
}
Output:
This-is-String-Concatenation!!
Sprintf()
Method
Using Sprintf()
method in fmt
package is also one of the simplest methods for string concatenation.
package main
import "fmt"
func main() {
str_1 := "We will"
str_2 := " Rock"
str_3 := " You!!"
str_final := fmt.Sprintf("%s%s%s", str_1, str_2, str_3)
fmt.Println(str_final)
}
Output:
We will Rock You!!
bytes.Buffer
Method
An efficient way of concatenating strings without the generation of the unnecessary string object is the bytes.Buffer
method. We can write strings into byte buffer using WriteString()
method and then transform it into string.
package main
import (
"bytes"
"fmt"
)
func main() {
var byte_buf bytes.Buffer
byte_buf.WriteString("Hello ")
byte_buf.WriteString("World!!")
fmt.Println(byte_buf.String())
}
Output:
Hello World!!
strings.Builder
Method
This method allows efficient concatenation of strings by using very less memory compared to others.
package main
import (
"fmt"
"strings"
)
func main() {
var str_build strings.Builder
str_build.WriteString("Hello ")
str_build.WriteString("World!!")
fmt.Println(str_build.String())
}
Output:
Hello World!!
Append With +=
Operator
It is similar to string concatenation using the plus operator but slightly shorter than the previous one. +=
operator appends a new string to the right of the specified string.
package main
import "fmt"
func main() {
str_1 := "Hello "
str_1 += "World!!"
fmt.Println("Final String:", str_1)
}
Output:
Final String: Hello World!!
Comparison of Different Methods
bytes.Buffer
and strings.Builder
methods are quite faster than other methods of string concatenation. Hence when we have to perform string concatenation in a huge amount, we prefer bytes.Buffer
and strings.Builder
methods. However, when the number of concatenations is small, we can use +
or +=
operators.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn