How to Convert an Int Value to String in Go
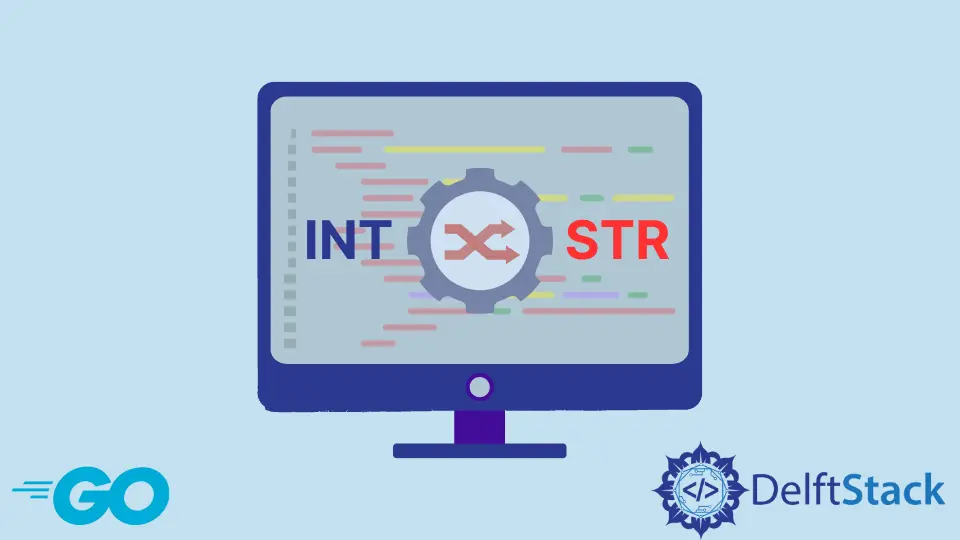
Data types determine the values that you can be assigned to the type and the operations that can be performed on it. Conversion of datatypes is widely used operation in programming and among datatype conversions conversion of int to the string value is widely popular.
It is used while printing number to the screen or working with the number as if it was a string. Go provides string and integer conversion directly from a package coming from the standard library strconv
.
If we use plain int
to string
conversion, the integer value is interpreted as a Unicode code point. And the resulting string will contain the character represented by the code point, encoded in UTF-8
.
package main
import "fmt"
func main() {
s := string(97)
fmt.Printf("Value of 97 after conversion : %v\n", s)
}
Output:
Value of 97 after conversion : a
But this is undesirable, and hence we use some standard functions to convert integers to the string data type, which are discussed below:
Itoa
Function From strconv
Package
Package strconv
implements conversions to and from string representations of basic data types. To convert an integer number into a string, we use Itoa
function from strconv
package.
package main
import (
"fmt"
"strconv"
)
func main() {
x := 138
fmt.Printf("Datatype of 138 before conversion : %T\n", x)
a := strconv.Itoa(x)
fmt.Printf("Datatype of 138 after conversion : %T\n", a)
fmt.Println("Number: " + a)
}
Output:
Datatype of 138 before conversion : int
Datatype of 138 after conversion : string
Number: 138
FormatInt
Function From strconv
Package
We use strconv.FormatInt
to format an int64
in a given base. FormatInt
gives the string representation of integer in the mentioned base, for 2
<= base
<= 36
and the result uses the lower-case letters a
to z
for digit values >= 10
.
package main
import (
"fmt"
"strconv"
)
func main() {
var integer_1 int64 = 31
fmt.Printf("Value of integer_1 before conversion : %v\n", integer_1)
fmt.Printf("Datatype of integer_1 before conversion : %T\n", integer_1)
var string_1 string = strconv.FormatInt(integer_1, 10)
fmt.Printf("Value of integer_1 after conversion in base 10: %v\n", string_1)
fmt.Printf("Datatype of integer_1 after conversion in base 10 : %T\n", string_1)
var string_2 string = strconv.FormatInt(integer_1, 16)
fmt.Printf("Value of integer_1 after conversion in base 16 : %v\n", string_2)
fmt.Printf("Datatype of integer_1 after conversion in base 16 : %T\n", string_2)
}
Output:
Value of integer_1 before conversion : 31
Datatype of integer_1 before conversion : int64
Value of integer_1 after conversion in base 10: 31
Datatype of integer_1 after conversion in base 10 : string
Value of integer_1 after conversion in base 16 : 1f
Datatype of integer_1 after conversion in base 16 : string
fmt.Sprint
Method
When we pass an integer into the fmt.Sprint
method, we get a string value of the integer.
package main
import (
"fmt"
)
func main() {
x := 138
fmt.Printf("Datatype of 138 before conversion : %T\n", x)
a := fmt.Sprint(x)
fmt.Printf("Datatype of 138 after conversion : %T\n", a)
fmt.Println("Number: " + a)
}
Output:
Datatype of 138 before conversion : int
Datatype of 138 after conversion : string
Number: 138
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn