Go에 파일이 있는지 여부 확인
Jay Singh
2022년8월23일
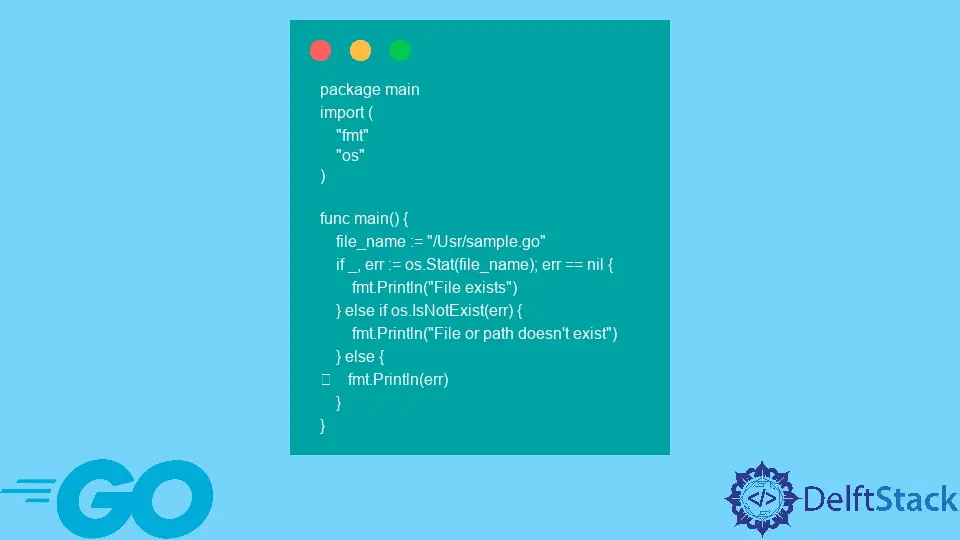
이 문서에서는 Go에 파일이 있는지 여부를 확인하는 방법에 대해 설명합니다.
IsNotExist()
및 Stat()
를 사용하여 파일이 Go에 있는지 여부 확인
Go 프로그래밍 언어의 os
패키지에서 IsNotExist()
및 Stat()
메서드를 사용하여 파일이 존재하는지 확인합니다.
Stat()
함수는 파일에 대한 정보가 포함된 객체를 반환합니다. 파일이 존재하지 않으면 오류 개체를 생성합니다.
다음은 IsNotExist()
및 Stat()
를 사용하는 코드의 예입니다.
예 1:
package main
import (
"fmt"
"os"
)
// function to check if file exists
func doesFileExist(fileName string) {
_, error := os.Stat(fileName)
// check if error is "file not exists"
if os.IsNotExist(error) {
fmt.Printf("%v file does not exist\n", fileName)
} else {
fmt.Printf("%v file exist\n", fileName)
}
}
func main() {
// check if demo.txt exists
doesFileExist("demo.txt")
// check if demo.csv exists
doesFileExist("demo.csv")
}
출력:
demo.txt file exist
demo.csv file does not exist
예 2:
package main
import (
"fmt"
"os"
)
func main() {
file_name := "/Usr/sample.go"
if _, err := os.Stat(file_name); err == nil {
fmt.Println("File exists")
} else if os.IsNotExist(err) {
fmt.Println("File or path doesn't exist")
} else {
fmt.Println(err)
}
}
출력:
File or path doesn't exist