Comprobar si un archivo existe o no en Go
Jay Singh
23 agosto 2022
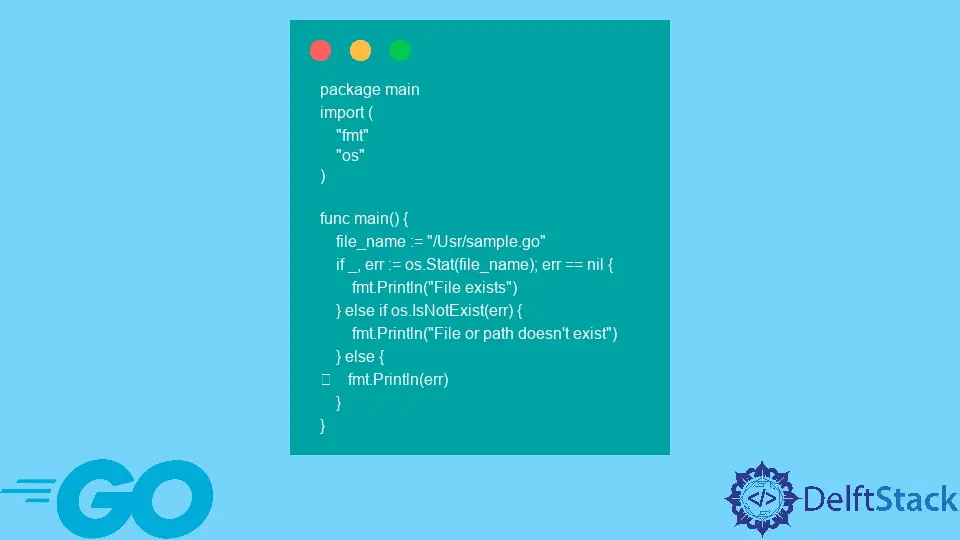
Este artículo discutirá cómo verificar si un archivo existe o no en Go.
Utilice IsNotExist()
y Stat()
para comprobar si el archivo existe o no en Go
Usamos los métodos IsNotExist()
y Stat()
del paquete os
en el lenguaje de programación Go para determinar si un archivo existe.
La función Stat()
devuelve un objeto que contiene información sobre un archivo. Si el archivo no existe, genera un objeto de error.
A continuación se muestran ejemplos de código que utilizan IsNotExist()
y Stat()
.
Ejemplo 1:
package main
import (
"fmt"
"os"
)
// function to check if file exists
func doesFileExist(fileName string) {
_, error := os.Stat(fileName)
// check if error is "file not exists"
if os.IsNotExist(error) {
fmt.Printf("%v file does not exist\n", fileName)
} else {
fmt.Printf("%v file exist\n", fileName)
}
}
func main() {
// check if demo.txt exists
doesFileExist("demo.txt")
// check if demo.csv exists
doesFileExist("demo.csv")
}
Producción :
demo.txt file exist
demo.csv file does not exist
Ejemplo 2:
package main
import (
"fmt"
"os"
)
func main() {
file_name := "/Usr/sample.go"
if _, err := os.Stat(file_name); err == nil {
fmt.Println("File exists")
} else if os.IsNotExist(err) {
fmt.Println("File or path doesn't exist")
} else {
fmt.Println(err)
}
}
Producción :
File or path doesn't exist