How to Create a White RGBA in HTML
-
Use
background:rgba()
to Create a White RGBA in HTML - Use Hexadecimal Color Codes to Create a White RGBA in HTML
- Use HSLA Functional Notation to Create a White RGBA in HTML
- Conclusion
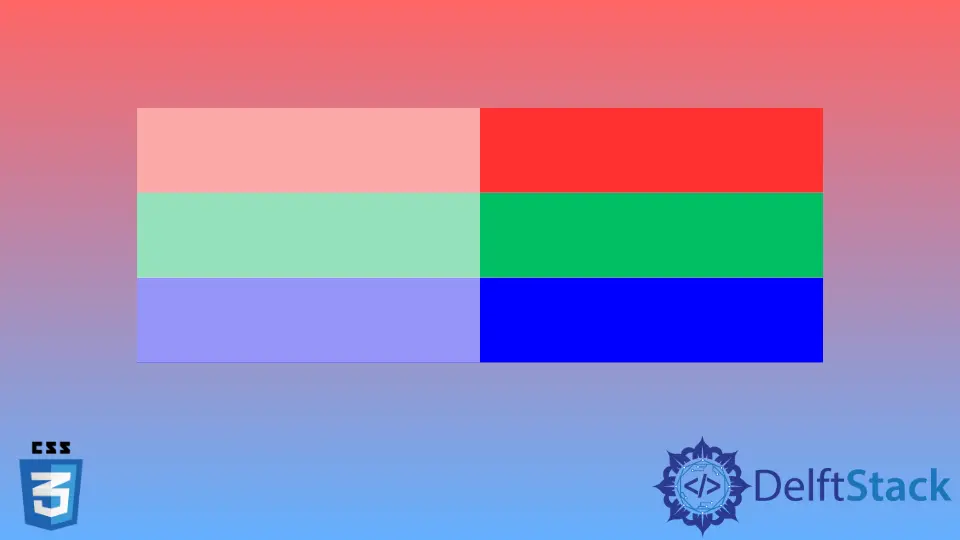
When it comes to web design, having a versatile color palette is crucial. One commonly used color is white, which can be combined with varying levels of transparency using RGBA (Red, Green, Blue, Alpha) values.
In this article, we will explore several methods to create a white RGBA color in HTML, providing detailed explanations and example codes for each approach.
Use background:rgba()
to Create a White RGBA in HTML
The rgba()
function is a CSS function that allows you to specify a color in terms of its red, green, blue, and alpha components. The alpha component controls the level of transparency, with a value of 0
representing full transparency and 1
representing full opacity.
The intensity of RGB can vary from 0-255
, but the value of Alpha only ranges from 0.0
(not visible/fully transparent) to 1.0
(visible/not transparent).
We can apply the white RGBA with the rgba()
function as a layer to some other colors. Thus, we can see the effects of white RGBA.
When we set the value 255
to all the color options, it will create a white color. We can set the opacity to make the layer visible.
Basic Usage of background:rgba()
To create a white RGBA color using the background
property with the rgba()
function, you can follow this basic structure:
.element {
background: rgba(255, 255, 255, 0.5);
}
In this example, the rgba()
function is used to set the background color to white (255
for red, green, and blue, representing full intensity). The last parameter, 0.5
, determines the level of transparency, making the element 50% opaque.
Applying Transparent White RGBA Background
For example, in HTML, create five div
elements and name the classes as layer
, blue
, yellow
, red
, and black
. Then, in CSS, select all the classes except layer
and set the height
to 40px
and width
to 100%
. Then select the yellow
class and set the background
to yellow
.
Similarly, set the background to all of the classes like in the below code. Next, select the layer
class and apply some styles to it.
Set width
to 50%
and height
to 400px
. Apply the absolute
positioning and set the background
to rgba(255,255,255,0.5)
.
<div class="layer"></div>
<div class="blue"></div>
<div class="yellow"></div>
<div class="red"></div>
<div class="black"></div>
.blue,.yellow,.red,.black {
height:40px;
width:100%;
}
.yellow{
background:yellow;
}
.blue{
background:blue;
}
.red{
background:red;
}
.black{
background:black;
}
.layer {
width:50%;
height:400px;
position:absolute;
background:rgba(255,255,255,0.5);
}
In the example above, we created four containers for the four colors. We applied a 100%
width to all these colors, and then we created an overlay with the layer
class.
We divided the whole width in half and applied a white RGBA. We used the value 255
for each RGB value and the opacity of 0.5
for it, so it created a white background overlay to the original colors.
We can see the opacity of the original color increasing in the first half section of the colors. We can change the opacity value according to our wish to see the changes.
The higher opacity value in the white color makes the background colors less transparent and vice versa. Thus, we can create a white RGBA overlay above the other colors.
Combining with Other Background Properties
The background
property can accept multiple values, allowing you to combine different background properties. For example:
.element {
background: url('background-image.jpg') rgba(255, 255, 255, 0.5) no-repeat center center;
}
In this example, we combine a background image with a white background with 50% opacity.
Using the background-color
Property
You can also achieve the same effect using the background-color
property:
.element {
background-color: rgba(255, 255, 255, 0.5);
}
Both the background
and background-color
properties will yield the same result.
Use Hexadecimal Color Codes to Create a White RGBA in HTML
Hexadecimal color codes represent colors using a base-16 numbering system. They consist of six characters, comprising of letters (A-F) and numbers (0-9).
Each pair of characters represents the intensity of red, green, and blue, respectively, allowing for a total of 16.8 million possible colors.
For white, the hexadecimal color code is #FFFFFF
, where FF
represents the highest intensity for red, green, and blue.
Hexadecimal color codes are a widely used method for defining colors in web development. To create a white RGBA color using hexadecimal codes, we’ll first define a base color of white (#FFFFFF
) and then adjust the alpha value to control the level of transparency.
<!DOCTYPE html>
<html>
<head>
<title>White RGBA using Hexadecimal Codes</title>
<style>
.white-rgba {
background-color: #FFFFFF; /* Hexadecimal code for white */
opacity: 0.7; /* Adjust opacity value (0.0 to 1.0) */
width: 100px;
height: 100px;
}
</style>
</head>
<body>
<div class="white-rgba"></div>
</body>
</html>
In the code above, we start by defining a <div>
element with the class white-rgba
.
In the CSS section, we set the background-color
property to #FFFFFF
, which represents pure white in hexadecimal format.
Next, we use the opacity
property to adjust the transparency level. In this example, opacity: 0.7;
makes the white background 70% opaque.
Finally, we set the width
and height
properties to give the <div>
a visible size.
Use HSLA Functional Notation to Create a White RGBA in HTML
HSLA is a color representation format that defines colors in terms of their Hue, Saturation, Lightness, and Alpha (transparency) values.
- Hue: Represents the type of color (e.g., red, blue, green) on a 360-degree color wheel.
- Saturation: Determines the intensity or vividness of a color.
0%
saturation results in grayscale, while100%
saturation is fully vivid. - Lightness: Indicates the brightness of the color.
0%
is black,100%
is white, and values in between represent varying levels of brightness. - Alpha: Specifies the level of transparency. A value of
0
is completely transparent, while1
is fully opaque.
To create a white RGBA color using HSLA functional notation, we’ll set the Hue and Saturation to values that produce white and then adjust the Alpha value for the desired level of transparency.
<!DOCTYPE html>
<html>
<head>
<title>White RGBA using HSLA Functional Notation</title>
<style>
.white-rgba {
background-color: hsla(0, 0%, 100%, 0.5); /* Adjust alpha value (0.0 to 1.0) */
width: 100px;
height: 100px;
}
</style>
</head>
<body>
<div class="white-rgba"></div>
</body>
</html>
In the above example, we have set the background-color
property to hsla(0, 0%, 100%, 0.5)
.
The hue is set to 0
degrees, and it represents the color red. Since we want white, the hue is set to 0
.
The saturation has been set to 0%
, meaning no colorfulness, resulting in grayscale. Lightness is set to 100%
to show a fully white color.
Lastly, alpha is set to 0.5
, making the transparency level to 50%.
Experimenting with HSLA Values
You can experiment with different HSLA values to achieve various shades of white with different levels of transparency. For example:
hsla(0, 0%, 100%, 0.2)
will give a very light gray with 20% opacity.hsla(0, 0%, 100%, 0.8)
will produce a very faint gray with 80% opacity.
By adjusting these values, you can create a wide range of white colors with varying degrees of transparency to suit your design needs.
Conclusion
This article provides a comprehensive guide on creating a white RGBA color in HTML using three different methods: RGBA functional notation, hexadecimal color codes, and HSLA functional notation.
- RGBA Functional Notation:
- This method utilizes the
rgba()
function in CSS to specify the red, green, blue, and alpha components for a white background. - It offers precise control over transparency levels, making it a versatile choice for web designers.
- This method utilizes the
- Hexadecimal Color Codes:
- Hexadecimal color codes, represented by six characters, are widely used in web development.
- By starting with the base color white (
#FFFFFF
) and adjusting the alpha value, designers can achieve the desired level of transparency.
- HSLA Functional Notation:
- HSLA notation represents colors in terms of Hue, Saturation, Lightness, and Alpha values.
- By setting the hue and saturation for white and adjusting the alpha value, a white RGBA color with varying transparency levels can be created.
Each method has its advantages and allows for customization based on specific design requirements. Whether preferring the intuitive control of HSLA, the familiarity of hexadecimal codes, or the fine-tuning capabilities of RGBA functional notation, designers now have a comprehensive understanding of creating a white RGBA color in HTML.
Experimentation with these techniques will open up a world of possibilities for web design projects.
Sushant is a software engineering student and a tech enthusiast. He finds joy in writing blogs on programming and imparting his knowledge to the community.
LinkedIn