How to Write a Backslash in a String in C#
-
Backslash (
\
) Character in C# -
Use Double Backslash (
\
) to Write a Backslash in the String in C# -
Use
@
Verbatim Strings to Write Backslash in a String in C# - Conclusion
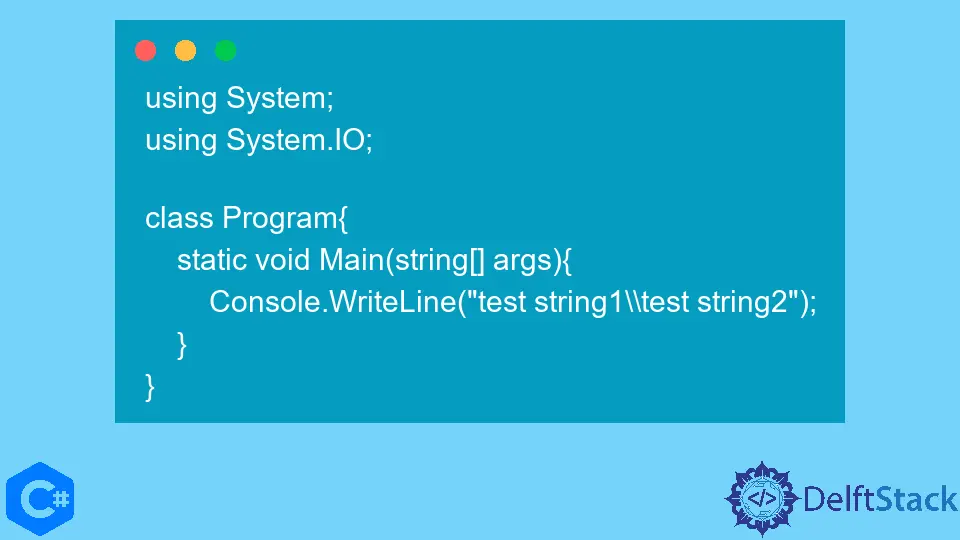
Strings are a fundamental data type in any programming language, including C#. They allow us to represent text and characters within our programs.
However, when dealing with special characters like the backslash (\
), we need to handle them carefully, as they have a special meaning in string literals.
In this article, we will explore how to write a backslash in a string in C#, ensuring that it appears as intended and does not interfere with the string formatting.
Backslash (\
) Character in C#
A single backslash (\
), combined with another character in the string known as the escape sequence and characters, performs a specific action or function. When inserted inside a string literal of a code, an escape sequence is a series of string characters that represent anything but themselves double-quoted.
For example, \t
gives a tab space, \a
gives an audible alert, and \n
enters a new line.
There are two methods to write a backslash \
character in C#. Both methods work the same way to write a backslash \
character in C# in the output or the string and are discussed and implemented below.
Use Double Backslash (\
) to Write a Backslash in the String in C#
The most common and widely used method to include a backslash in a string in C# is to use the double backslash (\\
) escape sequence. The compiler interprets this sequence as a single backslash in the resulting string.
Here’s a step-by-step guide to using the double backslash escape sequence:
-
Declare a string variable where you want to include the backslash.
string backslashString;
-
Assign the string value using the double backslash escape sequence to represent a single backslash.
backslashString = "This is a backslash: \\";
In this example,
"This is a backslash: \\"
will result in a string with the value"This is a backslash: \"
, where the double backslash is interpreted as a single backslash. -
Now, you can use the
backslashString
variable wherever you need a string with a backslash.Console.WriteLine(backslashString); // Output: This is a backslash: \
The
Console.WriteLine
method will print the string with a single backslash to the console.
Using the double backslash escape sequence is the standard and recommended way to include a backslash in a string in C#.
Here’s a complete working C# code example demonstrating how to write a backslash in a string using the escape sequence \\
:
using System;
class Program {
static void Main(string[] args) {
string backslashString = "This is a backslash: \\";
Console.WriteLine(backslashString); // Output: This is a backslash: \
string filePath = "C:\\MyFolder\\MyFile.txt";
Console.WriteLine("File path: " + filePath); // Output: File path: C:\MyFolder\MyFile.txt
}
}
When you run this program, you will see the following output:
This is a backslash: \
File path: C:\MyFolder\MyFile.txt
As you can see, inside Main
, we demonstrate two instances of using the escape sequence \\
to represent a backslash within strings.
First, we assign the string "This is a backslash: \\"
to a variable named backslashString
. When printed to the console, this variable displays This is a backslash: \
, showcasing the correct usage of the escape sequence.
Next, we depict an example of utilizing a backslash in a file path by assigning "C:\\MyFolder\\MyFile.txt"
to another variable named filePath
. This illustrates how escape sequences are crucial in accurately representing backslashes in file paths.
The file path is then printed to the console, demonstrating the expected output of File path: C:\MyFolder\MyFile.txt
.
Use @
Verbatim Strings to Write Backslash in a String in C#
Sometimes, you may want to include a literal backslash (\
) in your string without escaping any character. This is where the verbatim string literal comes in handy.
To create a verbatim string literal, you prefix the string with the @
symbol like this:
string path = @"C:\MyDocuments";
In the above example, the backslash (\
) is included as a literal in the string without any need for escaping. If a string begins with @
and ends with double quotes, the compiler recognizes it as a verbatim string and compiles it.
The major benefit of the @
symbol is that it instructs the string constructor to disregard the escape characters and line breaks.
Let’s say you want to represent a file path in your program, and you want to include a backslash in the path. Here’s how you would use a verbatim string literal to achieve this:
using System;
class Program {
static void Main() {
string filePath = @"C:\MyDocuments\File.txt";
Console.WriteLine("File path: " + filePath);
}
}
Output:
File path: C:\MyDocuments\File.txt
Within the Main
method, a string variable filePath
is declared and initialized with a file path "C:\MyDocuments\File.txt"
.
The verbatim string literal, denoted by @
before the string, allows the inclusion of backslashes directly without requiring escaping. Therefore, we get the output: File path: C:\MyDocuments\File.txt
.
Let’s write another code that uses the @
symbol to ignore any escape sequences and output the plain backslash.
using System;
using System.IO;
class Program {
static void Main(string[] args) {
Console.WriteLine(@"test string1\test string2");
}
}
Output:
test string1\test string2
As we can see, the string "test string1\test string2"
is passed to the Console.WriteLine
method with the @
symbol prefixing the string.
This ensures that the backslash in the string is treated as a literal character rather than an escape character; hence, we get the exact specified string: test string1\test string2
.
Conclusion
Handling special characters like the backslash (\
) in strings is a crucial aspect of programming in C#. To include a backslash in a string, you can either utilize double backslashes (\\
) to escape the backslash or opt for a verbatim string literal (@
prefix) to directly incorporate the backslash.
Choose the appropriate approach based on your coding style and specific requirements. By understanding and applying these techniques, you can effectively manage backslashes within strings, ensuring accurate representation in your C# programs.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn