How to Validate Email Address in C#
-
Validate Email Address With the
MailAddress
Class inC#
-
Validate Email Address With the
EmailAddressAttribute
Class inC#
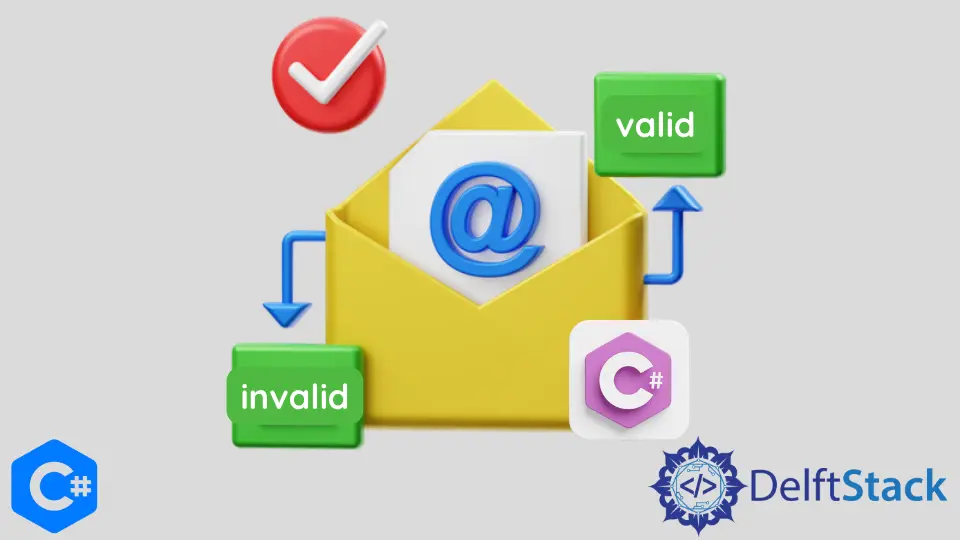
This tutorial will discuss methods to validate an email address in C#.
Validate Email Address With the MailAddress
Class in C#
The first thing that comes into mind when we talk about checking formats is regular expressions. But regular expressions are relatively complex, and a lot of time is required to learn regular expressions. If you already know regular expressions, then that is the best approach for you. This tutorial will use some pre-defined methods instead of any user-defined approaches to validate email addresses in C#. The MailAddress
class is used to represent an email address in C#. The constructor of the MailAddress
class takes a string and formats it into an email address. We can use the MailAddress
class to determine whether a given email address is valid or not. The following code example shows us how we can validate an email address with the MailAddress
class in C#.
using System;
namespace email_validation {
class Program {
static bool IsValidEmail(string email) {
try {
var addr = new System.Net.Mail.MailAddress(email);
return addr.Address == email;
} catch {
return false;
}
}
static void Main(string[] args) {
bool isOk = IsValidEmail("mma @gma.com");
Console.WriteLine(isOk);
}
}
}
Output:
false
We defined the function IsValidEmail(email)
that takes an email address as a string and returns true
if the email is valid and false if the email is invalid
. We used the constructor of the MailAddress
class inside the System.Net.Mail
namespace to create a new instance of the MailAddress
class. We then compared the Address
property of the MailAddress
class with our email address. If the Address
matches the email, the email is valid
. If the Address
doesn’t match the email or an exception occurs during the process, the email is invalid
.
Validate Email Address With the EmailAddressAttribute
Class in C#
The EmailAddressAttribute
class is used to validate an email address in C#. The IsValid(email)
function of the EmailAddressAttribute
class returns true
if the email
is valid or null and false
if the email
is invalid. The following code example shows us how to determine whether an email address is valid or invalid with the EmailAddressAttribute
class in C#.
using System;
using System.ComponentModel.DataAnnotations;
namespace email_validation {
class Program {
static bool validateEmail(string email) {
if (email == null) {
return false;
}
if (new EmailAddressAttribute().IsValid(email)) {
return true;
} else {
return false;
}
}
static void Main(string[] args) {
bool isOk = validateEmail("mma@gma.com");
Console.WriteLine(isOk);
}
}
}
Output:
true
We created the function validateEmail()
that takes an email address as a string parameter and returns true
if the email is valid and returns false
if it is invalid. The validateEmail()
function first returns false if the email
is null
. We created a new instance of the EmailAddressAttribute
class and passed our email
as a parameter to the IsValid()
function of the EmailAddressAttribute
class.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn