Thread vs Task in C#
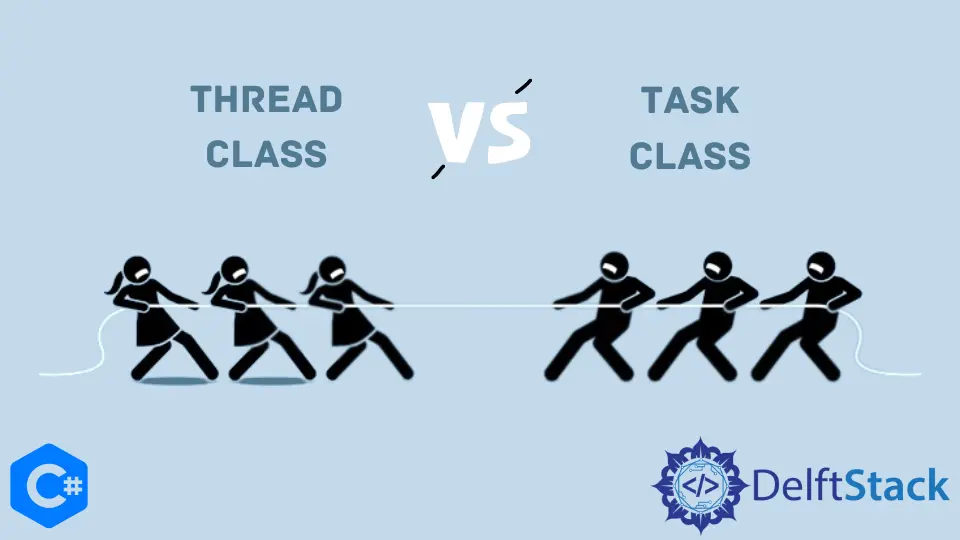
This tutorial will discuss the similarities and differences between the Thread
class and the Task
class in C#.
Thread in C#
The Thread
class creates an actual operating system level thread in C#. The thread created with the Thread
class takes resources like memory for the stack, and CPU overhead for the context switches from one thread to another. The Thread
class provides the highest degree of control like the Abort()
function, the Suspend()
function, the Resume()
function, etc. We can also specify some thread-level attributes like stack size. The following code example shows us how we can create a thread with the Thread
class in C#.
using System;
using System.Threading;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Thread thread1 = new Thread(new ThreadStart(fun1));
Thread thread2 = new Thread(new ThreadStart(fun2));
thread1.Start();
thread2.Start();
Console.WriteLine("The End");
}
}
}
Output:
The End
Thread 2
Thread 2
Thread 1
Thread 1
In the above code, we created threads thread1
and thread2
with the Thread
class in C#.
Task in C#
A Task
class creates an asynchronous system-level task in C#. The task scheduler executes the tasks created with the Task
class. The default scheduler runs the task inside the thread pool. Unlike the threads created with the Thread
class, the tasks created with the Task
class do not require any additional memory or CPU resources. The Task
class cannot be used to specify the thread-level attributes like stack size. Since the Task
class runs on the thread pool, any long-running tasks can fill up the thread pool, and the new task can end up waiting for the previous tasks to complete execution. The following code example shows us how we can create a task with the Task
class in C#.
using System;
using System.Threading;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Task task1 = Task.Factory.StartNew(() => fun1());
Task task2 = Task.Factory.StartNew(() => fun2());
Task.WaitAll(task1, task2);
Console.WriteLine("The End");
}
}
}
Output:
Thread 1
Thread 1
Thread 2
Thread 2
The End
We created tasks task1
and task2
with the Task
class in C# in the above code.
Thread vs Task in C#
Both the Thread
class and the Task
class are used for parallel programming in C#. A Thread
is a lower-level implementation while a Task
is a higher-level implementation. It takes resources while a Task
does not. It also provides more control than the Task
class. A Thread
should be preferred for any long-running operations, while a Task
should be preferred for any other asynchronous operations.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Thread
- Thread.Sleep() in C#
- How to Stop a Thread in C#
- Thread Safe List in C#
- How to Wait for a Thread to Finish in C#
- lock Statement in C#