Thread.Sleep() in C#
-
Why
thread.sleep()
Is Harmful inC#
-
How
thread.sleep()
Works inC#
- Asynchronous Programming
-
Run Two Methods Simultaneously (
async
andawait
Keywords in C#)
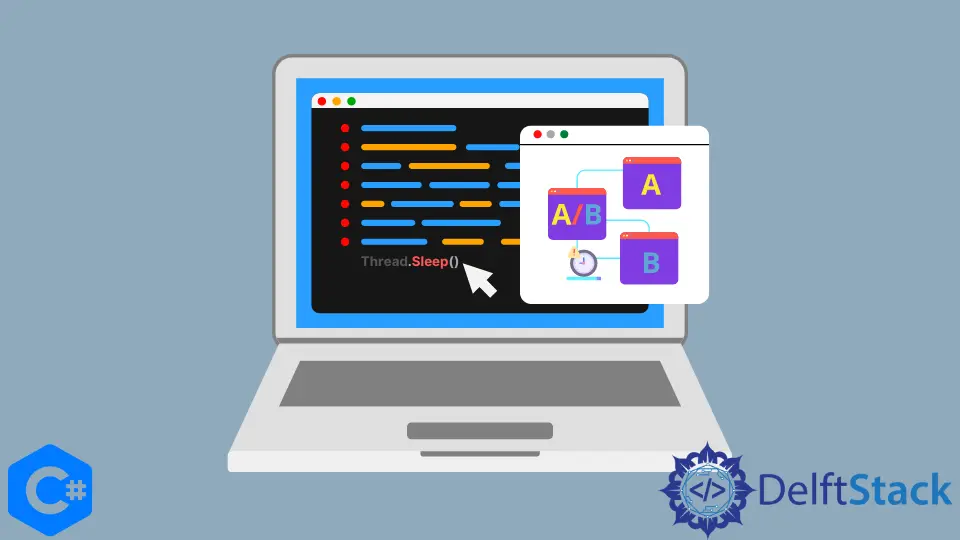
In this guide, we will learn why the use of thread.sleep()
in C# is considered harmful and why this particular approach should not be used.
What happens by using thread.sleep()
? Is there any alternative approach?
Find out everything in this guide. Let’s dive in.
Why thread.sleep()
Is Harmful in C#
To understand why thread.sleep()
is harmful, we must understand the work behind this method. Well, in C#, the sleep
method has its use.
You can use it to pause or suspend the current functionality (thread execution) for a specific period. We can pass the time in milliseconds to suspend the thread execution for some time.
We can also use the TimeSpan
property, as shown in the code below.
// Time in milliseconds
Thread.Sleep(5000);
Or
// Time in hours, minutes, seconds
TimeSpan tsObj = new TimeSpan(0, 0, 5);
Thread.Sleep(tsObj);
Now, both of the above approaches will stop the program for five seconds. This means, through the above code, we are trying to suspend the thread for 1 second.
But, what is the meaning of all this? Let’s understand the working of it.
How thread.sleep()
Works in C#
We all know that a program runs line-wise, meaning the whole program runs synchronously, one line after another. Sometimes, during programming, we need to perform a task that takes some time to be completed.
For instance, fetching the data from an API, we can use the sleep
function to let it wait, get that data from API, and run the rest of the program later. This is necessary yet a very harmful and poor approach.
For instance, the data we are trying to fetch could be big and take time. Our program will be stopped during that time; that’s not an ideal approach.
Thread.Sleep(n)
means stopping the running thread for as many time slices (also known as thread quantums) as possible in n
milliseconds. A time slice’s duration varies depending on the version/type of Windows and the processor used, but it typically lasts between 15 and 30 milliseconds.
This indicates that the thread will likely block for longer than n
milliseconds. It’s unlikely that your thread will re-awaken precisely n
milliseconds later.
Thus, Thread.Sleep
serves no purpose in timing.
Threads are a finite resource that requires roughly 200,000 cycle creation and 100,000 cycle destruction. By default, they use 2,000–8,000 cycles for each context transition and reserve 1 megabyte of virtual memory for their stack.
What’s the Alternative Approach
We sometimes need to delay our thread for the program to run perfectly. As mentioned above, we could need to fetch some API data, which takes time.
But stopping the whole program because of it is a bad approach. So, how do we avoid that?
Let’s introduce you to asynchronous programming.
Asynchronous Programming
The async
and await
keywords in C# help make asynchronous programming highly common these days. The entire application will have to wait to complete the operation when working with UI and use a long-running method that will take a long time, such as reading a huge file or clicking on a button.
In other words, if any process in a synchronous application becomes blocked, the entire application also becomes halted, and our application ceases to function until the entire task has been completed.
In this situation, asynchronous programming is highly useful. The application can carry on with tasks that don’t depend on completion by employing asynchronous programming.
With the aid of the async
and await
keywords, we will achieve all the advantages of conventional asynchronous programming with much less work.
Run Two Methods Simultaneously (async
and await
Keywords in C#)
Let’s say we have two methods, FirstMethod()
and SecondMethod()
, that are independent of one another and that FirstMethod()
takes a long time to perform its task.
Synchronous programming starts with FirstMethod()
and waits for it to finish before moving on to SecondMethod()
, which is then executed. Even if both procedures are independent, the process will take a lot of time.
We can run all the functions concurrently by utilizing simple thread programming, but this would block the user interface while we wait for the tasks to be finished. In traditional programming, we would need to write a lot of code to solve this issue, but if we utilize the async
and await
keywords, we can solve the issue with a lot less code.
We’ll see other examples as well, and in those cases, when SecondMethod()
depends on FirstMethod()
, it will use the await
keyword to wait for FirstMethod()
to finish running.
In C#, async
and await
are code markers that indicate where the control should return when a task has been completed.
Starting with real-world examples will help you better comprehend the programming behind them. Take a look at the following code.
class Program {
static void Main(string[] args) {
FirstMethod();
SecondMethod();
Console.ReadKey();
}
public static async Task FirstMethod() {
await Task.Run(() => {
for (int i = 0; i < 100; i++) {
Console.WriteLine(" First Method");
// Do something
Task.Delay(100).Wait();
}
});
}
public static void SecondMethod() {
for (int i = 0; i < 25; i++) {
Console.WriteLine(" Second Method ");
// Do something
Task.Delay(100).Wait();
}
}
}
The output of the above code will be as follows.
First Method
Second Method
Second Method
First Method
Second Method
First Method
Second Method
First Method
Second Method
First Method
Second Method
First Method
Second Method
First Method
Second Method
Second Method
First Method
Second Method
First Method
Second Method
First Method
Second Method
First Method
Second Method
First Method
Second Method
Second Method
Second Method
Second Method
Second Method
As you can see in the above code, that whole program does not stop just because we have to stop executing one thread. They both can run simultaneously with the use of asynchronous programming.
Let’s say you are at some point where you have to fetch some API data, which takes time. So, the program will move to the next line without getting the data, so we need to delay it a bit to get that data and then move on to the next line.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn