How to Stop a Thread in C#
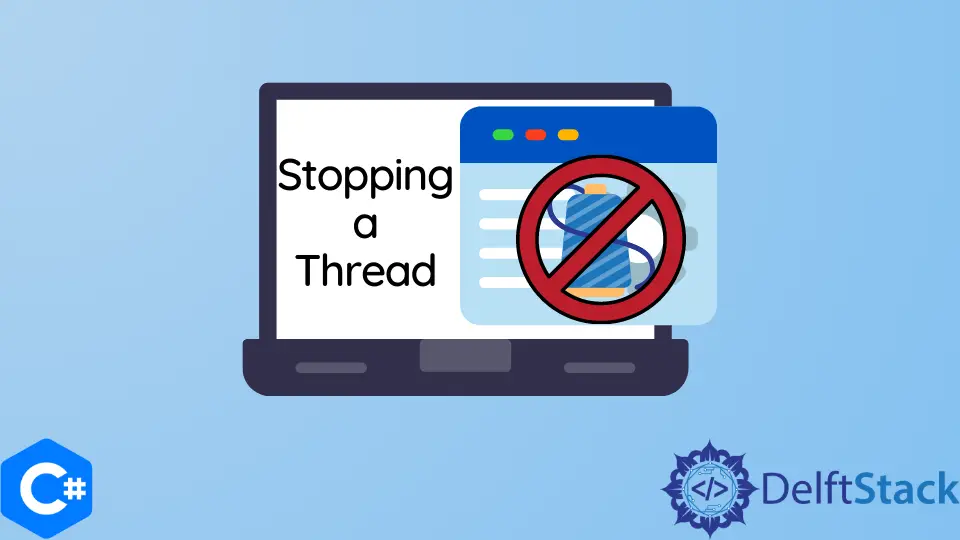
At times, while working with a thread in C#, you might come upon a situation where you will need to terminate a thread. C# does provide methods for you to do that successfully, and this article aims to explain the process of terminating a thread in C#.
For our goal of terminating a thread, we’ll utilize the Abort()
method. The ThreadAbortException
is thrown by the Abort()
method to the thread that called it, and as a response to this exception, the thread is terminated.
Before we can stop a thread, we must first initiate one. To accomplish creating a thread, follow the steps given below.
Use the Abort()
Method to Stop a Thread in C#
To utilize the System.Threading
library’s methods to start and end a thread, import it.
using System.Threading;
We’ll need to make a ThreadDemo
class.
class ThreadDemo {}
We’ll create a thread method named threadAssign()
within this class, with a loop that iterates and outputs First Thread
four times.
public void threadAssign() {
for (int threadCount = 0; threadCount < 6; threadCount++) {
Console.WriteLine("First Thread");
}
}
After that, we’ll create a new class called ThreadExampleMain
, including the main function.
class ThreadExampleMain {
public static void Main() {}
}
We need to perform the following operations to start and stop a thread inside the Main()
method. Create and initialize the variable threadDemo
with the datatype ThreadDemo
.
ThreadDemo threadDemo = new ThreadDemo();
We’ll use the Thread
class to initialize a new variable and assign it to the thread we created earlier.
Thread thread = new Thread(new ThreadStart(threadDemo.threadAssign));
The thread may be started by invoking the Start()
function.
thread.Start();
Finally, we may terminate the thread using the System.Threading library’s Abort()
method. We’ll also print "Thread is Abort"
to indicate that the thread has been terminated.
Console.WriteLine("Thread is abort");
thread.Abort();
Source Code:
using System;
using System.Threading;
class ThreadDemo {
void threadAssign() {
for (int threadCount = 0; threadCount < 6; threadCount++) {
Console.WriteLine("First Thread");
}
}
class ThreadExampleMain {
public static void Main() {
ThreadDemo threadDemo = new ThreadDemo();
Thread thread = new Thread(new ThreadStart(threadDemo.threadAssign));
thread.Start();
Console.WriteLine("Thread is abort");
thread.Abort();
}
}
}
Output:
First Thread
First Thread
First Thread
First Thread
First Thread
First Thread
Thread is abort
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn