在 C# 中停止线程
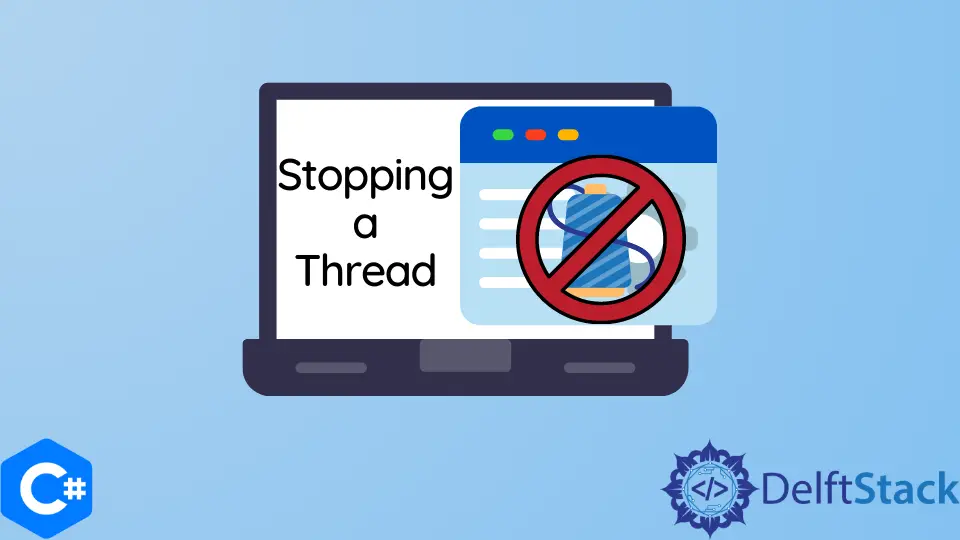
有时,在 C# 中使用线程时,你可能会遇到需要终止线程的情况。C# 确实为你提供了成功执行此操作的方法,本文旨在解释在 C# 中终止线程的过程。
为了终止线程的目标,我们将使用 Abort()
方法。ThreadAbortException
由 Abort()
方法抛出给调用它的线程,作为对该异常的响应,线程被终止。
在我们可以停止一个线程之前,我们必须先启动一个。要完成创建线程,请按照以下步骤操作。
在 C# 中使用 Abort()
方法停止线程
要利用 System.Threading
库的方法来启动和结束线程,请导入它。
using System.Threading;
我们需要创建一个 ThreadDemo
类。
class ThreadDemo {}
我们将在这个类中创建一个名为 threadAssign()
的线程方法,并带有一个循环,该循环迭代并输出 First Thread
四次。
public void threadAssign() {
for (int threadCount = 0; threadCount < 6; threadCount++) {
Console.WriteLine("First Thread");
}
}
之后,我们将创建一个名为 ThreadExampleMain
的新类,包括 main 函数。
class ThreadExampleMain {
public static void Main() {}
}
我们需要执行以下操作来启动和停止 Main()
方法中的线程。使用数据类型 ThreadDemo
创建并初始化变量 threadDemo
。
ThreadDemo threadDemo = new ThreadDemo();
我们将使用 Thread
类来初始化一个新变量并将其分配给我们之前创建的线程。
Thread thread = new Thread(new ThreadStart(threadDemo.threadAssign));
线程可以通过调用 Start()
函数来启动。
thread.Start();
最后,我们可以使用 System.Threading 库的 Abort()
方法终止线程。我们还将打印 "Thread is Abort"
以指示线程已终止。
Console.WriteLine("Thread is abort");
thread.Abort();
源代码:
using System;
using System.Threading;
class ThreadDemo {
void threadAssign() {
for (int threadCount = 0; threadCount < 6; threadCount++) {
Console.WriteLine("First Thread");
}
}
class ThreadExampleMain {
public static void Main() {
ThreadDemo threadDemo = new ThreadDemo();
Thread thread = new Thread(new ThreadStart(threadDemo.threadAssign));
thread.Start();
Console.WriteLine("Thread is abort");
thread.Abort();
}
}
}
输出:
First Thread
First Thread
First Thread
First Thread
First Thread
First Thread
Thread is abort
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn