C# 中的线程与任务
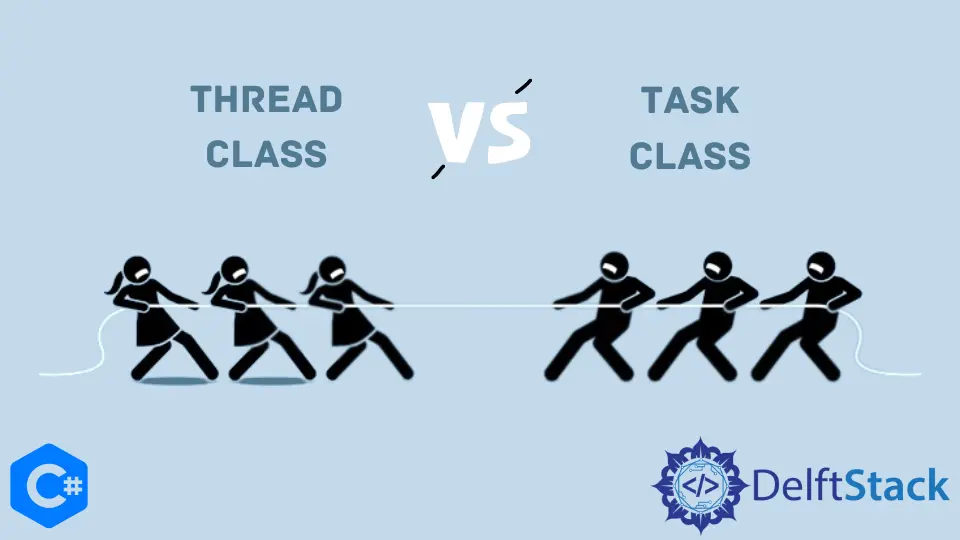
本教程将讨论 C# 中的 Thread
类和 Task
类之间的异同。
C# 中的线程
Thread
类在 C# 中创建实际的操作系统级别的线程。用 Thread
类创建的线程会占用堆栈内存等资源,上下文的 CPU 开销会从一个线程切换到另一个线程。Thread
类提供最高程度的控制,例如 Abort()
函数,Suspend()
函数,Resume()
函数等。我们还可以指定一些线程级属性,例如堆栈大小。以下代码示例向我们展示了如何使用 C# 中的 Thread
类创建线程。
using System;
using System.Threading;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Thread thread1 = new Thread(new ThreadStart(fun1));
Thread thread2 = new Thread(new ThreadStart(fun2));
thread1.Start();
thread2.Start();
Console.WriteLine("The End");
}
}
}
输出:
The End
Thread 2
Thread 2
Thread 1
Thread 1
在上面的代码中,我们使用 C# 中的 Thread
类创建了线程 thread1
和 thread2
。
C# 中的任务
一个 Task
类在 C# 中创建一个异步系统级任务。任务计划程序执行使用任务
类创建的任务。默认调度程序在线程池中运行任务。与用 Thread
类创建的线程不同,用 Task
类创建的任务不需要任何额外的内存或 CPU 资源。Task
类不能用于指定线程级属性,例如堆栈大小。由于 Task
类在线程池上运行,因此任何长时间运行的任务都可以填满线程池,而新任务最终可能会等待先前的任务完成执行。以下代码示例向我们展示了如何使用 C# 中的 Task
类创建任务。
using System;
using System.Threading;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Task task1 = Task.Factory.StartNew(() => fun1());
Task task2 = Task.Factory.StartNew(() => fun2());
Task.WaitAll(task1, task2);
Console.WriteLine("The End");
}
}
}
输出:
Thread 1
Thread 1
Thread 2
Thread 2
The End
在上面的代码中,我们使用 C# 中的 Task
类创建了任务 task1
和 task2
。
C# 中的线程与任务
Thread
类和 Task
类都用于 C# 中的并行编程。线程
是较低级别的实现,而任务
是较高级别的实现。它需要资源,而任务
却不需要。与 Task
类相比,它还提供了更多的控制。对于任何长时间运行的操作,应优先选择线程
,而对于任何其他异步操作,应优先选择任务
。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn