C# 中的執行緒與任務
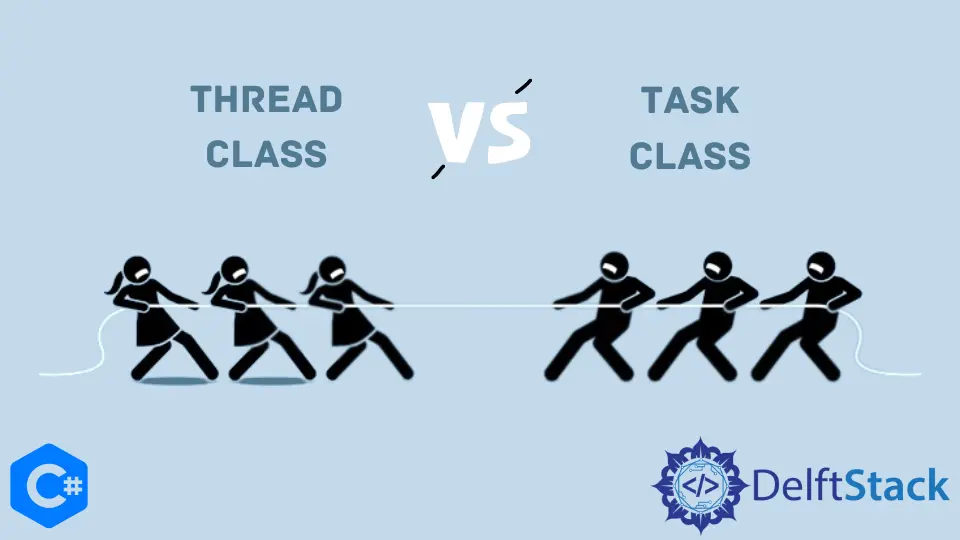
本教程將討論 C# 中的 Thread
類和 Task
類之間的異同。
C# 中的執行緒
Thread
類在 C# 中建立實際的作業系統級別的執行緒。用 Thread
類建立的執行緒會佔用堆疊記憶體等資源,上下文的 CPU 開銷會從一個執行緒切換到另一個執行緒。Thread
類提供最高程度的控制,例如 Abort()
函式,Suspend()
函式,Resume()
函式等。我們還可以指定一些執行緒級屬性,例如堆疊大小。以下程式碼示例向我們展示瞭如何使用 C# 中的 Thread
類建立執行緒。
using System;
using System.Threading;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Thread thread1 = new Thread(new ThreadStart(fun1));
Thread thread2 = new Thread(new ThreadStart(fun2));
thread1.Start();
thread2.Start();
Console.WriteLine("The End");
}
}
}
輸出:
The End
Thread 2
Thread 2
Thread 1
Thread 1
在上面的程式碼中,我們使用 C# 中的 Thread
類建立了執行緒 thread1
和 thread2
。
C# 中的任務
一個 Task
類在 C# 中建立一個非同步系統級任務。任務計劃程式執行使用任務
類建立的任務。預設排程程式線上程池中執行任務。與用 Thread
類建立的執行緒不同,用 Task
類建立的任務不需要任何額外的記憶體或 CPU 資源。Task
類不能用於指定執行緒級屬性,例如堆疊大小。由於 Task
類線上程池上執行,因此任何長時間執行的任務都可以填滿執行緒池,而新任務最終可能會等待先前的任務完成執行。以下程式碼示例向我們展示瞭如何使用 C# 中的 Task
類建立任務。
using System;
using System.Threading;
using System.Threading.Tasks;
namespace wait_for_thread {
class Program {
static void fun1() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 1");
}
}
static void fun2() {
for (int i = 0; i < 2; i++) {
Console.WriteLine("Thread 2");
}
}
static void Main(string[] args) {
Task task1 = Task.Factory.StartNew(() => fun1());
Task task2 = Task.Factory.StartNew(() => fun2());
Task.WaitAll(task1, task2);
Console.WriteLine("The End");
}
}
}
輸出:
Thread 1
Thread 1
Thread 2
Thread 2
The End
在上面的程式碼中,我們使用 C# 中的 Task
類建立了任務 task1
和 task2
。
C# 中的執行緒與任務
Thread
類和 Task
類都用於 C# 中的並行程式設計。執行緒
是較低階別的實現,而任務
是較高階別的實現。它需要資源,而任務
卻不需要。與 Task
類相比,它還提供了更多的控制。對於任何長時間執行的操作,應優先選擇執行緒
,而對於任何其他非同步操作,應優先選擇任務
。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn