C# 中的 lock 語句
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp Thread
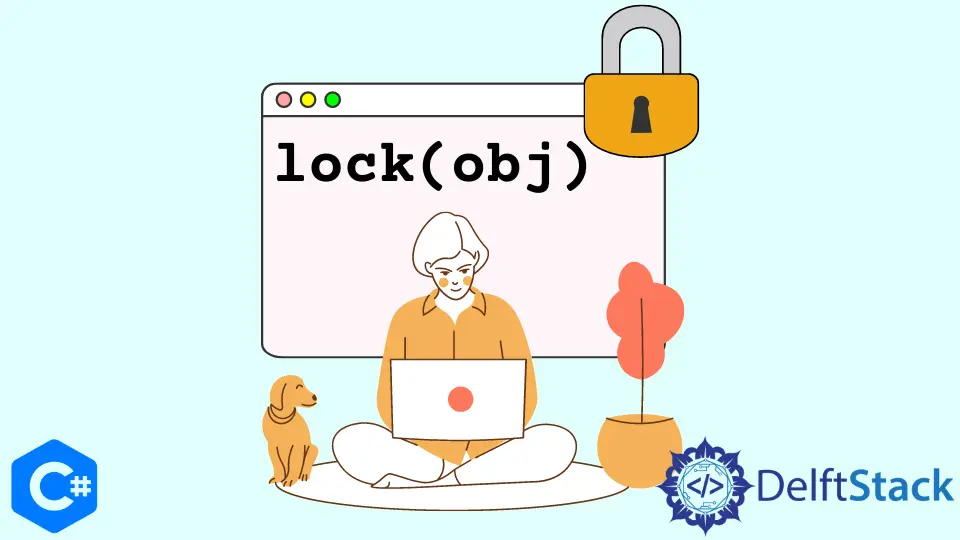
在本教程中,我們將討論 C# 中的 lock
語句。
C# 中的 lock
語句
lock(obj)
語句規定,在 C# 中,下面這段程式碼不能被多個執行緒同時訪問。lock(obj)
語句中的 obj
引數是 object
類的例項。lock(obj)
語句提供了一種在 C# 中管理執行緒的有效方法。如果一個執行緒訪問 lock(obj)
內的程式碼,而另一個執行緒想要訪問相同的程式碼,則第二個執行緒將不得不等待第一個執行緒執行它。lock(obj)
語句可確保順序執行指定的程式碼段。為了演示這種現象,我們將首先使用 lock(obj)
語句向你顯示程式碼的結果,而不用任何執行緒管理。
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
輸出:
The value to be printed is: 1
The value to be printed is: 1
The value to be printed is: 2
The value to be printed is: 3
The value to be printed is: 2
The value to be printed is: 3
我們可以看到,執行緒 firstthread
和 secondthread
都隨機訪問迴圈內的程式碼。我們將通過 lock(obj)
語句向你顯示執行緒管理的程式碼結果。
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
lock (lockname) {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
輸出:
The value to be printed is: 1
The value to be printed is: 2
The value to be printed is: 3
The value to be printed is: 1
The value to be printed is: 2
The value to be printed is: 3
這次,firstthread
和 secondthread
依次訪問迴圈內的程式碼。我們可以將用來更改變數值的程式碼放在 lock(obj)
語句中,以防止任何資料丟失。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn