Instruction lock en C#
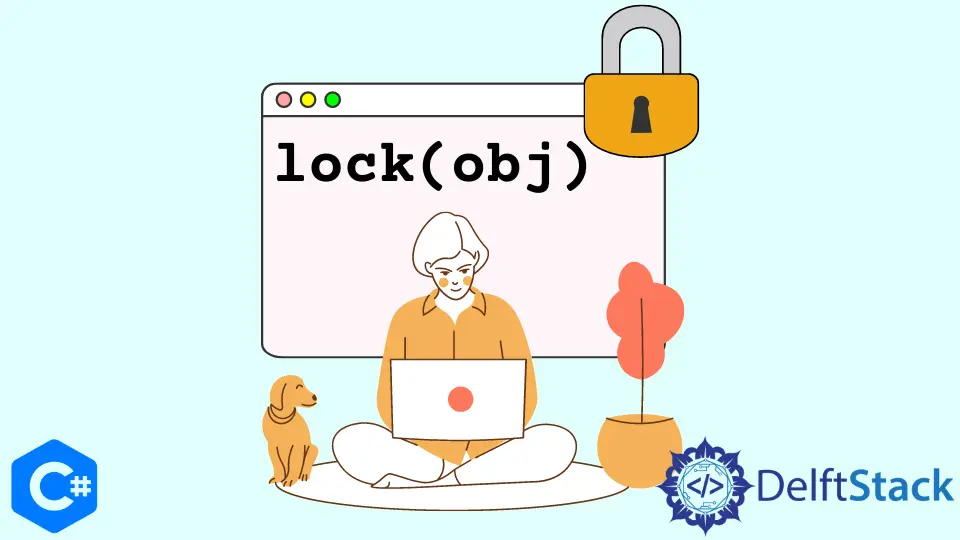
Dans ce tutoriel, nous aborderons l’instruction lock
en C#.
L’instruction lock
en C#
L’instruction lock(obj)
spécifie que la section de code suivante ne peut pas être accédée par plus d’un thread à la fois en C#. Le paramètre obj
à l’intérieur de l’instruction lock(obj)
est une instance de la classe object
. L’instruction lock(obj)
fournit un moyen efficace de gérer les threads en C#. Si le code à l’intérieur du lock(obj)
est accédé par un thread et qu’un autre thread veut accéder au même code, le second thread devra attendre que le premier thread l’exécute. L’instruction lock(obj)
assure l’exécution séquentielle de la section de code spécifiée. Pour démontrer ce phénomène, nous allons d’abord vous montrer le résultat du code sans aucune gestion de thread avec l’instruction lock(obj)
.
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
Production:
The value to be printed is : 1 The value to be printed is : 1 The value to be printed is : 2 The
value to be printed is : 3 The value to be printed is : 2 The value to be printed is : 3
Comme nous pouvons le voir, les threads firstthread
et secondthread
accèdent tous deux au code à l’intérieur de la boucle de manière aléatoire. Nous allons vous montrer le résultat du code avec la gestion des threads avec l’instruction lock(obj)
.
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
lock (lockname) {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
Production:
The value to be printed is : 1 The value to be printed is : 2 The value to be printed is : 3 The
value to be printed is : 1 The value to be printed is : 2 The value to be printed is : 3
Cette fois, le code à l’intérieur de la boucle est accédé séquentiellement à la fois par firstthread
et secondthread
. On peut placer du code dans lequel on change la valeur d’une variable dans une instruction lock(obj)
pour éviter toute perte de données.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn