Istruzione lock in C#
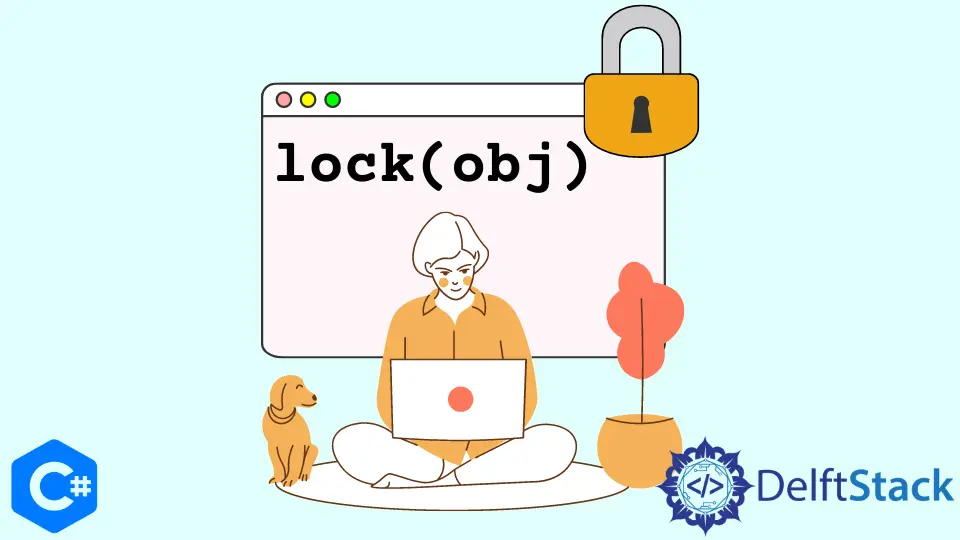
In questo tutorial, discuteremo l’istruzione lock
in C#.
L’istruzione lock
in C#
L’istruzione lock(obj)
specifica che la seguente sezione di codice non è accessibile da più di un thread contemporaneamente in C#. Il parametro obj
all’interno dell’istruzione lock(obj)
è un’istanza della classe object
. L’istruzione lock(obj)
fornisce un modo efficiente di gestire i thread in C#. Se un thread accede al codice all’interno del lock(obj)
e un altro thread vuole accedere allo stesso codice, il secondo thread dovrà attendere che il primo thread lo esegua. L’istruzione lock(obj)
garantisce l’esecuzione sequenziale della sezione di codice specificata. Per dimostrare questo fenomeno, ti mostreremo prima il risultato del codice senza alcuna gestione dei thread con l’istruzione lock(obj)
.
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
Produzione:
The value to be printed is : 1 The value to be printed is : 1 The value to be printed is : 2 The
value to be printed is : 3 The value to be printed is : 2 The value to be printed is : 3
Come possiamo vedere, i thread firstthread
e secondthread
accedono entrambi al codice all’interno del bucle in modo casuale. Ti mostreremo il risultato del codice con la gestione dei thread con l’istruzione lock(obj)
.
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
lock (lockname) {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
Produzione:
The value to be printed is : 1 The value to be printed is : 2 The value to be printed is : 3 The
value to be printed is : 1 The value to be printed is : 2 The value to be printed is : 3
Questa volta, al codice all’interno del bucle si accede in sequenza sia da firstthread
che da secondthread
. Possiamo inserire del codice in cui modificare il valore di una variabile all’interno di un’istruzione lock(obj)
per prevenire qualsiasi perdita di dati.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn